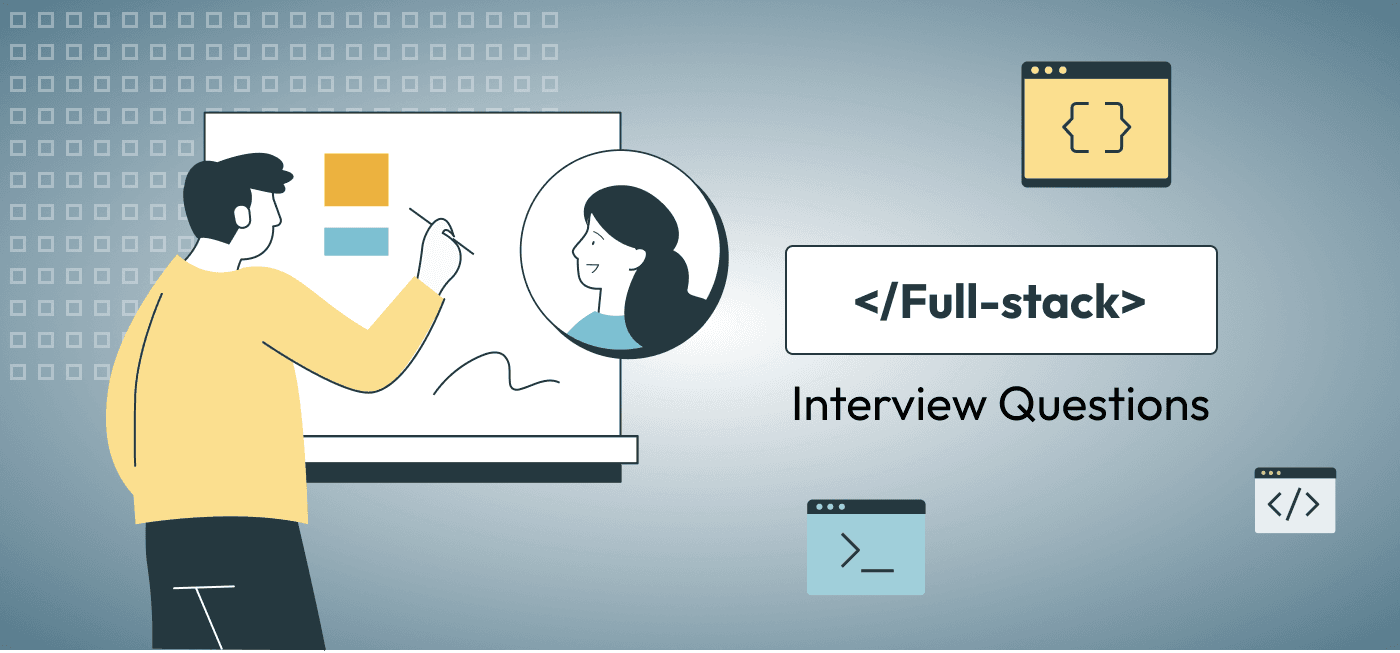
Hiring + recruiting | Blog Post
15 Full-Stack Developer Interview Questions for Hiring Full-Stack Engineers
Todd Adams
Share this post
In today’s tech landscape, full-stack developers play an essential role, bridging front-end and back-end technologies to deliver seamless applications. Hiring skilled full-stack engineers requires probing their knowledge in both front-end and back-end frameworks, database management, API integration, and overall system architecture. Below are 15 insightful questions to help assess a candidate’s competency in the multi-faceted demands of full-stack development.
Full-Stack Developer Interview Questions
1. What is your approach to structuring a full-stack application, and how do you manage dependencies between the front end and back end?
Question Explanation:
This Full-Stack Developer interview question assesses the candidate’s understanding of designing a maintainable and scalable full-stack application, along with their approach to separating concerns and managing dependencies.
Expected Answer:
A solid response should start by detailing the candidate’s preferred structure for organizing a full-stack application. They might describe dividing the application into distinct folders for front end and back end code (e.g., /client
and /server
), each managed independently for modularity. For example, in a MERN stack, a typical folder structure might look like:
project-root/
├── client/
│ ├── public/
│ ├── src/
│ ├── components/
│ ├── hooks/
│ ├── services/
├── server/
│ ├── controllers/
│ ├── models/
│ ├── routes/
│ └── utils/
For dependency management, they might discuss using tools like npm workspaces, Docker containers, or a monorepo approach to streamline shared dependencies while keeping front-end and back-end packages separate.
