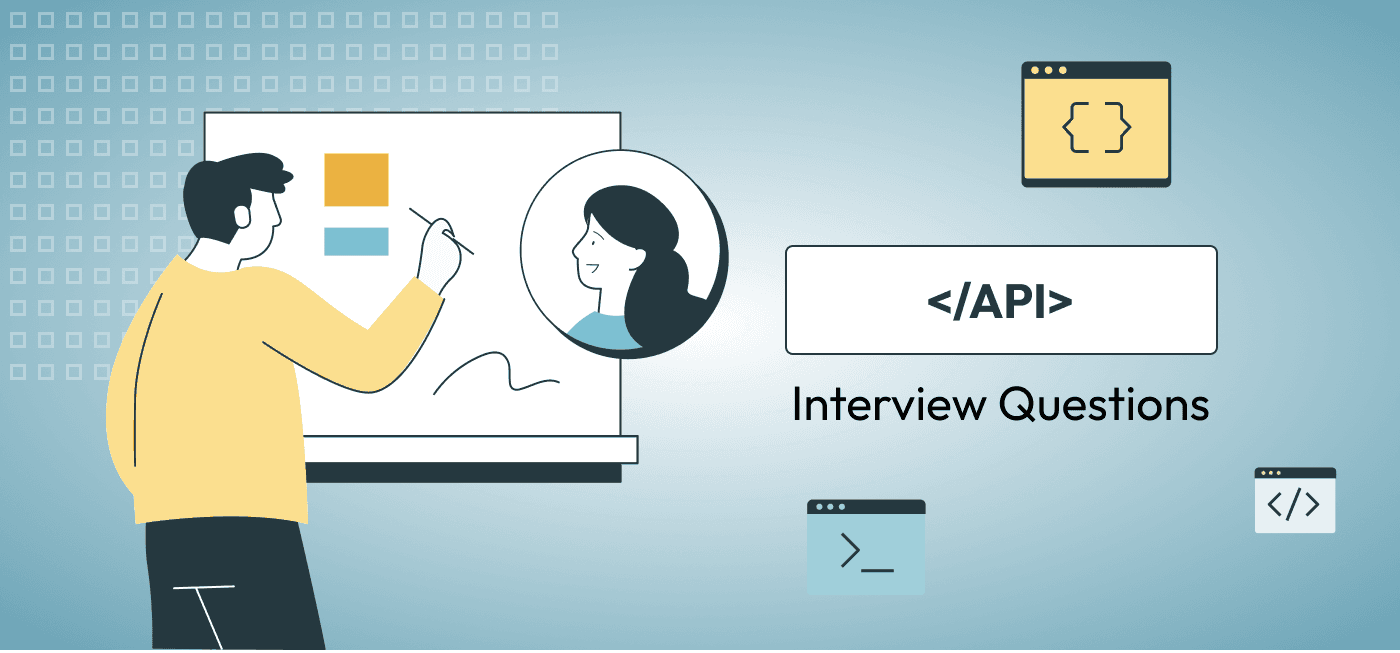
Hiring + recruiting | Blog Post
15 API Developer Interview Questions for Hiring API Engineers
Todd Adams
Share this post
When hiring an API developer, it’s crucial to assess their understanding of APIs, including their design, implementation, testing, and maintenance. Strong API developers possess skills in REST, GraphQL, and other API frameworks, along with proficiency in integrating APIs into various systems. The following API developer interview questions are designed to evaluate a candidate’s expertise in API development, problem-solving abilities, and capacity to handle real-world challenges.
API Developer Interview Questions
1. What are the differences between RESTful APIs and GraphQL?
Question Explanation:
This API Developer interview question assesses the candidate’s understanding of two widely used API paradigms: REST and GraphQL. A strong answer will demonstrate knowledge of their design principles, use cases, and trade-offs.
Expected Answer:
RESTful APIs follow a resource-based architecture where each URL represents a resource, and standard HTTP methods (GET, POST, PUT, DELETE) are used to interact with these resources. REST APIs typically return fixed structures of data defined by the server.
GraphQL, on the other hand, is a query language for APIs that allows clients to request specific data structures. Unlike REST, GraphQL consolidates multiple endpoints into a single entry point, enabling more flexible and efficient data retrieval.
