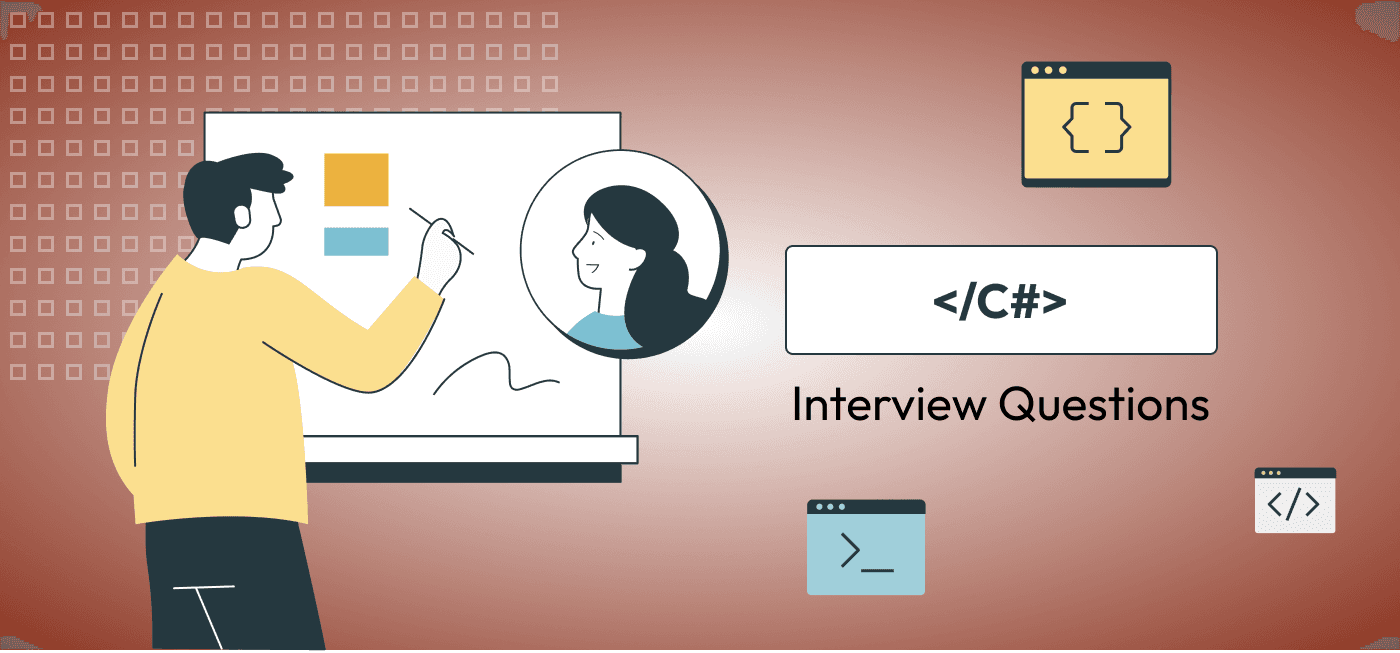
Hiring + recruiting | Blog Post
15 C# Interview Questions for Hiring C# Developers
Todd Adams
Share this post
Hiring the right C# developer requires carefully crafted interview questions that test a candidate’s grasp of core concepts, object-oriented programming, .NET integration, and practical problem-solving skills. This set of questions is designed to evaluate a developer’s knowledge and proficiency in C# and their ability to apply it in real-world scenarios.
C# Interview Questions
1. What is the difference between abstract
classes and interfaces
in C#?
Question Explanation:
This C# interview question assesses a candidate’s understanding of key object-oriented programming principles and their ability to choose between abstract
classes and interfaces
for different scenarios.
Expected Answer:
Abstract
classes and interfaces
are both used to define a contract for derived classes, but they have fundamental differences:
- Abstract Classes:
- Can have both abstract methods (no implementation) and concrete methods (with implementation).
- Can include fields, properties, constructors, and access modifiers.
- A class can inherit only one abstract class due to single inheritance.
- Interfaces:
- Can only contain method signatures, properties, events, and indexers until C# 8.0 introduced default implementations.
- Does not allow fields or constructors.
- A class can implement multiple interfaces, promoting multiple inheritance.
