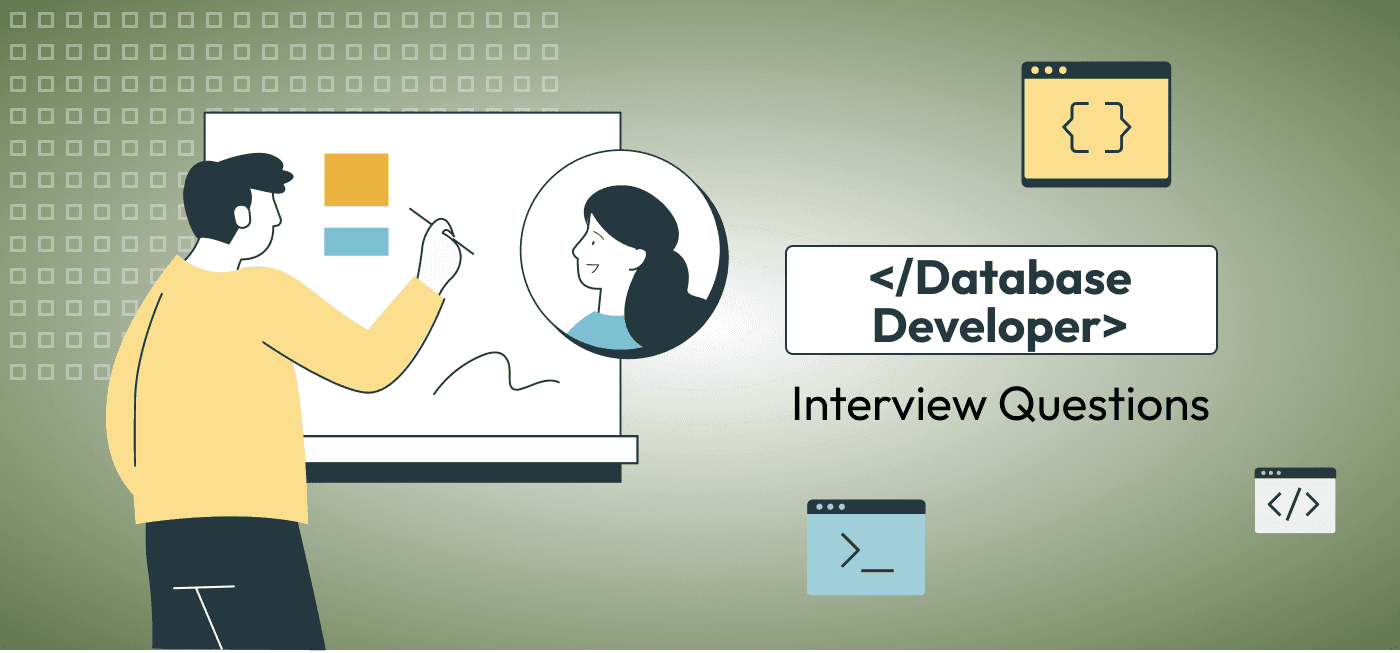
Hiring + recruiting | Blog Post
15 Database Developer Interview Questions for Hiring Database Developers
Todd Adams
Share this post
When hiring a database developer, it’s crucial to evaluate their expertise in designing, maintaining, and optimizing database systems. Strong database developers must possess a solid understanding of SQL, data modeling, database architecture, performance tuning, and other advanced database features. Below is a list of insightful interview questions tailored to assess their proficiency in these areas.
Database Developer Interview Questions
1. Can you explain the differences between a primary key and a unique key in a relational database?
Question Explanation
Understanding the distinction between a primary key and a unique key is fundamental for database design. This Database Developer interview question assesses the candidate’s knowledge of relational database constraints and their impact on ensuring data integrity.
Expected Answer
A primary key is a column or set of columns in a database table that uniquely identifies each row. It cannot contain NULL values and must be unique across all rows in the table. A table can have only one primary key.
A unique key, on the other hand, also ensures the uniqueness of values in a column or set of columns but can accept a single NULL value (depending on the database system). Unlike a primary key, a table can have multiple unique keys.