Key differences include:
- Data Fetching: REST can over-fetch or under-fetch data, whereas GraphQL allows fetching only the required fields.
- Versioning: REST APIs often use versioning (e.g.,
/v1/resource
), while GraphQL evolves through schema updates. - Error Handling: REST uses HTTP status codes for errors, while GraphQL centralizes error handling in the response body.
Evaluating Responses:
Look for clarity in explanation, understanding of use cases, and mention of pros/cons like REST being simpler and easier to cache, while GraphQL offers flexibility and efficiency at the cost of complexity.
2. How would you design an API for a real-time chat application?
Question Explanation:
This API Developer interview question tests a candidate’s ability to think through real-world API design scenarios, considering the requirements for functionality, scalability, and performance in real-time systems.
Expected Answer:
To design an API for a real-time chat application:
- Endpoints: Create endpoints for managing users (
/users
), retrieving chat rooms (/rooms
), sending messages (/messages
), and fetching message history (/messages/history
). - WebSocket Integration: Use WebSockets or server-sent events (SSE) for real-time communication instead of standard HTTP requests.
- Authentication: Implement token-based authentication (e.g., JWT) for secure access to chat features.
- Scalability: Use database sharding or a message queue system (e.g., RabbitMQ) to handle high traffic.
- Rate Limiting: Apply rate limits to prevent abuse.
- Data Format: Use JSON or Protocol Buffers for lightweight data transfer.
Evaluating Responses:
Look for detailed reasoning about real-time technologies like WebSockets and mention of scalability, security, and performance. Bonus points for considering edge cases such as handling offline users.
3. What is the purpose of API versioning, and how would you implement it?
Question Explanation:
API versioning ensures backward compatibility and smooth transitions for clients as APIs evolve. This API Developer interview question evaluates the candidate’s grasp of maintaining long-term API reliability.
Expected Answer:
API versioning allows changes in functionality or structure without disrupting existing clients. Versions can be implemented through:
- URI Versioning: Including the version in the URL (e.g.,
/v1/resource
). - Header Versioning: Using custom headers (e.g.,
X-API-Version: 1
). - Query Parameter: Specifying the version in the query string (e.g.,
?version=1
).
Versioning is crucial for accommodating breaking changes, like modifying response formats, introducing new fields, or removing deprecated functionality.
Evaluating Responses:
Good answers mention pros and cons of each versioning strategy and emphasize backward compatibility. Bonus points for discussing strategies to deprecate older versions.
4. Explain the role of authentication and authorization in API security. How would you implement them?
Question Explanation:
Authentication and authorization are critical for API security. This API Developer interview question gauges a candidate’s understanding of securing API endpoints against unauthorized access.
Expected Answer:
- Authentication verifies a user’s identity, ensuring the user is who they claim to be. Common methods include API keys, OAuth2, and JWT (JSON Web Tokens).
- Authorization determines what actions or resources a user is permitted to access, based on their roles or permissions.
To implement:
- Use a robust authentication mechanism like OAuth2 for third-party applications or JWT for token-based authentication.
- Secure sensitive endpoints with role-based access control (RBAC).
- Use HTTPS to encrypt all communications and prevent token interception.
Evaluating Responses:
Strong answers should demonstrate knowledge of security best practices, including token expiration, secure storage, and mitigation of vulnerabilities like replay attacks. Look for candidates who emphasize the separation of authentication and authorization logic.
5. What is rate limiting, and why is it essential for APIs? How would you implement it?
Question Explanation:
Rate limiting is a key aspect of API security and performance. This API Developer interview question assesses a candidate’s understanding of protecting APIs from abuse (e.g., DDoS attacks) and ensuring fair usage.
Expected Answer:
Rate limiting restricts the number of requests a client can make to an API within a specific timeframe. It is crucial for:
- Preventing Abuse: Stops users or bots from overwhelming the server with excessive requests.
- Ensuring Fairness: Ensures equal access for all users by avoiding resource monopolization.
- Protecting Infrastructure: Safeguards the server from crashes due to traffic spikes.
