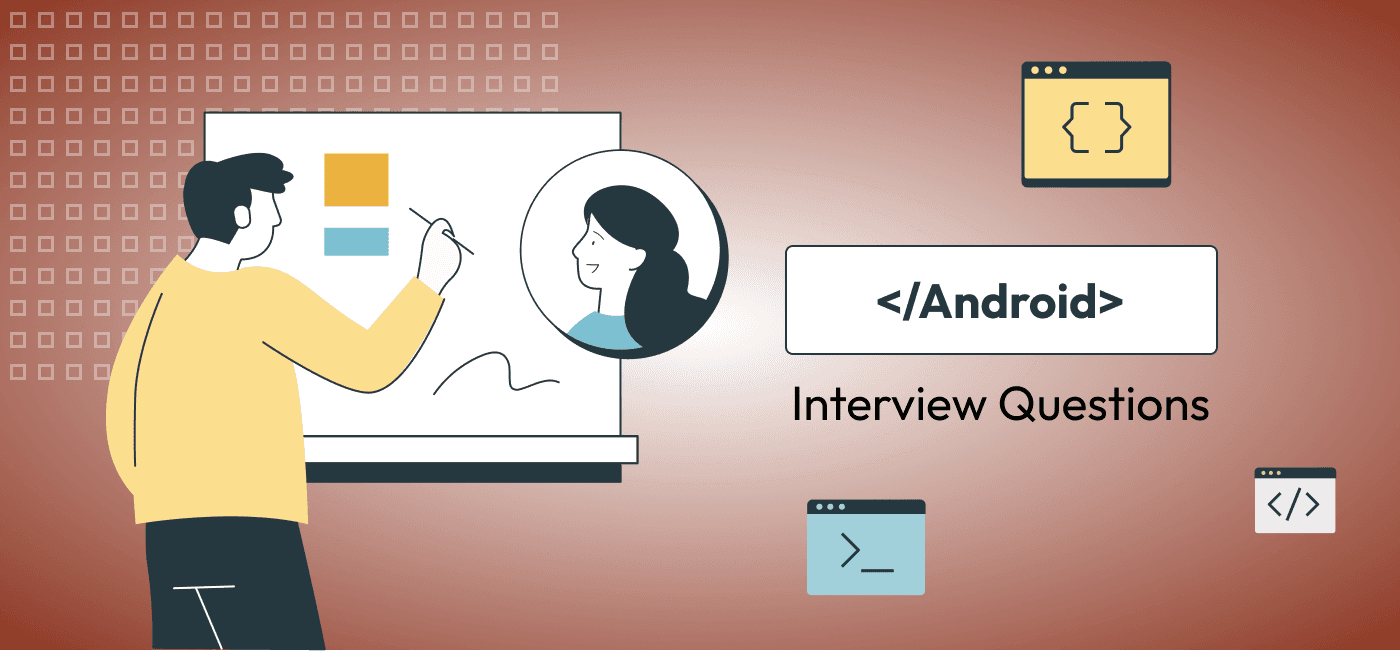
Hiring + recruiting | Blog Post
15 Android Interview Questions for Hiring Android Engineers
Todd Adams
Share this post
Hiring an Android engineer requires evaluating a candidate’s understanding of the Android ecosystem, mobile app architecture, and proficiency with key tools and libraries. The following 15 Android interview questions are designed to help interviewers assess a candidate’s knowledge and ability to build efficient, maintainable, and high-performance Android applications.
Android Interview Questions
1. What is the Android activity lifecycle, and why is it important?
Question Explanation:
The activity lifecycle is fundamental to how Android apps behave and manage resources. Understanding it is essential for creating responsive, memory-efficient applications. Knowing when and how an activity transitions between different states is key to ensuring smooth user experiences, handling configuration changes, and preventing memory leaks. This Android interview question will assist
Expected Answer:
The Android activity lifecycle consists of several stages that represent an activity’s state as it interacts with the system and the user. These states are:
onCreate()
: Called when the activity is first created. This is where initialization code like setting the layout and preparing data happens.onStart()
: Called when the activity becomes visible to the user.onResume()
: Called when the activity comes to the foreground and starts interacting with the user.onPause()
: Called when the system is about to move the activity to the background, such as when the user navigates to another app.onStop()
: Called when the activity is no longer visible.onDestroy()
: Called when the activity is finishing or being destroyed by the system.