They should also mention tools for dependency and version management, like npm
or yarn
for JavaScript projects, and discuss strategies like semantic versioning to manage compatibility between components. For projects with shared logic (e.g., data validation), some developers use shared modules or libraries to ensure consistency across both front end and back end.
Evaluating Responses:
Look for candidates who can discuss specific architectural principles and tools and who can adapt their structure for scalability. Strong responses will include examples of when and why they chose a particular structure and how they adapted it for performance or maintainability.
2. Explain the Model-View-Controller (MVC) design pattern. How have you used it in past projects?
Question Explanation:
MVC is a fundamental design pattern in full-stack development, separating concerns into three main areas: the Model, View, and Controller. This Full-Stack Developer interview question examines the candidate’s knowledge of MVC principles and their ability to apply them effectively.
Expected Answer:
An ideal answer will include a clear breakdown of each component of MVC:
- Model: Represents the data and business logic, interacting with the database or external data sources.
- View: Handles the user interface, presenting data from the Model and receiving input from the user.
- Controller: Acts as an intermediary, processing user input from the View, updating the Model, and determining which View to render.
Candidates might explain their experience with MVC in a specific tech stack, like Node.js with Express or Ruby on Rails. For instance, they may describe using the Controller to handle route logic in Express, fetching data from MongoDB (the Model), and passing it to an EJS template or React component (the View). They could provide a concrete example of how MVC helped them keep code organized and enabled easier updates.
Evaluating Responses:
Assess their depth of understanding in how MVC separates concerns and its practical benefits, like testability and code reuse. Good candidates will give specific examples of using MVC, particularly if they can describe overcoming challenges (like handling complex data relationships in the Model).
3. How do you decide between using server-side rendering (SSR) versus client-side rendering (CSR)?
Question Explanation:
This Full-Stack Developer interview question explores the candidate’s knowledge of rendering techniques, including SSR and CSR, and when each is appropriate for performance, SEO, and user experience considerations.
Expected Answer:
A strong answer will explain that Server-Side Rendering (SSR) involves generating HTML on the server for each request, which benefits SEO and improves performance on slower devices by delivering pre-rendered content. Client-Side Rendering (CSR), on the other hand, relies on the browser to render the content, allowing for a faster initial load of the app shell but potentially slower content load times and less optimal SEO.
They might illustrate a decision process: for static sites or content-heavy pages where SEO is critical, SSR might be the preferred choice (e.g., using Next.js in a React project). For dynamic, interactive applications where frequent user interactions are key, CSR could be more efficient, using frameworks like React or Vue.
They could further explain hybrid approaches (e.g., Next.js’s support for static generation and SSR) that allow certain pages to be server-rendered while others are rendered on the client for the best of both worlds.
Evaluating Responses:
Look for an understanding of the trade-offs between SSR and CSR, including performance, SEO, and user experience. Strong candidates will also discuss frameworks that support both methods and specific use cases where they applied each approach.
4. Can you describe the process of setting up authentication and authorization in a full-stack application?
Question Explanation:
Authentication and authorization are crucial for application security. This Full-Stack Developer interview question assesses the candidate’s ability to secure user data and manage permissions across the front end and back end.
Expected Answer:
A well-rounded answer should differentiate authentication (verifying user identity) and authorization (verifying user permissions). The candidate might describe using libraries like JWT (JSON Web Tokens) for stateless authentication or OAuth for third-party integrations.
For instance, they could explain a common JWT-based workflow where a user logs in, and the server generates a token that the client stores (often in localStorage or cookies). Subsequent requests from the client include this token in the headers, which the server validates to confirm identity and access rights. They might also mention setting up role-based access controls to enforce permissions, such as differentiating admin and standard user functionalities.
On the front end, they might describe handling token storage, secure cookie usage, and conditional rendering based on user roles, ensuring that only authorized users can access specific UI elements or routes.
Evaluating Responses:
Look for a clear understanding of authentication versus authorization and specific knowledge of secure implementation practices (like storing tokens securely and preventing CSRF attacks). Strong candidates will also demonstrate familiarity with tools or libraries like Passport.js, Firebase Authentication, or Auth0 for handling these processes.
5. Describe how you handle API integrations. How do you debug or troubleshoot issues with API requests?
Question Explanation:
This Full-Stack Developer interview question assesses the candidate’s proficiency in managing external and internal APIs, as well as their approach to testing, debugging, and ensuring robust API interactions.
Expected Answer:
A thorough answer would detail the candidate’s workflow for integrating APIs, beginning with exploring API documentation to understand endpoints, request/response formats, and any authentication requirements. They may describe using tools like Postman or cURL to test requests independently before implementing them in the codebase. They should also mention how they handle responses, including parsing data and managing errors effectively with try/catch blocks and logging.