Implementation can be done via:
- Fixed Window: Sets a maximum number of requests per time window (e.g., 100 requests per minute).
- Sliding Window: Smoothens rate limits by allowing overlapping time intervals.
- Token Bucket Algorithm: Allocates tokens that are consumed with each request, replenished at a steady rate.
- Tools/Frameworks: Use API gateways like NGINX or third-party tools such as Kong, or implement using libraries like
express-rate-limit
for Node.js.
Evaluating Responses:
A good response will demonstrate knowledge of rate-limiting algorithms and mention practical implementations. Look for candidates who address potential issues like user blocking or performance trade-offs.
6. Can you describe the process of creating a RESTful API from scratch? What tools and frameworks would you use?
Question Explanation:
This API Developer interview question evaluates a candidate’s ability to design and build a RESTful API from the ground up, including considerations for tools, frameworks, and architecture.
Expected Answer:
- Requirement Analysis: Understand the client’s requirements and identify resources (e.g., users, products).
- Define Endpoints: Map CRUD operations to resources using REST conventions (e.g.,
GET /users
,POST /users
). - Set Up Environment: Use a framework like Express.js (Node.js), Django (Python), or Spring Boot (Java) to streamline development.
- Database Integration: Choose a database (e.g., MySQL, MongoDB) and design a schema for the resources.
- Implement Logic: Add controllers and services to handle API requests and interact with the database.
- Secure the API: Implement authentication (e.g., OAuth2, JWT) and HTTPS.
- Testing: Use tools like Postman or automated testing frameworks (e.g., Jest, Pytest) to validate endpoints.
- Documentation: Document endpoints using Swagger or similar tools for client reference.
Evaluating Responses:
Look for a structured answer that highlights planning, development, testing, and documentation. Bonus points for mentioning best practices like RESTful conventions and modular design.
7. What is the significance of HTTP methods (GET, POST, PUT, DELETE, etc.) in API design, and how do you decide which method to use?
Question Explanation:
This API Developer interview question tests a candidate’s understanding of the semantics of HTTP methods and their role in creating intuitive, standards-compliant APIs.
Expected Answer:
HTTP methods provide a standardized way to interact with resources in a RESTful API:
- GET: Retrieve data without modifying the server state (e.g.,
GET /users
for all users). - POST: Create new resources (e.g.,
POST /users
to add a user). - PUT: Update or replace an existing resource (e.g.,
PUT /users/{id}
). - PATCH: Partially update a resource (e.g.,
PATCH /users/{id}
to change a single attribute). - DELETE: Remove a resource (e.g.,
DELETE /users/{id}
).
Selection depends on the desired operation:
- GET for read operations.
- POST for creation since it typically does not rely on an existing resource.
- PUT/PATCH for updates, depending on whether the change is full or partial.
- DELETE for removals.
Evaluating Responses:
Strong answers demonstrate an understanding of idempotency (e.g., PUT and DELETE are idempotent while POST is not) and adherence to RESTful principles. Candidates should emphasize clarity and predictability in API design.
8. How do you handle errors in APIs? What are best practices for returning error messages?
Question Explanation:
Error handling is vital for ensuring a good developer experience and debugging ease. This API Developer interview question assesses knowledge of robust error-handling strategies and adherence to standards.
Expected Answer:
Handling errors involves:
- HTTP Status Codes: Use standard codes to indicate errors (e.g.,
400
for bad requests,401
for unauthorized access,404
for not found, and500
for server errors). - Detailed Messages: Provide clear error messages in the response body that specify what went wrong and possible remediation steps. Example:
{
"error": "Invalid request",
"details": "The 'email' field is required."
}
- Consistent Structure: Maintain a uniform error response format across all endpoints.
- Log Errors: Log errors server-side for debugging and analytics.
- Don’t Expose Sensitive Information: Avoid exposing stack traces or sensitive internal details to the client.
Best Practices:
- Include a unique error code for easier troubleshooting.
- Document possible errors for each endpoint in the API documentation.
- Provide fallback responses for unexpected errors (e.g.,
500
).
Evaluating Responses:
Good candidates will demonstrate knowledge of HTTP status codes, error response formats, and logging. Look for awareness of user experience and security considerations.