Understanding when these methods are called helps in managing resources efficiently. For example, starting and stopping UI updates or releasing network resources when the activity pauses or stops helps reduce unnecessary resource consumption.
Evaluating Responses:
Look for answers that highlight understanding of each lifecycle stage and the importance of handling tasks like saving UI states, freeing up resources, and ensuring smooth transitions. Candidates should also mention lifecycle-aware components like ViewModel to demonstrate modern Android development practices.
2. Explain the differences between Activity
and Fragment
. When would you use a Fragment
over an Activity
?
Question Explanation:
Both Activity
and Fragment
are building blocks in Android apps, but they serve different purposes. This Android interview question assesses the candidate’s understanding of how to architect an app for flexibility and modularity using Fragments
, which are crucial for creating dynamic UI experiences.
Expected Answer:
An Activity
represents a single screen with a user interface in an Android app. It is the entry point for user interactions with the app. A Fragment
, on the other hand, is a modular section of an activity that can have its own layout and lifecycle, but it must be hosted within an Activity
.
The main differences are:
- Lifecycle: While both
Activity
andFragment
have lifecycles, the fragment’s lifecycle is linked to its hosting activity. For instance, when an activity is destroyed, its fragments are also destroyed. - Reusability: Fragments allow greater flexibility and modularity because they can be reused across multiple activities.
- UI Modularity: Fragments are especially useful for handling UI changes, such as switching between different sections of the app on larger screens (e.g., tablets).
You would use a Fragment
when you want a modular and reusable part of your UI that can be displayed in different activities or used in a single activity with dynamic layouts. For example, in a tablet app, you might have two fragments side by side, while in a phone app, you display them one at a time in a single activity.
Evaluating Responses:
Good answers will explain the lifecycle and functional differences, and provide examples of when a fragment is the appropriate choice (e.g., dynamic UIs, reusability). Candidates should highlight the use of the fragment manager for adding, removing, or replacing fragments dynamically.
3. How does Android handle background tasks? What are some ways to perform background operations?
Question Explanation:
Background tasks are crucial for performing operations that do not block the main UI thread, such as network requests or long computations. This Android interview question examines the candidate’s understanding of concurrency and performance optimization in Android.
Expected Answer:
Android provides several mechanisms for performing background tasks:
- AsyncTask (deprecated in Android 11): This was traditionally used for short background operations, but it is now discouraged due to limitations in handling lifecycle changes.
- IntentService: A service that handles asynchronous tasks on a background thread. It automatically stops once the task is complete, but it is also being phased out in favor of newer APIs.
- WorkManager: This is the recommended solution for deferrable, guaranteed background work. It supports periodic tasks, chaining tasks, and constraints such as network or charging status.
- JobScheduler: Useful for scheduling background jobs that persist across app restarts, introduced in Android 5.0 (Lollipop).
- Coroutines (Kotlin): Coroutines are now a popular, lightweight concurrency tool for background tasks that simplify asynchronous programming. They allow suspending functions, which can be paused and resumed without blocking the thread.
For long-running tasks, services (foreground or background) may be used, especially when the task needs to continue beyond the app’s lifecycle.
Evaluating Responses:
Look for an understanding of deprecated vs. modern approaches. The best responses should mention using WorkManager
for reliable background processing, Kotlin Coroutines for asynchronous operations, and the importance of avoiding operations on the main thread. Candidates should also be aware of lifecycle management in background tasks.
4. Can you explain the role of ViewModel
in Android architecture? How does it help with configuration changes?
Question Explanation:
ViewModel is a part of Android’s Jetpack library and is fundamental for managing UI-related data in a lifecycle-conscious way. This Android interview question checks the candidate’s familiarity with modern Android architecture (MVVM) and their ability to manage UI state.
Expected Answer:
ViewModel
is a class designed to store and manage UI-related data in a lifecycle-conscious way. It is typically used in the MVVM (Model-View-ViewModel) architecture pattern. The primary role of ViewModel
is to hold the UI state and data that survives configuration changes, such as screen rotations.