For debugging, they might explain how they monitor network requests through developer tools in the browser to check for issues such as incorrect request headers, status codes, or payload mismatches. Additionally, they may describe techniques for analyzing API responses and using logging frameworks to troubleshoot and resolve issues in a controlled environment.
A strong response would also cover handling rate limits, error response patterns, and retries, as well as applying caching strategies (e.g., storing responses to reduce repeated requests).
Evaluating Responses:
Look for an understanding of best practices for API integration, like using Postman or cURL for testing, handling rate limits, and strategies for error management. Strong candidates will provide examples of troubleshooting specific issues and demonstrate familiarity with debugging tools.
6. Explain the importance of RESTful APIs in full-stack development. How does REST compare to GraphQL?
Question Explanation:
This Full-Stack Developer interview question assesses the candidate’s knowledge of RESTful APIs and their application in full-stack development, as well as their understanding of how REST compares to GraphQL for data management.
Expected Answer:
An effective answer would describe REST as an architectural style where APIs are organized around resources and follow standard HTTP methods (GET, POST, PUT, DELETE) to manipulate these resources. RESTful APIs promote a consistent, scalable structure that’s widely understood across front-end and back-end systems, making them ideal for distributed applications.
In comparing REST and GraphQL, they should mention that GraphQL offers more flexibility, allowing clients to request only the specific data they need, reducing over-fetching and under-fetching issues that can occur with REST. This flexibility can improve performance and client-side control, especially in data-intensive applications with complex relational data.
However, the candidate should also discuss trade-offs: RESTful APIs are generally easier to cache and more straightforward for simple applications, while GraphQL requires a more complex setup and specialized tooling but can lead to a more optimized data-fetching experience for the client.
Evaluating Responses:
Look for an understanding of both REST and GraphQL, as well as practical scenarios where one might be more appropriate than the other. A strong answer will highlight REST’s simplicity and compatibility with HTTP standards, alongside GraphQL’s efficiency in handling complex data requirements.
7. What’s your experience with database management systems? Describe a scenario where you had to optimize a database query.
Question Explanation:
This Full-Stack Developer interview question evaluates the candidate’s familiarity with different types of databases, their proficiency in writing efficient queries, and their experience with performance optimization.
Expected Answer:
A detailed answer will include an overview of the candidate’s experience with relational databases (like MySQL or PostgreSQL) and NoSQL databases (such as MongoDB or Cassandra). They should mention why they used a particular type of database in a project, based on requirements like relational integrity, flexibility, or scalability.
For optimization, the candidate might describe a scenario where they encountered a slow query, explaining how they analyzed its performance (e.g., using EXPLAIN
in SQL) and identified bottlenecks. Solutions could include adding indexes on frequently queried columns, refactoring the query to reduce complexity, or implementing pagination to handle large datasets.
A strong candidate might also discuss database profiling and using ORMs (like Sequelize or Mongoose) effectively, as well as optimizing schema design by normalizing data or using caching mechanisms such as Redis for frequently accessed data.
Evaluating Responses:
Look for experience in optimizing database queries, including indexing strategies, refactoring complex queries, and understanding data normalization. Ideal candidates will describe how they identified performance bottlenecks and implemented specific solutions.
8. How do you handle asynchronous operations in JavaScript, and why is this important in full-stack applications?
Question Explanation:
Asynchronous programming is essential for full-stack applications that handle network requests, database operations, and I/O tasks. This Full-Stack Developer interview question evaluates the candidate’s knowledge of asynchronous operations and JavaScript’s non-blocking nature.
Expected Answer:
A strong answer should start by explaining that asynchronous operations allow JavaScript to execute non-blocking tasks like network requests and database operations without freezing the UI or blocking the main thread. The candidate should mention common asynchronous techniques, including callbacks, Promises, and the modern async/await
syntax, with async/await
being preferred for readability and error handling.
They might describe using async/await
to handle API requests, awaiting responses, and wrapping operations in try/catch
blocks for error handling. They could provide an example, such as:
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) throw new Error("Network response was not ok");
const data = await response.json();
return data;
} catch (error) {
console.error("Fetch error:", error);
}
}
Candidates may also discuss scenarios where Promise.all() is beneficial for executing multiple asynchronous operations in parallel, or how they handle timeouts and retries for failed requests.
Evaluating Responses:
Look for an understanding of JavaScript’s asynchronous capabilities, including async/await
, Promises, and callback handling. Strong candidates will demonstrate knowledge of handling errors, executing parallel requests, and writing code that leverages asynchronous operations for responsive, efficient applications.