9. What are some strategies for optimizing API performance? Provide specific examples.
Question Explanation:
Optimizing API performance is critical for scalability and user experience. This API Developer interview question evaluates a candidate’s understanding of techniques to reduce latency, improve response times, and handle high traffic.
Expected Answer:
API performance optimization involves several strategies:
- Caching: Use tools like Redis or built-in HTTP cache headers (
ETag
,Cache-Control
) to store frequently accessed responses. Example: Cache results of a product catalog API to avoid repetitive database queries. - Database Optimization: Optimize queries by indexing, denormalizing tables, or using an appropriate database type (e.g., relational vs. NoSQL). Use connection pooling to reduce overhead.
- Pagination and Chunking: Limit the data returned by endpoints with pagination (
?page=1&limit=20
) or chunking for large datasets. - Load Balancing: Distribute API traffic across multiple servers to prevent bottlenecks.
- Compression: Compress responses using Gzip or Brotli to reduce payload sizes.
- Asynchronous Processing: Implement asynchronous operations and queues (e.g., RabbitMQ, Kafka) for non-critical tasks to avoid blocking API responses.
Evaluating Responses:
Strong responses will mention specific tools and techniques, along with an understanding of trade-offs. Bonus points for highlighting monitoring tools (e.g., New Relic, Prometheus) to track performance.
10. How do you test APIs? What tools and methods would you recommend?
Question Explanation:
Testing is essential to ensure API functionality, reliability, and security. This API Developer interview question assesses the candidate’s knowledge of API testing practices and tools.
Expected Answer:
API testing involves:
- Functional Testing: Verifies endpoints perform as expected. Tools: Postman, SoapUI.
- Integration Testing: Ensures seamless interaction between API and dependent systems. Use testing frameworks like Jest (Node.js) or Pytest (Python).
- Load Testing: Tests API under stress to identify breaking points. Tools: JMeter, Locust.
- Security Testing: Identifies vulnerabilities such as unauthorized access or injection attacks. Tools: OWASP ZAP, Burp Suite.
- Automated Testing: Write test suites for CI/CD pipelines to validate endpoints automatically.
Test example:
import requests
def test_get_users():
response = requests.get('https://api.example.com/users')
assert response.status_code == 200
assert 'application/json' in response.headers['Content-Type']
Evaluating Responses:
Look for a clear understanding of testing types and tools. Strong candidates will emphasize automation and mention edge cases like invalid inputs or large payloads.
11. What is CORS, and how would you handle cross-origin requests in your API?
Question Explanation:
CORS (Cross-Origin Resource Sharing) is a key concept in API security and functionality. This API Developer interview question evaluates a candidate’s understanding of handling cross-origin requests and preventing security vulnerabilities.
Expected Answer:
CORS is a mechanism that allows restricted resources on a web server to be accessed from a different origin (domain, protocol, or port). By default, browsers block such requests for security reasons.
To handle CORS:
- Enable Specific Origins: Configure the server to allow trusted origins using the
Access-Control-Allow-Origin
header. Example:Access-Control-Allow-Origin: https://trusted-client.com
- Define Allowed Methods and Headers: Use
Access-Control-Allow-Methods
andAccess-Control-Allow-Headers
to permit specific HTTP methods and headers. - Preflight Requests: Handle OPTIONS requests for preflight checks by returning appropriate headers.
Example (Express.js):
const cors = require('cors');
app.use(cors({ origin: 'https://trusted-client.com' }));
Evaluating Responses:
Look for clear explanations of CORS headers and handling of preflight requests. Bonus points for awareness of security risks, like wildcarding (*
) sensitive origins, and for suggesting tools like middleware.
12. Describe how you would document an API. What tools or techniques do you use to ensure clarity for developers consuming the API?
Question Explanation:
Clear API documentation improves usability for developers. This API Developer interview question assesses a candidate’s knowledge of tools and best practices for creating effective API documentation.
Expected Answer:
To document an API effectively:
- Use Tools: Tools like Swagger (OpenAPI), Postman, or API Blueprint help generate and visualize API documentation.