Key features of ViewModel
include:
- Lifecycle awareness:
ViewModel
is scoped to the lifecycle of an activity or fragment, meaning it stays in memory even if the screen is rotated or the activity is temporarily destroyed. - Data retention: Unlike traditional approaches where data might be lost during configuration changes (like screen rotation), data in a
ViewModel
is retained, avoiding the need to reload data from the network or database unnecessarily. - Separation of concerns: The
ViewModel
allows developers to decouple the UI (View) from business logic. TheView
observes changes in theViewModel
, which in turn interacts with the data layer.
By using ViewModel
, developers can avoid leaks and errors that occur when an activity or fragment is recreated during lifecycle changes.
Evaluating Responses:
Good responses will demonstrate an understanding of the ViewModel’s lifecycle awareness and its ability to preserve data. Candidates should mention how ViewModel works in conjunction with LiveData or other observable data holders to ensure reactive UI updates without risking memory leaks or wasted resources.
5. What is the difference between Implicit and Explicit intents in Android? Can you provide examples of both?
Question Explanation:
Intents are a core part of Android’s component-based architecture. Understanding the differences between implicit and explicit intents is important for determining how different components of an app or even external apps interact.
Expected Answer:
In Android, intents are used to request an action from another app component.
- Explicit Intents specify the exact component (such as an
Activity
,Service
, orBroadcastReceiver
) that should handle the intent. These are typically used within the same application when you know the target component in advance. For example, launching an activity from the same app:
Intent intent = new Intent(this, TargetActivity.class);
startActivity(intent);
- Implicit Intents, on the other hand, do not specify a particular component. Instead, they declare an action to be performed, allowing the system to find the appropriate app or component that can handle the request. Implicit intents are often used to request actions from external apps (such as sending an email or opening a web page). Example:
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse("https://www.example.com"));
startActivity(intent);
In this case, the system will choose an appropriate app (e.g., a web browser) to open the URL.
Evaluating Responses:
Good answers will explain the differences clearly and include appropriate examples. Look for candidates to describe scenarios where implicit intents are used to communicate between apps, while explicit intents are reserved for communication within the same app. Strong answers should emphasize how implicit intents facilitate modularity and interoperability between apps.
6. How do you optimize an Android app for performance and responsiveness?
Question Explanation:
Performance optimization is critical to providing a smooth user experience in Android apps. This Android interview question tests the candidate’s understanding of best practices for improving app performance, including memory management, smooth UI rendering, and battery consumption.
Expected Answer:
There are several key strategies for optimizing Android apps:
- Avoiding work on the main thread: Time-consuming operations, such as network requests or complex calculations, should be moved to background threads using tools like
WorkManager
,Thread
, or Kotlin Coroutines. Keeping the main thread free ensures the app remains responsive to user input. - Efficient memory management: Using object pooling, avoiding memory leaks (e.g., holding references to
Context
incorrectly), and freeing resources when no longer needed (e.g., stopping services) helps prevent out-of-memory errors. Tools likeLeakCanary
can help detect memory leaks. - Optimizing layout rendering: Reducing the number of nested views and unnecessary layouts can speed up UI rendering. Using
ConstraintLayout
efficiently helps reduce the complexity of UI hierarchies. Additionally, reusing UI components viaRecyclerView
(instead ofListView
) enhances performance when dealing with large data sets. - Lazy loading and caching: Loading only the data necessary at a given time (lazy loading) and caching data locally (in memory or disk) avoids unnecessary network requests or reloading, speeding up the user experience.
- Minimizing overdraw: Overdraw occurs when the system draws a pixel multiple times in a single frame. Optimizing your UI to reduce this can greatly improve rendering performance.
Evaluating Responses:
Look for answers that include specific performance strategies and tools such as memory profilers, layout inspectors, or third-party libraries like Glide for image loading. Candidates should emphasize avoiding blocking the UI thread and managing memory efficiently. Excellent responses will also touch on app size optimization and battery conservation strategies.
7. What are the benefits of using Jetpack components in Android development? Which ones have you worked with?
Question Explanation:
Jetpack is a set of libraries that help developers follow best practices, reduce boilerplate code, and write more maintainable apps. This Android interview question gauges a candidate’s familiarity with modern Android development practices.