9. What are some best practices for front-end state management? How do you approach state synchronization with the back end?
Question Explanation:
This Full-Stack Developer interview question assesses the candidate’s understanding of managing state effectively in a front-end application and their approach to synchronizing state with back-end data.
Expected Answer:
An ideal answer should mention common state management patterns and tools, such as React’s Context API for small to medium applications, and more advanced tools like Redux or MobX for larger applications with complex state requirements. They may discuss organizing state into local (component-level) and global (app-wide) states, aiming to minimize global state usage to improve performance and maintainability.
For synchronizing state with the back end, the candidate might describe using API calls to fetch and update data, along with strategies for handling these updates in real-time or in batches, depending on the app’s requirements. They may also discuss optimistic updates to provide immediate feedback in the UI, with rollback mechanisms in case of errors, and using tools like React Query to fetch, cache, and sync data efficiently.
A robust answer will address strategies for handling stale data and implementing periodic background syncs to keep the client state aligned with the server without overwhelming the server with frequent requests.
Evaluating Responses:
Look for an understanding of state management tools and approaches to data synchronization. Strong candidates will discuss the pros and cons of different approaches and provide examples of managing local and global state, handling real-time updates, and managing complex data flows efficiently.
10. Can you explain how you ensure responsiveness and cross-browser compatibility in your applications?
Question Explanation:
This Full-Stack Developer interview question examines the candidate’s knowledge of responsive web design principles and strategies for making applications accessible across different browsers and devices.
Expected Answer:
A comprehensive answer should begin with an explanation of responsive design techniques like using CSS media queries, flexbox, and CSS Grid to create layouts that adapt to various screen sizes and orientations. The candidate should mention using relative units like percentages, em
, and rem
to make UI elements scale proportionally, along with viewport meta tags for mobile devices.
For cross-browser compatibility, they might discuss testing on different browsers using tools like BrowserStack or CrossBrowserTesting and handling browser-specific quirks through vendor prefixes or feature detection with libraries like Modernizr. They should also mention progressive enhancement and graceful degradation, ensuring that core features work even on older or less capable browsers.
Strong answers would include techniques for mobile optimization, like deferring non-essential assets, lazy loading images, and minimizing JavaScript to improve load times on slower networks.
Evaluating Responses:
Look for familiarity with CSS layout tools, media queries, and a practical approach to handling cross-browser compatibility. Candidates should show awareness of mobile-first design, responsive best practices, and tools to verify compatibility across different browsers and devices.
11. Describe how you manage dependencies and versioning in a full-stack project.
Question Explanation:
Dependency and version management are crucial for ensuring consistency and maintainability in a full-stack project. This Full-Stack Developer interview question evaluates the candidate’s familiarity with managing packages and maintaining compatibility between different components.
Expected Answer:
A strong response should start by explaining dependency management using package managers like npm or yarn. The candidate should describe maintaining separate package.json
files for front-end and back-end dependencies or using a monorepo structure for larger projects, managed with tools like Lerna.
For version control, they might mention using Semantic Versioning (semver) to track changes between versions, as well as version-locking mechanisms (package-lock.json
in npm or yarn.lock
in Yarn) to ensure consistent installations across environments. They may also discuss automating dependency updates using tools like Dependabot or Renovate to keep dependencies current without breaking functionality.
Advanced candidates could describe how they handle dependency conflicts, resolve vulnerabilities using npm audit
, and use CI/CD pipelines to verify that updates do not introduce regressions.
Evaluating Responses:
Look for a clear understanding of dependency management tools, version control, and practices for maintaining stability across environments. Strong candidates will demonstrate a proactive approach to handling updates and conflicts, as well as strategies for automation and version-locking consistency.
12. What role does containerization (e.g., Docker) play in your development and deployment process?
Question Explanation:
This Full-Stack Developer interview question assesses the candidate’s understanding of containerization and how it benefits development and deployment, particularly in creating isolated, consistent environments for full-stack applications.
Expected Answer:
A detailed answer should begin with an explanation of containerization and how Docker allows developers to package an application with all its dependencies, creating a consistent environment across development, testing, and production. The candidate should mention using Docker to build lightweight images of front-end and back-end services, and orchestrate these with Docker Compose to handle multi-container setups for services like databases, APIs, and client-side code.
They might describe the benefits of containerization for reducing “it works on my machine” issues by ensuring that each environment is identical, regardless of the host system. Additionally, they could mention using Docker in CI/CD pipelines to build, test, and deploy applications reliably, and tools like Kubernetes for managing and scaling containers in production.