Example:
public abstract class Animal {
public abstract void Speak(); // Abstract method
public void Eat() { // Concrete method
Console.WriteLine("Eating...");
}
}
public interface IWalk {
void Walk(); // Interface method
}
public class Dog : Animal, IWalk {
public override void Speak() {
Console.WriteLine("Woof!");
}
public void Walk() {
Console.WriteLine("Walking...");
}
}
Evaluating Responses:
Look for clarity on:
- The primary features of
abstract
classes and interfaces. - The practical scenario where each is preferred.
- Awareness of the changes in interfaces in C# 8.0.
2. Explain the async
and await
keywords in C#. How do they work?
Question Explanation:
This C# interview question examines a candidate’s knowledge of asynchronous programming, which is essential for creating responsive and scalable applications.
Expected Answer:
- The
async
keyword is used to define an asynchronous method that allows non-blocking operations. - The
await
keyword is used to wait asynchronously for a task to complete without blocking the calling thread. - Together, they allow applications to handle tasks like I/O operations, API calls, or long-running computations efficiently.
Example:
public async Task FetchDataAsync() {
Console.WriteLine("Starting data fetch...");
await Task.Delay(2000); // Simulate delay
Console.WriteLine("Data fetch completed.");
}
- In this example,
await Task.Delay(2000)
releases the calling thread, allowing other operations to run while waiting for the delay to complete.
Evaluating Responses:
Ensure the candidate:
- Clearly understands the distinction between synchronous and asynchronous programming.
- Can explain potential pitfalls like deadlocks and best practices, such as avoiding
async void
methods except for event handlers.
3. How does garbage collection work in .NET, and how does it impact C# applications?
Question Explanation:
This C# interview question tests the candidate’s understanding of memory management, a critical aspect of application performance and stability.
Expected Answer:
- The .NET garbage collector (GC) manages memory automatically by reclaiming unused objects. It works in generations:
- Generation 0: Short-lived objects (e.g., temporary variables).
- Generation 1: Medium-lived objects.
- Generation 2: Long-lived objects (e.g., static data).
- GC operates in three phases:
- Mark: Identifies objects in use.
- Sweep: Reclaims memory of unused objects.
- Compact: Reorganizes memory for efficiency.
- Developers can influence GC using the
Dispose
pattern, finalizers, andGC.Collect()
(use sparingly).
Example:
class Program : IDisposable {
private bool disposed = false;
public void Dispose() {
if (!disposed) {
// Release resources
disposed = true;
}
}
}
Evaluating Responses:
Look for understanding of:
- Generational garbage collection and its benefits.
- Best practices, like avoiding manual GC invocation unless necessary.
- Awareness of how unmanaged resources are handled.
4. Can you explain the difference between IEnumerable
, ICollection
, and IList
in C#?
Question Explanation:
This C# interview question assesses knowledge of collection interfaces and their appropriate usage.
Expected Answer:
IEnumerable
:- Represents a sequence of elements that can be enumerated using
foreach
. - Read-only and does not support direct indexing.
- Suitable for simple iteration over data.
- Represents a sequence of elements that can be enumerated using
ICollection
:- Inherits from
IEnumerable
and adds functionalities likeCount
,Add
,Remove
, andContains
. - Suitable for managing a collection with additional operations.
- Inherits from
IList
:- Inherits from
ICollection
and adds index-based access and manipulation. - Allows accessing elements by index (
list[0]
) and modifying their values.
- Inherits from
Example:
IEnumerable<int> numbers = new List<int> { 1, 2, 3 };
foreach (var number in numbers) {
Console.WriteLine(number);
}
ICollection<int> collection = new List<int>();
collection.Add(1);
collection.Remove(1);
IList<int> list = new List<int> { 1, 2, 3 };
int first = list[0]; // Index access
list[1] = 10; // Modify element
Evaluating Responses:
Ensure the candidate:
- Correctly identifies the roles and limitations of each interface.
- Provides practical examples of their usage.
- Demonstrates an understanding of when to use each interface based on application needs.
5. What is the difference between ref
and out
parameters in C#?
Question Explanation:
This C# interview question evaluates a candidate’s understanding of advanced parameter-passing techniques in C#, specifically scenarios where values need to be modified or initialized outside a method.
Expected Answer:
ref
Parameter:- Requires the variable to be initialized before being passed to the method.