Expected Answer:
Jetpack components are designed to simplify Android development by providing solutions to common challenges and ensuring apps are more robust, testable, and maintainable. Some key benefits include:
- Lifecycle awareness: Jetpack components such as
LiveData
andViewModel
are lifecycle-aware, meaning they automatically adjust to lifecycle changes (e.g., screen rotations or background/foreground transitions), reducing the risk of memory leaks and crashes. - Modularity and flexibility: Jetpack offers a modular approach, allowing developers to pick and choose only the components they need. For example, using
Navigation
for managing in-app navigation orRoom
for managing local databases. - Ease of integrating modern architecture: Jetpack makes it easier to implement architecture patterns like MVVM (Model-View-ViewModel), providing components like
ViewModel
for separating business logic from the UI andLiveData
for observing data changes in a lifecycle-safe manner. - Increased testability: Components such as
WorkManager
andRoom
are designed to be test-friendly, helping developers write reliable unit and integration tests.
Examples of Jetpack components a candidate might mention:
ViewModel
andLiveData
for managing UI-related data in a lifecycle-aware manner.Navigation
for handling app navigation and deep linking.Room
for local database management, replacing raw SQLite handling.WorkManager
for managing deferrable background tasks.
Evaluating Responses:
Look for candidates who have hands-on experience with multiple Jetpack components, especially core components like ViewModel
, LiveData
, and Navigation
. Ideal responses will emphasize how these libraries help follow best practices and improve app architecture, reducing boilerplate code and making the app more testable and maintainable.
8. How does Android handle app permissions? What changes were introduced in Android 6.0 (Marshmallow)?
Question Explanation:
Understanding Android’s permission system is crucial for ensuring user privacy and security, as well as maintaining a good user experience. Marshmallow (Android 6.0) introduced significant changes to how permissions are handled, requiring developers to adapt their apps accordingly.
Expected Answer:
Prior to Android 6.0 (Marshmallow), users were required to grant all permissions at the time of installation, a model known as install-time permissions. If a user declined, they could not install the app. This often led to poor user experiences and security issues, as users were often unaware of what permissions the app actually needed during usage.
With Android 6.0, runtime permissions were introduced. Now, apps request permissions at runtime, only when they are needed. For example, an app would only request access to the camera when the user tries to take a photo. Users can also revoke permissions at any time via the settings.
Key changes introduced in Android 6.0 include:
- Dangerous permissions (such as accessing the camera, location, or contacts) must be requested at runtime.
- Normal permissions (like access to the internet) are automatically granted at install time, as they don’t affect user privacy.
- Permissions can be denied or revoked by users, and developers must handle these cases gracefully.
Here’s an example of requesting a permission at runtime:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.CAMERA)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.CAMERA}, REQUEST_CAMERA_PERMISSION);
}
Evaluating Responses:
Good responses should emphasize the shift from install-time to runtime permissions and why this change was important for privacy and security. Candidates should discuss how to handle permission denial and revoke scenarios, and best practices for explaining to users why permissions are necessary.
9. Can you explain what Android’s RecyclerView
is and how it differs from ListView
? How would you implement a RecyclerView
?
Question Explanation:
This Android interview question tests the candidate’s understanding of efficient UI components in Android and highlights their experience with RecyclerView
, a more flexible and powerful alternative to ListView
for displaying large sets of data.
Expected Answer:
RecyclerView
is an advanced version of ListView
used for displaying lists or grids of data in Android. While ListView
is older and simpler, RecyclerView
provides more features and greater control over how data is displayed. Key differences include:
- ViewHolder Pattern:
RecyclerView
enforces the ViewHolder pattern, which improves performance by reusing view objects and avoiding unnecessaryfindViewById()
calls. In contrast,ListView
requires manual implementation of the ViewHolder pattern. - Layout Managers:
RecyclerView
allows for flexible layouts viaLayoutManager
classes, such asLinearLayoutManager
(for vertical or horizontal lists),GridLayoutManager
(for grids), andStaggeredGridLayoutManager
.ListView
only supports a vertical list. - Item Animations and Decorations:
RecyclerView
provides built-in support for adding item animations and decorations (like dividers) between items, features that are limited or non-existent inListView
.
To implement a RecyclerView
:
- Add the
RecyclerView
widget to your layout file. - Create an adapter by extending
RecyclerView.Adapter
and defining aViewHolder
class to represent individual list items. - Set the
LayoutManager
(e.g.,LinearLayoutManager
) and connect the adapter to theRecyclerView
.