Example:
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
email VARCHAR(255) UNIQUE,
name VARCHAR(100)
);
Here, employee_id
is the primary key, while email
is a unique key.
Evaluating Responses
- Look for a clear distinction between primary and unique keys.
- Candidates should mention that a primary key is non-nullable and that unique keys can allow one NULL value.
- Bonus points if they provide examples of real-world scenarios or SQL code to illustrate the concepts.
2. What strategies do you use to optimize a slow-running SQL query? Provide examples.
Question Explanation
Optimizing SQL queries is a critical skill for database developers. This Database Developer interview question evaluates the candidate’s understanding of query optimization techniques and their ability to analyze performance issues.
Expected Answer
Several strategies can be used to optimize SQL queries:
- Indexing: Ensure appropriate indexes are in place for columns used in WHERE clauses, JOIN conditions, and ORDER BY statements.
- Query Analysis: Use tools like
EXPLAIN
orEXPLAIN PLAN
to identify inefficiencies. - *Avoid SELECT : Fetch only the necessary columns to reduce overhead.
- Optimize Joins: Use indexed joins and ensure join conditions are efficient.
- Partitioning and Sharding: Partition large tables or distribute data across multiple nodes to improve query performance.
- Caching: Cache frequently accessed results.
- Rewrite Queries: Sometimes, restructuring the query or using Common Table Expressions (CTEs) can lead to better performance.
Example of indexing:
CREATE INDEX idx_lastname ON employees (last_name);
Evaluating Responses
- Strong candidates will mention multiple optimization techniques.
- Look for an understanding of how and why certain methods improve performance.
- Bonus points for mentioning tools like
EXPLAIN
,ANALYZE
, or real-world scenarios they have encountered.
3. Describe the process of normalizing a database. Why is it important, and what are the potential downsides?
Question Explanation
Normalization is a core concept in database design. This Database Developer interview question assesses the candidate’s understanding of how to design efficient and consistent schemas.
Expected Answer
Normalization is the process of organizing a database to reduce redundancy and improve data integrity. It involves dividing a database into multiple related tables and defining relationships between them.
The steps of normalization include:
- First Normal Form (1NF): Eliminate duplicate columns and ensure each column contains atomic values.
- Second Normal Form (2NF): Remove partial dependencies, ensuring every column depends on the entire primary key.
- Third Normal Form (3NF): Eliminate transitive dependencies, ensuring non-key columns depend only on the primary key.
Importance:
Normalization prevents data anomalies, saves storage by eliminating redundancy, and ensures consistency in the data.
Downsides:
Over-normalization can lead to performance issues due to excessive JOIN operations, making data retrieval slower.
Evaluating Responses
- Look for a clear explanation of the normalization process and its goals.
- Strong candidates should also acknowledge the trade-offs, such as the impact on query performance.
- Bonus points for providing examples of normalized and denormalized schemas.
4. What is a stored procedure, and how does it differ from a function? When would you use each?
Question Explanation
Stored procedures and functions are critical tools for encapsulating database logic. This Database Developer interview question tests the candidate’s ability to understand and differentiate these constructs.
Expected Answer
A stored procedure is a precompiled set of SQL statements stored in the database that performs a specific task. It can accept input parameters, execute SQL code, and return multiple results (or none). Stored procedures are often used for complex operations, batch processing, or data manipulation tasks.
A function, on the other hand, is a database object that performs calculations and returns a single value or table. Functions can be used in SQL queries and cannot modify database state.
Key differences:
- Output: Procedures can return multiple values; functions return only one.
- Side Effects: Functions are generally read-only, while procedures can perform data updates.
- Usage in Queries: Functions can be used in SELECT statements; procedures cannot.
Example of a procedure:
CREATE PROCEDURE UpdateSalary(IN emp_id INT, IN increment DECIMAL(10,2))
BEGIN
UPDATE employees
SET salary = salary + increment
WHERE employee_id = emp_id;
END;
Example of a function:
CREATE FUNCTION GetEmployeeCount() RETURNS INT
BEGIN
RETURN (SELECT COUNT(*) FROM employees);
END;
Evaluating Responses
- Look for a clear explanation of the differences between stored procedures and functions.