- The method can modify the value, and the changes persist outside the method.
out
Parameter:- Does not require the variable to be initialized before being passed.
- The method must assign a value to the parameter before it returns.
Example:
void ModifyRef(ref int number) {
number += 10; // Modify the value
}
void ModifyOut(out int number) {
number = 20; // Assign value before returning
}
int refNum = 5;
ModifyRef(ref refNum); // Requires initialization
Console.WriteLine(refNum); // Output: 15
int outNum; // No initialization needed
ModifyOut(out outNum);
Console.WriteLine(outNum); // Output: 20
Evaluating Responses:
Look for:
- Correct identification of initialization requirements.
- Understanding of scenarios for each (e.g.,
ref
for modifications,out
for providing multiple outputs). - Clear examples demonstrating their usage.
6. How does C# support dependency injection? Can you give an example?
Question Explanation:
This C# interview question tests a candidate’s knowledge of dependency injection (DI), a core concept in modern software design for achieving loosely coupled and testable code.
Expected Answer:
- Dependency injection is a design pattern where dependencies are provided to a class, rather than the class creating them internally.
- C# supports DI through constructor injection, property injection, and method injection.
- DI frameworks like ASP.NET Core’s built-in DI container, Autofac, and Ninject simplify dependency management.
Example: Constructor Injection
public interface IMessageService {
void SendMessage(string message);
}
public class EmailService : IMessageService {
public void SendMessage(string message) {
Console.WriteLine($"Email sent: {message}");
}
}
public class Notification {
private readonly IMessageService _messageService;
public Notification(IMessageService messageService) {
_messageService = messageService;
}
public void Notify(string message) {
_messageService.SendMessage(message);
}
}
// In an ASP.NET Core application, this could be registered as:
// services.AddScoped<IMessageService, EmailService>();
Evaluating Responses:
Assess for:
- Clear understanding of DI principles.
- Practical implementation examples (constructor injection is most common).
- Awareness of how DI frameworks simplify dependency management.
7. What is LINQ, and how is it used in C#? Provide an example.
Question Explanation:
This C# interview question assesses a candidate’s familiarity with LINQ (Language Integrated Query), a feature in C# for querying and manipulating data in a concise and readable manner.
Expected Answer:
- LINQ integrates query capabilities directly into C# using a syntax similar to SQL.
- It can be used to query collections, databases (via LINQ to SQL/Entity Framework), XML, and other data sources.
- LINQ queries can be written in two styles: query syntax or method syntax.
Example:
int[] numbers = { 1, 2, 3, 4, 5 };
// Query syntax
var evenNumbersQuery = from num in numbers
where num % 2 == 0
select num;
// Method syntax
var evenNumbersMethod = numbers.Where(num => num % 2 == 0);
// Display results
foreach (var num in evenNumbersQuery) {
Console.WriteLine(num); // Output: 2, 4
}
Evaluating Responses:
Look for:
- A clear explanation of LINQ’s purpose and benefits (e.g., readability, integration with C#).
- Examples demonstrating practical usage.
- Awareness of LINQ’s capabilities for querying different data sources.
8. Explain the concept of delegates in C#. How do they differ from events?
Question Explanation:
This C# interview question evaluates the candidate’s understanding of delegates and events, which are key to implementing callback functions and event-driven programming in C#.
Expected Answer:
- Delegates:
- A delegate is a type that holds references to methods with a specific signature.
- It allows methods to be passed as parameters, enabling callback functionality.
- Events:
- An event is a wrapper around a delegate that restricts direct invocation.
- It is used in event-driven programming to signal state changes or actions.
Example:
// Delegate
public delegate void Notify(string message);
// Delegate usage
public class DelegateExample {
public Notify NotificationHandler;
public void NotifyCaller(string message) {
NotificationHandler?.Invoke(message);
}
}
// Event
public class EventExample {
public event Notify NotificationEvent;
public void TriggerEvent(string message) {
NotificationEvent?.Invoke(message);
}
}
// Usage
var delegateExample = new DelegateExample();
delegateExample.NotificationHandler = (msg) => Console.WriteLine(msg);
delegateExample.NotifyCaller("Delegate invoked!");
var eventExample = new EventExample();
eventExample.NotificationEvent += (msg) => Console.WriteLine(msg);
eventExample.TriggerEvent("Event triggered!");
Evaluating Responses:
Look for:
- Clear distinction between delegates and events (e.g., direct invocation for delegates vs. event encapsulation).