Example:
RecyclerView recyclerView = findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
MyAdapter adapter = new MyAdapter(dataList);
recyclerView.setAdapter(adapter);
Evaluating Responses:
Strong candidates will discuss the performance benefits of RecyclerView
, especially when dealing with large datasets. Look for answers that include practical implementation steps, mention the ViewHolder pattern, and explain the flexibility provided by different LayoutManager
types. Candidates should also understand the limitations of ListView
compared to RecyclerView
.
10. What is ProGuard in Android? How do you use it to optimize and secure your APK?
Question Explanation:
ProGuard is an important tool for Android app developers to optimize and obfuscate their code. This Android interview question evaluates the candidate’s understanding of how to shrink APK size and protect code from reverse engineering.
Expected Answer:
ProGuard is a tool that helps reduce the size of APK files and obfuscate Java bytecode to make it harder to reverse engineer Android apps. It achieves this by performing the following tasks:
- Code Shrinking: ProGuard removes unused classes, methods, fields, and attributes from the app, which reduces the APK size.
- Obfuscation: It renames classes and variables with short, meaningless names, making it more difficult for someone to reverse-engineer the app.
- Optimization: ProGuard optimizes bytecode and removes redundant operations, further improving app performance.
- Pre-verification: It pre-verifies code, which can improve runtime performance.
ProGuard is configured in the proguard-rules.pro
file. By default, Android Studio includes this file, and ProGuard is enabled for release builds. Developers can add custom rules to ensure certain classes or methods are not removed or obfuscated, especially for reflection-based libraries.
For example, to prevent ProGuard from obfuscating a specific class:
-keep class com.example.MyClass { *; }
To enable ProGuard for a release build, developers ensure that the following is included in the build.gradle
file:
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
Evaluating Responses:
Look for candidates to explain how ProGuard helps with both size reduction and code obfuscation. They should also provide insight into managing ProGuard rules effectively, ensuring important classes or methods are kept intact while optimizing others. Bonus points for candidates who mention R8, the successor to ProGuard that is now integrated into Android builds.
11. Explain how dependency injection works in Android. What is Dagger, and how is it used in Android applications?
Question Explanation:
Dependency injection (DI) is a software design pattern that is crucial for building testable, maintainable, and modular applications. Dagger is one of the most popular DI frameworks in Android. This Android interview question checks the candidate’s understanding of DI and experience using Dagger in real-world projects.
Expected Answer:
Dependency Injection is a design pattern where an object’s dependencies (e.g., services, classes) are provided to it, rather than the object creating the dependencies itself. This promotes loose coupling, making the code more modular, testable, and easier to maintain.
In Android, Dagger is a popular DI framework used to manage dependencies. It generates boilerplate code for injecting dependencies, reducing manual coding and improving performance. Dagger uses annotations like @Inject
, @Module
, and @Component
to define how dependencies should be provided and injected.
Basic steps to implement Dagger:
- Define a Module: A module provides the dependencies using methods annotated with
@Provides
or@Binds
.
@Module
public class NetworkModule {
@Provides
public NetworkService provideNetworkService() {
return new NetworkService();
}
}
- Create a Component: A component defines where the dependencies should be injected, typically in an activity or fragment.
@Component(modules = {NetworkModule.class})
public interface ApplicationComponent {
void inject(MainActivity activity);
}
- Inject the Dependencies: Use the component to inject the dependencies into the target class.
public class MainActivity extends AppCompatActivity {
@Inject
NetworkService networkService;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
DaggerApplicationComponent.create().inject(this);
// Now networkService is available
}
}
Dagger allows dependencies to be injected at runtime and enables developers to test components in isolation by injecting mock dependencies during testing.
Evaluating Responses:
Good candidates will clearly explain the concept of dependency injection, how it helps with code maintainability and testability, and how Dagger works in Android. Strong responses will include practical examples and highlight the advantages of using Dagger over manual dependency injection, such as automatic code generation and better performance.
12. What is a Content Provider in Android, and when would you use one?
Question Explanation:
Content Providers are used for sharing data between apps, which is a key aspect of Android’s inter-process communication system. This Android interview question tests the candidate’s understanding of how Android apps share data in a secure and structured way.
Expected Answer:
A Content Provider is a component that enables an app to share its data with other apps or to access data from other apps. Data can be stored in a variety of formats, such as databases, files, or over the network. Content providers use a URI to identify the data they expose and use permissions to enforce data security.