- Candidates should provide practical examples of when to use each.
- Bonus points for discussing limitations or best practices in using procedures and functions.
5. Can you explain indexing? What are the different types of indexes, and how do they impact database performance?
Question Explanation
Indexing is a fundamental concept that significantly impacts database performance. This Database Developer interview question tests the candidate’s understanding of how indexes work and their ability to leverage them effectively in real-world scenarios.
Expected Answer
An index is a database object that improves the speed of data retrieval operations. It acts as a pointer or lookup table, allowing the database to locate rows more quickly than a full table scan.
Types of Indexes:
- Clustered Index: Reorders the rows in the table based on the index key, physically storing data in sorted order. A table can have only one clustered index.
- Non-Clustered Index: Creates a separate structure that points to the rows in the table without changing their physical order.
- Unique Index: Ensures that all values in the indexed column(s) are unique.
- Full-Text Index: Optimized for text-search queries.
- Composite Index: Created on multiple columns to optimize queries using a combination of these columns.
- Bitmap Index: Used in low-cardinality scenarios, often in data warehouses.
Impact on Performance:
Indexes speed up read operations by reducing the number of rows scanned. However, they add overhead to write operations like INSERT, UPDATE, and DELETE, as the indexes need to be updated alongside the table.
Example of creating an index:
CREATE INDEX idx_lastname ON employees (last_name);
Evaluating Responses
- Look for a clear explanation of how indexes improve query performance.
- Candidates should mention the trade-offs, such as slower writes and increased storage.
- Bonus points for discussing how to choose the appropriate type of index for specific use cases.
6. What is ACID compliance, and why is it critical in database transactions?
Question Explanation
ACID (Atomicity, Consistency, Isolation, Durability) compliance ensures reliability and integrity in database transactions. This Database Developer interview question evaluates the candidate’s understanding of transactional systems and their importance in database management.
Expected Answer
ACID compliance consists of the following principles:
- Atomicity: Ensures that a transaction is all-or-nothing. If any part fails, the entire transaction is rolled back.
- Consistency: Ensures that a transaction takes the database from one valid state to another, maintaining data integrity.
- Isolation: Ensures that concurrent transactions do not interfere with each other, providing consistent results.
- Durability: Ensures that once a transaction is committed, its changes are permanent, even in the event of a crash.
Importance:
ACID compliance is critical for ensuring data reliability, especially in applications like banking, e-commerce, and inventory systems where data integrity is paramount. Without ACID, transactions could lead to data corruption or inconsistencies.
Evaluating Responses
- Look for a clear definition of each ACID property.
- Strong candidates will provide real-world examples, such as banking systems requiring atomicity for account transfers.
- Bonus points for mentioning trade-offs or challenges, such as performance impacts in highly concurrent systems.
7. How would you design a database schema for a complex e-commerce application? What factors would you consider?
Question Explanation
This Database Developer interview question assesses the candidate’s ability to design a scalable, efficient, and normalized schema while considering business requirements and potential challenges.
Expected Answer
When designing a database schema for an e-commerce application, consider the following factors:
- Entities and Relationships: Identify key entities such as Users, Products, Orders, Categories, and Inventory. Define relationships (e.g., one-to-many between Orders and Products).
- Normalization: Normalize the schema to avoid redundancy while ensuring performance is not impacted.
- Scalability: Design for future growth, including partitioning, indexing, and sharding strategies.
- Transaction Handling: Ensure ACID compliance for financial transactions.
- Reporting Needs: Create denormalized views or summary tables for analytics without affecting transactional performance.
- Security: Store sensitive data like passwords in hashed form and use encryption where needed.
- Constraints: Define primary keys, foreign keys, and indexes to ensure data integrity and performance.