- Practical examples of both concepts.
- Awareness of when to use each in application development.
9. What is the Dispose
pattern in C#, and why is it important?
Question Explanation:
This C# interview question evaluates a candidate’s understanding of resource management in C#, specifically the proper release of unmanaged resources to prevent memory leaks.
Expected Answer:
- The
Dispose
pattern is implemented using theIDisposable
interface, allowing developers to explicitly release unmanaged resources like file handles, database connections, or network sockets. - The
Dispose
method is used for manual cleanup, and thefinalizer
(destructor) acts as a safety net ifDispose
is not called.
Example:
public class ResourceHandler : IDisposable {
private bool disposed = false;
public void UseResource() {
if (disposed)
throw new ObjectDisposedException("ResourceHandler");
Console.WriteLine("Using resource...");
}
public void Dispose() {
Dispose(true);
GC.SuppressFinalize(this); // Prevent finalizer call
}
protected virtual void Dispose(bool disposing) {
if (!disposed) {
if (disposing) {
// Release managed resources
Console.WriteLine("Disposing managed resources...");
}
// Release unmanaged resources
Console.WriteLine("Disposing unmanaged resources...");
disposed = true;
}
}
~ResourceHandler() {
Dispose(false);
}
}
Evaluating Responses:
- Look for a clear explanation of the purpose of
Dispose
andfinalizers
. - Ensure awareness of
GC.SuppressFinalize()
and why it’s used. - Assess examples showing both managed and unmanaged resource cleanup.
10. Can you describe what Extension Methods
are and give a practical example?
Question Explanation:
This C# interview question assesses knowledge of C#’s ability to enhance existing classes without modifying their source code.
Expected Answer:
- Extension methods are static methods defined in static classes that allow adding new functionality to existing types, including classes and interfaces, without altering their definition.
- They use the
this
keyword before the first parameter to associate the method with the target type.
Example:
public static class StringExtensions {
public static bool IsPalindrome(this string str) {
var reversed = new string(str.Reverse().ToArray());
return string.Equals(str, reversed, StringComparison.OrdinalIgnoreCase);
}
}
// Usage
string word = "level";
bool isPalindrome = word.IsPalindrome();
Console.WriteLine($"Is '{word}' a palindrome? {isPalindrome}"); // Output: True
Evaluating Responses:
- Look for a proper explanation of how extension methods work.
- Assess understanding of their practical applications (e.g., enhancing libraries or frameworks).
- Ensure clarity on their limitations, such as the inability to override existing methods.
11. How does C# implement polymorphism?
Question Explanation:
This C# interview question tests a candidate’s understanding of one of the foundational principles of object-oriented programming: polymorphism, which enables flexibility and reusability in code.
Expected Answer:
- Polymorphism allows a method or object to behave differently based on the context.
- Compile-time polymorphism (method overloading) is achieved by defining multiple methods with the same name but different signatures in the same class.
- Run-time polymorphism (method overriding) is implemented using virtual methods and inheritance.
Example:
// Compile-time polymorphism
public class Calculator {
public int Add(int a, int b) => a + b;
public double Add(double a, double b) => a + b;
}
// Run-time polymorphism
public class Animal {
public virtual void Speak() => Console.WriteLine("Animal speaks.");
}
public class Dog : Animal {
public override void Speak() => Console.WriteLine("Dog barks.");
}
// Usage
Calculator calc = new Calculator();
Console.WriteLine(calc.Add(2, 3)); // Output: 5
Console.WriteLine(calc.Add(2.5, 3.5)); // Output: 6
Animal animal = new Dog();
animal.Speak(); // Output: Dog barks
Evaluating Responses:
- Ensure the candidate explains both compile-time and run-time polymorphism.
- Look for proper examples showing method overloading and method overriding.
- Assess understanding of scenarios where polymorphism enhances flexibility.
12. What are nullable value types in C#, and when should you use them?
Question Explanation:
This C# interview question evaluates the candidate’s ability to handle nullable data and avoid runtime exceptions caused by null values.
Expected Answer:
- Nullable value types (
T?
) allow value types (e.g.,int
,bool
) to hold anull
value. - Useful when representing data that may be undefined, such as database fields or optional configuration values.