- Include Key Information: Document endpoints, methods, parameters, request/response bodies, authentication requirements, and error codes.
- Provide Examples: Include example requests and responses for each endpoint in popular languages or cURL commands.
- Interactive Documentation: Provide interactive sandboxes where developers can test endpoints directly (e.g., Swagger UI).
Best practices:
- Keep documentation up-to-date with API changes.
- Organize content for easy navigation (e.g., by resource or feature).
- Include a “getting started” section for onboarding.
Evaluating Responses:
Look for practical suggestions and awareness of developer experience. Strong candidates should emphasize automation (e.g., generating docs from code) and mention the importance of real-world examples and consistent updates.
13. What is an API gateway, and how does it differ from a reverse proxy?
Question Explanation:
This API Developer interview question evaluates the candidate’s understanding of API architecture and the role of API gateways and reverse proxies in managing and routing traffic.
Expected Answer:
An API Gateway is a server that acts as an entry point for API clients, managing tasks such as authentication, rate limiting, routing, and load balancing. It provides a unified interface for clients, often aggregating responses from multiple microservices.
A Reverse Proxy, on the other hand, is primarily a traffic management tool. It routes client requests to different backend servers and can perform tasks like SSL termination and caching. Unlike an API gateway, it doesn’t include API-specific features like versioning, request transformation, or authentication.
Key Differences:
- Functionality: API gateways handle API-specific concerns, while reverse proxies focus on general web traffic routing.
- Features: API gateways include advanced features like request transformation and analytics.
- Use Case: Use an API gateway for managing APIs; use a reverse proxy for general server requests.
Evaluating Responses:
Look for clear differentiation between the two concepts, with emphasis on use cases and examples. Bonus points for mentioning tools like Kong or AWS API Gateway for API gateways and NGINX for reverse proxies.
14. How would you ensure backward compatibility when updating an API?
Question Explanation:
Backward compatibility ensures existing clients continue to function when an API is updated. This API Developer interview question assesses the candidate’s ability to manage changes while maintaining reliability.
Expected Answer:
To ensure backward compatibility:
- API Versioning: Use versioning strategies like URI versioning (
/v1/resource
), header-based versioning, or query parameters (?version=1
). - Deprecation Policy: Clearly communicate deprecated features and provide a timeline for their removal.
- Non-Breaking Changes: Add new fields instead of modifying existing ones, and ensure default behavior remains unchanged for older clients.
- Feature Toggles: Gradually roll out new features while retaining older ones.
Best Practices:
- Write comprehensive tests to validate compatibility.
- Monitor client behavior after updates to identify potential issues.
Evaluating Responses:
Look for practical strategies and an understanding of client impact. Bonus points for addressing documentation updates and using feature toggles or A/B testing to minimize disruption.
15. What are webhooks, and how are they different from standard API endpoints? Can you give an example of their implementation?
Question Explanation:
This API Developer interview question assesses the candidate’s understanding of webhooks and how they differ from traditional API calls, focusing on their use in real-time integrations.
Expected Answer:
Webhooks are a way for servers to notify clients in real-time when specific events occur. Unlike standard API endpoints, which require the client to poll the server, webhooks allow the server to send data to a pre-configured URL whenever an event is triggered.
Example:
- A payment gateway (e.g., Stripe) sends a webhook to notify a client application when a transaction is completed.
Implementation:
- Clients register a callback URL with the server.
- The server sends an HTTP POST request to the callback URL when the event occurs.
- The client processes the payload sent by the server.
Example in Express.js:
app.post('/webhook', (req, res) => {
const event = req.body;
console.log('Received webhook event:', event);
res.status(200).send('Webhook received');
});
Evaluating Responses:
Good answers should demonstrate an understanding of how webhooks work and their advantages, such as real-time updates. Look for mention of security considerations, such as verifying webhook signatures to prevent spoofing.
API Developer Interview Questions Conclusion
Conducting interviews for API developers requires a mix of theoretical and practical questions to assess their knowledge and hands-on expertise. The 15 questions above cover core concepts, design principles, and best practices crucial for API development. By using these questions, you can identify candidates who possess the technical proficiency and problem-solving skills necessary to build and maintain robust APIs.