Example schema snippet:
CREATE TABLE users (
user_id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(255) UNIQUE
);
CREATE TABLE orders (
order_id INT PRIMARY KEY,
user_id INT,
order_date DATETIME,
total_amount DECIMAL(10,2),
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
Evaluating Responses
- Strong answers include considerations for performance, scalability, and security.
- Look for a logical explanation of entity relationships and schema normalization.
- Bonus points for addressing specific e-commerce challenges, such as handling high-concurrency transactions.
8. Explain the concept of database partitioning. What are its benefits and challenges?
Question Explanation
Database partitioning is a strategy for handling large datasets efficiently. This Database Developer interview question tests the candidate’s understanding of how partitioning works and its trade-offs.
Expected Answer
Partitioning is the process of dividing a database table into smaller, more manageable pieces called partitions, while keeping them as part of the same logical table.
Types of Partitioning:
- Range Partitioning: Divides data based on a range of values (e.g., dates).
- Hash Partitioning: Distributes data based on a hash function, ensuring even distribution.
- List Partitioning: Divides data based on a predefined list of values.
- Composite Partitioning: Combines two or more types of partitioning.
Benefits:
- Improves query performance by scanning only relevant partitions.
- Simplifies data management by allowing maintenance at the partition level.
- Enables parallel processing and scalability.
Challenges:
- Increases complexity in database design and management.
- May result in unbalanced partitions if not designed properly.
- Some operations, like JOINs across partitions, can become slower.
Example:
CREATE TABLE sales (
sale_id INT,
sale_date DATE,
amount DECIMAL(10,2)
) PARTITION BY RANGE (sale_date) (
PARTITION p1 VALUES LESS THAN ('2023-01-01'),
PARTITION p2 VALUES LESS THAN ('2024-01-01')
);
Evaluating Responses
- Look for a clear explanation of partitioning types and benefits.
- Strong candidates should mention trade-offs and potential pitfalls.
- Bonus points for examples of how they’ve implemented partitioning in past projects.
9. How do you handle data migration between two database systems? Walk us through the key considerations.
Question Explanation
Data migration is a critical operation in many projects, such as upgrading systems or moving to a new platform. This Database Developer interview question evaluates the candidate’s ability to handle data migration while ensuring minimal downtime, data integrity, and consistency.
Expected Answer
Handling data migration involves several steps and considerations:
- Planning and Analysis:
- Understand the schema, data volume, and dependencies of both the source and target databases.
- Identify data discrepancies, compatibility issues, and required transformations.
- Data Mapping and Transformation:
- Define mapping between source and target schema.
- Use ETL (Extract, Transform, Load) tools to preprocess data if necessary.
- Migration Strategy:
- Choose between one-time migration (e.g., for smaller datasets) or incremental migration (for large, live systems).
- Use tools like
pg_dump
/pg_restore
,mysqldump
, or third-party solutions.
- Testing and Validation:
- Perform trial migrations on test environments.
- Validate data completeness and integrity using checksums or row counts.
- Execution and Monitoring:
- Schedule migrations during low-traffic periods to minimize impact.
- Monitor the migration process for errors or bottlenecks.
- Post-Migration Validation:
- Ensure the target system is fully operational.
- Perform regression testing and user acceptance testing.
Evaluating Responses
- Look for a systematic approach with attention to data integrity, downtime minimization, and testing.
- Strong candidates should discuss tools, strategies for large datasets, and handling unexpected issues.
- Bonus points for sharing examples of past migrations and challenges faced.
10. What is the difference between NoSQL and SQL databases? In what scenarios would you recommend using NoSQL?
Question Explanation
SQL and NoSQL databases have different strengths, and this Database Developer interview question evaluates the candidate’s ability to choose the appropriate type for specific use cases.
Expected Answer
SQL Databases:
- Relational and structured; data is stored in tables with rows and columns.
- Use SQL for querying and require predefined schemas.
- Suitable for applications requiring complex queries, ACID compliance, and structured data.
NoSQL Databases:
- Non-relational; data is stored in various formats (key-value pairs, documents, graphs, or wide-columns).
- Schema-less or flexible schemas.