- Can be checked for null using
HasValue
or compared directly tonull
.
Example:
int? nullableInt = null;
if (nullableInt.HasValue) {
Console.WriteLine($"Value: {nullableInt.Value}");
} else {
Console.WriteLine("No value");
}
// Using null-coalescing operator
int result = nullableInt ?? 0; // Default to 0 if null
Console.WriteLine(result); // Output: 0
Evaluating Responses:
- Look for a clear explanation of why nullable types are essential.
- Ensure the candidate demonstrates how to check and handle null values effectively.
- Assess understanding of best practices, such as default values using the null-coalescing operator.
13. Explain the difference between String
and StringBuilder
in C#.
Question Explanation:
This C# interview question assesses a candidate’s understanding of immutable and mutable string handling in C#, which has significant performance implications in scenarios involving frequent string manipulation.
Expected Answer:
String
:- Immutable: Once a string is created, it cannot be modified. Any operation that appears to modify a string (e.g., concatenation) creates a new string in memory.
- Suitable for operations where the value of a string changes infrequently.
StringBuilder
:- Mutable: Designed for scenarios requiring frequent modifications to a string’s value.
- Offers methods like
Append
,Insert
, andReplace
for efficient string manipulation.
Example:
// String (Immutable)
string str = "Hello";
str += " World"; // Creates a new string in memory
Console.WriteLine(str);
// StringBuilder (Mutable)
StringBuilder sb = new StringBuilder("Hello");
sb.Append(" World"); // Modifies the existing object
Console.WriteLine(sb.ToString());
Evaluating Responses:
- Ensure understanding of immutability in
String
and its performance implications. - Look for practical scenarios where
StringBuilder
is preferred. - Assess examples that clearly demonstrate the differences in memory usage and performance.
14. What is the purpose of the Task
class in C#, and how is it different from Thread
?
Question Explanation:
This C# interview question evaluates the candidate’s knowledge of concurrency and parallelism in C#, specifically the modern task-based asynchronous programming model.
Expected Answer:
Task
:- Represents an asynchronous operation that can run in parallel without blocking the main thread.
- Managed by the Task Parallel Library (TPL), allowing easier scheduling and cancellation.
- Supports chaining using
ContinueWith
orasync/await
for readable asynchronous code.
Thread
:- Represents a low-level unit of execution that runs independently.
- Requires explicit management, which can lead to complex code.
Example:
// Using Task
Task.Run(() => {
Console.WriteLine("Task running asynchronously.");
});
// Using Thread
Thread thread = new Thread(() => {
Console.WriteLine("Thread running.");
});
thread.Start();
Key Difference:
- Task: High-level abstraction with built-in thread pooling for efficiency.
- Thread: Low-level API for direct control over execution.
Evaluating Responses:
- Look for understanding of how
Task
simplifies concurrency. - Ensure awareness of scenarios where threads may still be necessary (e.g., long-running background tasks).
- Assess examples that show practical use cases of
Task
andThread
.
15. How do you implement error handling in C#? Can you describe best practices?
Question Explanation:
This C# interview question tests a candidate’s knowledge of exception handling mechanisms and their ability to write robust, maintainable code.
Expected Answer:
- Error handling in C# is implemented using
try
,catch
,finally
, andthrow
. - Best practices include:
- Catching only specific exceptions to avoid masking issues.
- Avoiding empty catch blocks.
- Using
finally
for cleanup tasks. - Logging errors for diagnostic purposes.
Example:
try {
int result = 10 / int.Parse("0");
} catch (DivideByZeroException ex) {
Console.WriteLine($"Error: {ex.Message}");
} catch (FormatException ex) {
Console.WriteLine($"Invalid input: {ex.Message}");
} finally {
Console.WriteLine("Operation completed.");
}
// Re-throwing exceptions
try {
throw new InvalidOperationException("Something went wrong.");
} catch (Exception ex) {
Console.WriteLine($"Caught exception: {ex.Message}");
throw; // Re-throws the original exception
}
Evaluating Responses:
- Ensure understanding of
try-catch-finally
constructs and their appropriate use. - Look for awareness of common pitfalls, such as overcatching
Exception
or ignoring exceptions. - Assess understanding of error logging and re-throwing exceptions when necessary.