Candidates may also explain how they handle persistent storage, manage environment variables securely in Docker, and implement networking between containers for full-stack setups.
Evaluating Responses:
Look for an understanding of Docker’s role in providing isolated, consistent environments and how containerization streamlines development and deployment. Strong candidates will describe specific use cases, from local development to CI/CD integration and deployment to production, showing a clear understanding of Docker’s advantages in a full-stack setup.
13. Explain how you handle user data storage and retrieval in a secure manner.
Question Explanation:
This Full-Stack Developer interview question examines the candidate’s understanding of security best practices for storing and managing sensitive user data in a full-stack application.
Expected Answer:
An ideal answer would include securing data storage both on the client and server sides. On the server, candidates should mention hashing passwords using algorithms like bcrypt to prevent storing plain text passwords. For sensitive data, they should explain using encryption both in transit (using HTTPS) and at rest (such as encrypting database fields or using encrypted file storage). They might also mention using environment variables to store sensitive credentials securely (e.g., API keys, database credentials).
For secure data retrieval, they should discuss using role-based access control (RBAC) to restrict access based on user permissions and token-based authentication (such as JWT) to authorize users without exposing their credentials. On the client side, they might describe storing tokens securely in httpOnly cookies to prevent JavaScript access and mitigate XSS attacks.
The candidate could also touch on regular security audits to identify vulnerabilities, using tools like OWASP ZAP or Snyk, and implementing Content Security Policies (CSPs) to prevent injection attacks.
Evaluating Responses:
Look for a comprehensive understanding of security best practices, including password hashing, data encryption, and secure token storage. Strong candidates should discuss measures for securing data in transit and at rest, as well as tools or frameworks they use to ensure data security.
14. How do you test your code, both on the front end and back end, before deploying it to production?
Question Explanation:
This Full-Stack Developer interview question assesses the candidate’s approach to testing in a full-stack environment, including their familiarity with testing frameworks and practices for ensuring code quality and reliability.
Expected Answer:
A thorough answer would explain the candidate’s testing strategy, starting with unit testing to validate individual functions or components on both the front end and back end. They might mention using Jest or Mocha for JavaScript testing and tools like Enzyme or React Testing Library for front-end component testing.
For back-end testing, candidates should discuss integration testing to verify that different parts of the application work together as expected, such as testing routes and database interactions using tools like Supertest for Node.js. Additionally, they might use end-to-end (E2E) testing frameworks like Cypress or Selenium to simulate real user interactions across the full stack.
Candidates may also touch on using CI/CD pipelines to run automated tests for every code push, ensuring quality checks before deployment. They might conclude with strategies for mocking dependencies in tests and using code coverage tools to identify untested parts of the application.
Evaluating Responses:
Look for familiarity with unit, integration, and end-to-end testing approaches and tools. Strong candidates will demonstrate an understanding of testing for both front-end and back-end code, as well as knowledge of test automation in CI/CD workflows.
15. Describe a challenging bug or performance issue you’ve encountered in a full-stack project. How did you resolve it?
Question Explanation:
This Full-Stack Developer interview question probes the candidate’s problem-solving skills, particularly in handling complex bugs or performance bottlenecks in a full-stack context.
Expected Answer:
A good answer would start with a clear description of the issue. For instance, the candidate might discuss a performance bottleneck due to slow database queries, an inefficient algorithm, or network latency. They should explain how they identified the problem, such as by using profiling tools (like Chrome DevTools, Postman, or database profiling tools) or analyzing logs to pinpoint the issue.
For example, they could describe optimizing a slow database query by adding indexes, refactoring the query, or implementing caching. Alternatively, they might mention debugging a front-end issue by using network analysis to find excessive API calls and batch them, or by using lazy loading to reduce load times.
Candidates should emphasize a logical troubleshooting approach, walking through each step they took to isolate and address the issue, and conclude by describing the positive impact on the application’s performance or stability.
Evaluating Responses:
Look for clear communication of the issue and a structured approach to resolving it. Strong candidates should demonstrate technical debugging skills, use specific tools, and explain the improvements their solution achieved.
Full-Stack Developer Interview Questions Conclusion
These Full-Stack Developer interview questions are designed to reveal a candidate’s expertise across various aspects of full-stack development, from front-end UI considerations to back-end data handling and server management. By assessing their responses, you can better gauge their depth of experience, problem-solving abilities, and overall readiness to tackle the multifaceted challenges of full-stack engineering in a dynamic environment.