A typical use case for a Content Provider is sharing structured data, such as contacts, between different apps. The Android system itself provides several built-in content providers, such as the ContactsProvider, MediaStore, and CalendarProvider, which allow other apps to access contacts, media files, or calendar events.
To implement a custom Content Provider:
- Extend the
ContentProvider
class. - Implement methods like
query()
,insert()
,update()
, anddelete()
to interact with the underlying data store. - Expose the data using a URI pattern (e.g.,
content://com.example.provider/table
).
Example of querying a Content Provider:
Cursor cursor = getContentResolver().query(
ContactsContract.Contacts.CONTENT_URI,
null, null, null, null);
Content Providers are typically used in cases where you need to:
- Share data across different apps securely.
- Manage access to complex data (e.g., databases).
- Provide a standardized interface for accessing shared data.
Evaluating Responses:
Look for candidates to explain the role of Content Providers clearly and provide examples of both built-in and custom implementations. They should also mention when it’s appropriate to use a Content Provider (e.g., when sharing data between apps or accessing shared data). Bonus points for explaining security mechanisms, such as read/write permissions.
13. How do you manage state in an Android application? Can you explain the role of LiveData
and StateFlow
?
Question Explanation:
Managing state is crucial in Android development, particularly when dealing with configuration changes, background tasks, and complex user interactions. This Android interview question tests a candidate’s ability to manage UI-related state effectively and knowledge of modern Android components for reactive state management.
Expected Answer:
In Android, state refers to data that needs to be preserved and managed across user interactions and configuration changes. Several tools and patterns help manage state effectively:
- LiveData: A lifecycle-aware observable component provided by Jetpack.
LiveData
is designed to hold UI-related data that can be observed by activities or fragments. It ensures that data updates happen only when the lifecycle is in an active state, preventing memory leaks and crashes due to improper lifecycle management.
Example usage of LiveData
:
class MyViewModel extends ViewModel {
private MutableLiveData<String> data = new MutableLiveData<>();
public LiveData<String> getData() {
return data;
}
public void setData(String value) {
data.setValue(value);
}
}
In an activity or fragment:
viewModel.getData().observe(this, new Observer<String>() {
@Override
public void onChanged(String s) {
// Update the UI
}
});
- StateFlow: Part of Kotlin’s
Flow
API,StateFlow
is a more modern alternative toLiveData
for managing state in Kotlin-based apps. UnlikeLiveData
,StateFlow
is part of Kotlin Coroutines and represents a state holder that emits updates in a flow-like manner. It is particularly useful for managing state in more reactive or asynchronous contexts.
Example of StateFlow
:
class MyViewModel : ViewModel() {
private val _stateFlow = MutableStateFlow<String>("Initial State")
val stateFlow: StateFlow<String> = _stateFlow
fun updateState(value: String) {
_stateFlow.value = value
}
}
In an activity or fragment:
lifecycleScope.launchWhenStarted {
viewModel.stateFlow.collect { state ->
// Update the UI
}
}
Both LiveData
and StateFlow
are designed to handle state changes efficiently and ensure that the UI is updated in response to these changes.
Evaluating Responses:
Good answers will include a comparison between LiveData
and StateFlow
and explain the lifecycle-awareness and reactive capabilities of both tools. Candidates should also highlight their use cases and practical examples of managing state across configuration changes, such as screen rotations.
14. What are the different types of storage mechanisms available in Android, and when would you use each?
Question Explanation:
Android offers several storage options for saving data persistently, each suited for different use cases. This Android interview question assesses a candidate’s understanding of the available storage mechanisms and their ability to select the appropriate one based on the app’s needs.
Expected Answer:
Android provides several storage mechanisms, each suited for different types of data and access patterns:
- SharedPreferences: Used to store small amounts of primitive data (key-value pairs). Ideal for saving simple user preferences or settings. The data is stored in an XML file inside the app’s private storage.
- Example: Saving a boolean value to indicate whether the user has completed onboarding.
SharedPreferences sharedPref = getSharedPreferences("MyPrefs", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPref.edit();
editor.putBoolean("onboardingComplete", true);
editor.apply();
- Internal Storage: Files saved to internal storage are private to the app. This method is used for sensitive data or data that should not be accessed by other apps. Files can be written using the
FileOutputStream
.
- Example: Storing user-specific data like session tokens.