- Offer horizontal scalability and are often optimized for specific use cases.
When to Use NoSQL:
- Applications requiring high scalability and performance (e.g., social networks, IoT, real-time analytics).
- Handling semi-structured or unstructured data, such as JSON documents or logs.
- Rapid development where schema evolution is frequent.
Example:
- Use SQL for financial systems needing strict consistency.
- Use NoSQL like MongoDB for storing user profiles with varying attributes.
Evaluating Responses
- Look for clear distinctions between SQL and NoSQL.
- Candidates should discuss trade-offs, such as the consistency vs. scalability debate.
- Bonus points for real-world examples of when they’ve chosen one over the other.
11. Can you write a SQL query to find the second-highest salary in a table without using the LIMIT
clause?
Question Explanation
This is a practical problem-solving question to evaluate the candidate’s proficiency with SQL and their ability to write queries for specific scenarios.
Expected Answer
One way to find the second-highest salary is using a subquery:
SELECT MAX(salary) AS second_highest_salary
FROM employees
WHERE salary < (SELECT MAX(salary) FROM employees);
Explanation:
- The inner query
(SELECT MAX(salary) FROM employees)
finds the highest salary. - The outer query finds the maximum salary that is less than the highest salary, effectively identifying the second-highest salary.
Alternative using ROW_NUMBER
:
WITH ranked_salaries AS (
SELECT salary, ROW_NUMBER() OVER (ORDER BY salary DESC) AS rank
FROM employees
)
SELECT salary AS second_highest_salary
FROM ranked_salaries
WHERE rank = 2;
Evaluating Responses
- Look for syntactically correct SQL queries that handle edge cases (e.g., duplicate salaries).
- Strong candidates should explain their approach clearly.
- Bonus points for using advanced techniques like window functions (
ROW_NUMBER
orRANK
).
12. Describe how you would troubleshoot and resolve deadlocks in a database system.
Question Explanation
Deadlocks occur when two or more transactions prevent each other from proceeding. This Database Developer interview question evaluates the candidate’s understanding of concurrency and their problem-solving skills in handling database conflicts.
Expected Answer
Steps to Troubleshoot Deadlocks:
- Identify the Deadlock:
- Use database logs or monitoring tools (e.g., SQL Server Profiler, MySQL’s
SHOW ENGINE INNODB STATUS
) to detect deadlocks.
- Use database logs or monitoring tools (e.g., SQL Server Profiler, MySQL’s
- Understand the Cause:
- Analyze queries to determine the conflicting resources and locking patterns.
- Identify transactions and tables involved in the deadlock.
- Resolve the Immediate Issue:
- Manually terminate one of the conflicting transactions if required.
- Retry the aborted transaction to ensure application functionality.
Preventing Deadlocks:
- Consistent Locking Order: Ensure all transactions access resources in a consistent order.
- Minimize Lock Time: Keep transactions short and avoid user interaction within a transaction.
- Use Appropriate Isolation Levels: Lower isolation levels like
READ COMMITTED
orREPEATABLE READ
may reduce deadlocks. - Implement Deadlock Retry Logic: Design applications to handle transaction rollbacks gracefully.
Evaluating Responses
- Look for a clear troubleshooting process and preventive measures.
- Strong candidates should demonstrate awareness of locking mechanisms and isolation levels.
- Bonus points for discussing tools or examples from their experience dealing with deadlocks.
13. What are the differences between clustered and non-clustered indexes? When would you use each?
Question Explanation
Indexes are essential for database performance, and understanding the distinction between clustered and non-clustered indexes is crucial. This Database Developer interview question evaluates the candidate’s knowledge of indexing and their ability to apply it effectively.
Expected Answer
Clustered Index:
- A clustered index determines the physical order of data in a table, sorting rows by the indexed column(s).
- A table can have only one clustered index because data rows can only be stored in one order.
- Example: A primary key often uses a clustered index.
Non-Clustered Index:
- A non-clustered index creates a separate structure that references the rows in the table. It does not change the physical order of the data.
- A table can have multiple non-clustered indexes.
- Example: Indexes on frequently searched columns that are not the primary key.
When to Use Each:
- Use a clustered index for columns with a unique and frequently searched value (e.g., ID fields).
- Use non-clustered indexes for columns involved in WHERE, JOIN, or ORDER BY clauses (e.g., email or last name).
Example of creating both:
CREATE TABLE employees (
employee_id INT PRIMARY KEY CLUSTERED,
last_name VARCHAR(100),
email VARCHAR(255)
);
CREATE NONCLUSTERED INDEX idx_email ON employees(email);
Evaluating Responses
- Strong answers include clear distinctions and real-world examples.
- Look for an explanation of how clustered and non-clustered indexes affect performance.
- Bonus points for discussing potential trade-offs, such as increased storage for non-clustered indexes.
14. How do you ensure data security and integrity in a database system you manage?
Question Explanation
This Database Developer interview question assesses the candidate’s understanding of database security practices and their ability to implement safeguards to protect data integrity and prevent unauthorized access.
Expected Answer
Data Security Measures:
- Access Control: Implement role-based access control (RBAC) to limit user permissions based on their roles.
- Encryption:
- Encrypt data at rest using technologies like TDE (Transparent Data Encryption).
- Encrypt data in transit using SSL/TLS.
- Authentication and Authorization: Use strong authentication methods, such as multi-factor authentication (MFA).
Data Integrity Measures:
- Constraints: Use primary keys, foreign keys, unique constraints, and check constraints to enforce data integrity at the schema level.
- Transactions: Use ACID-compliant transactions to ensure consistency.
- Auditing and Monitoring:
- Monitor database logs for suspicious activity.
- Use tools for database activity monitoring (DAM).
Example Practice:
- For a financial system, encrypt sensitive columns like credit card numbers and enforce referential integrity with foreign keys.
Evaluating Responses
- Look for comprehensive coverage of security and integrity measures.
- Strong candidates will discuss both preventive measures (e.g., encryption, RBAC) and monitoring.
- Bonus points for sharing examples from past experiences and mentioning tools like
Vault
orAWS RDS security features
.
15. What experience do you have with database replication? How do you manage data consistency across replicas?
Question Explanation
Replication is critical for scaling and ensuring high availability in modern database systems. This Database Developer interview question evaluates the candidate’s experience with replication techniques and their ability to maintain data consistency.
Expected Answer
Experience with Replication:
Replication involves copying data from one database server (primary) to another (replica). Common types include:
- Master-Slave (Primary-Replica): The primary handles writes; replicas handle reads.
- Master-Master (Active-Active): Both nodes can handle writes, often requiring conflict resolution.
- Asynchronous Replication: Replicas update after the primary commits changes, leading to potential lag.
- Synchronous Replication: Changes are committed only when all replicas acknowledge, ensuring consistency but adding latency.
Managing Data Consistency:
- Monitoring Replication Lag: Use monitoring tools to detect and address delays (e.g., MySQL’s
SHOW SLAVE STATUS
). - Conflict Resolution: For multi-master setups, implement conflict resolution policies.
- Backup and Recovery: Regularly back up the primary and replicas to ensure recovery options.
- Testing: Test failover mechanisms to ensure replicas can become primaries when needed.
Example Tool:
- In PostgreSQL, use streaming replication (
pg_basebackup
) for high availability.
Evaluating Responses
- Look for familiarity with replication types and their trade-offs.
- Strong candidates should discuss tools they’ve used (e.g., MySQL, PostgreSQL, MongoDB replication).
- Bonus points for mentioning scenarios they’ve handled, such as disaster recovery or managing replication lag.
Database Developer Interview Questions Conclusion
These 15 questions are designed to assess a database developer’s technical depth, problem-solving skills, and understanding of database systems. By exploring their approach to optimization, design, and troubleshooting, you can identify candidates capable of effectively managing and scaling your organization’s databases. The answers to these questions will help you evaluate their ability to maintain data integrity, enhance performance, and adapt to evolving database technologies.