FileOutputStream fos = openFileOutput("myfile.txt", Context.MODE_PRIVATE);
fos.write(data.getBytes());
fos.close();
- External Storage: Used to store large files (like images or videos) that may need to be accessed by other apps or by the user (e.g., media files). It requires permission from the user to read/write files on external storage, and the data may be visible to other apps.
- Example: Saving images to a public directory.
File file = new File(getExternalFilesDir(Environment.DIRECTORY_PICTURES), "image.jpg");
- SQLite Database: Suitable for structured data storage. SQLite is a lightweight database engine built into Android. It’s ideal for managing complex datasets, such as user profiles, app data, or logs.
- Example: Storing a list of user tasks in a todo app.
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put("task_name", "Complete project");
db.insert("tasks", null, values);
- Room: A Jetpack library built on top of SQLite that simplifies database operations and adds compile-time validation of SQL queries. It provides an abstraction layer over SQLite and is more developer-friendly with support for annotations and Kotlin Coroutines.
- Example: Managing local data persistence in an offline-first app.
@Entity
public class Task {
@PrimaryKey
public int id;
public String taskName;
}
@Dao
public interface TaskDao {
@Insert
void insertTask(Task task);
@Query("SELECT * FROM Task")
List<Task> getAllTasks();
}
Evaluating Responses:
Candidates should provide clear use cases for each storage mechanism and explain when to use each based on security, scalability, and performance considerations. Look for knowledge of newer libraries like Room and the ability to differentiate between internal and external storage requirements.
15. Can you explain the architecture of a recent Android project you worked on? How did you approach things like navigation, data management, and UI updates?
Question Explanation:
This Android interview question invites candidates to demonstrate their experience and thought process when architecting an Android app. It tests their ability to break down complex systems and their familiarity with architectural patterns like MVVM or Clean Architecture.
Expected Answer:
A candidate should describe their project architecture, typically involving a layered approach. For instance, using the MVVM (Model-View-ViewModel) pattern, they might break down their app as follows:
- View: Responsible for rendering the UI and observing the ViewModel for data changes. In Android, the
Activity
orFragment
acts as the View.
- Example: Using
LiveData
orStateFlow
in the View to update the UI reactively.
viewModel.userData.observe(this, Observer { data ->
// Update UI with user data
})
- ViewModel: The ViewModel holds the business logic and the state of the View. It communicates with the repository layer for data operations and exposes observable data to the View.
- Example: ViewModel fetching data from a repository:
class UserViewModel(private val repository: UserRepository) : ViewModel() {
val userData: LiveData<User> = repository.getUserData()
}
- Repository: Acts as a mediator between the ViewModel and the data sources (e.g., a local database or a remote API). It abstracts the data sources and provides a clean API for the ViewModel to interact with.
- Example: A repository fetching user data from a Room database and a remote API:
class UserRepository(private val userDao: UserDao, private val apiService: ApiService) {
fun getUserData(): LiveData<User> {
return userDao.getUser()
}
suspend fun fetchRemoteData() {
val response = apiService.getUser()
userDao.insertUser(response)
}
}
- Navigation: Using the Jetpack Navigation component for handling in-app navigation. This provides a declarative way to handle navigation flows, including deep linking and back stack management.
- Example: Defining navigation in
nav_graph.xml
and navigating between fragments:
<fragment
android:id="@+id/firstFragment"
android:name="com.example.FirstFragment"
tools:layout="@layout/fragment_first" />
In the ViewModel or Activity:
findNavController().navigate(R.id.action_firstFragment_to_secondFragment)
- Data Management: Using Room for local database management and Retrofit or Coroutines for handling network calls. This separation of concerns allows for more testable and scalable code.
Evaluating Responses:
The ideal candidate will demonstrate a clear understanding of modern architectural patterns like MVVM, Clean Architecture, or MVI. They should discuss how they handle complex requirements like navigation, data persistence, and network operations. Answers that mention modern libraries (Room, Retrofit, Navigation Component) and describe a thoughtful approach to separation of concerns show deep experience.
Android Interview Questions Conclusion
These Android interview questions cover a wide range of Android development topics, from basic app architecture to advanced performance tuning and state management. Asking these questions will help assess a candidate’s technical proficiency, experience with Android’s unique challenges, and ability to write efficient, maintainable code.