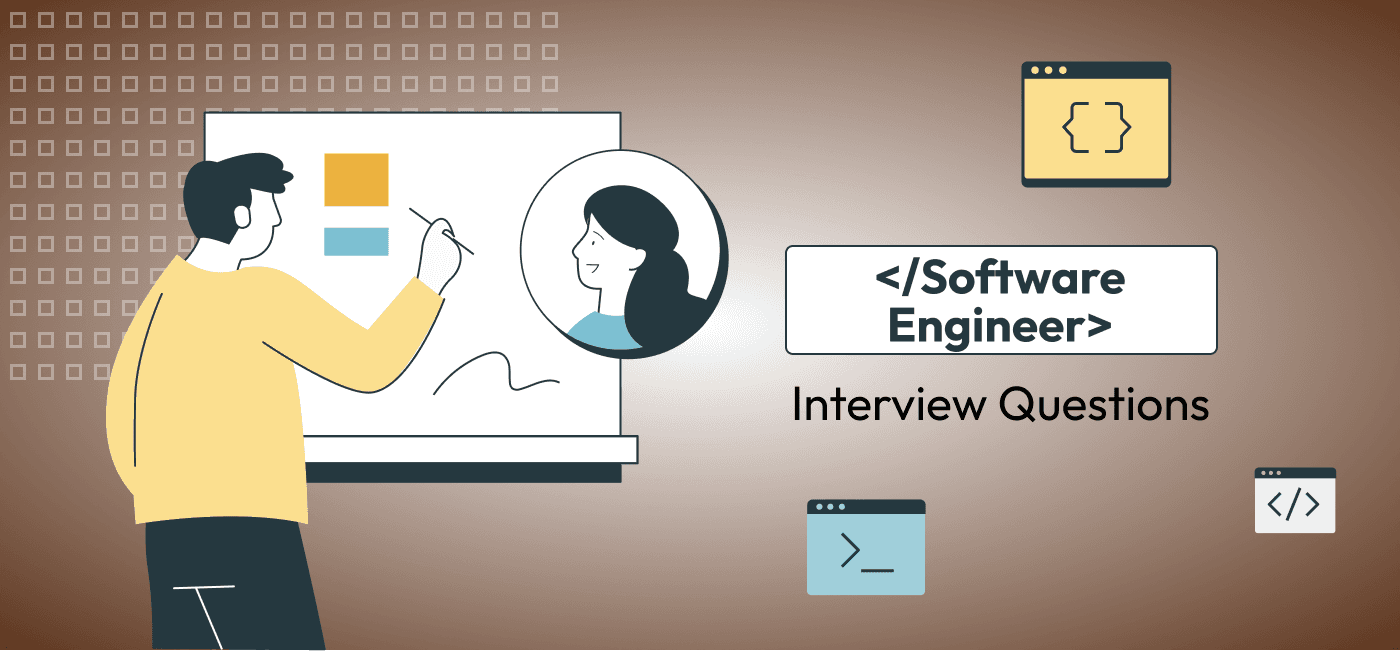
Hiring + recruiting | Blog Post
15 General Software Engineer Interview Questions for Hiring Software Engineers
Todd Adams
Share this post
When hiring for a software engineering role, it’s essential to evaluate candidates on a range of skills that cover coding proficiency, problem-solving abilities, design thinking, and familiarity with best practices in software development. This set of software engineer interview questions is designed to probe these areas, ensuring that candidates have a well-rounded skill set suitable for a dynamic and challenging engineering environment.
1. Can you describe your experience with object-oriented programming and explain its core principles?
Question Explanation: Object-oriented programming (OOP) is a fundamental programming paradigm used in software development. Understanding a candidate’s experience with OOP and their grasp of its core principles is crucial for evaluating their ability to design robust and maintainable code.
Expected Answer: Object-oriented programming is a paradigm based on the concept of “objects,” which can contain data and code to manipulate that data. The four core principles of OOP are:
- Encapsulation: This principle involves bundling the data (attributes) and the methods (functions) that operate on the data into a single unit or class. Encapsulation helps in hiding the internal state of an object from the outside world and only exposing a controlled interface. For example:
class Car:
def __init__(self, make, model):
self._make = make # _make is a protected attribute
self._model = model # _model is a protected attribute
def get_car_info(self):
return f"{self._make} {self._model}"
- Inheritance: This principle allows a class to inherit properties and methods from another class. It promotes code reusability and establishes a relationship between different classes. For example:
class Vehicle:
def __init__(self, make, model):
self.make = make
self.model = model
def get_info(self):
return f"{self.make} {self.model}"
class Car(Vehicle):
def __init__(self, make, model, doors):
super().__init__(make, model)
self.doors = doors
def get_car_info(self):
return f"{self.make} {self.model}, {self.doors} doors"
- Polymorphism: This principle allows objects to be treated as instances of their parent class rather than their actual class. The most common use is method overriding, where a child class can provide a specific implementation of a method that is already defined in its parent class. For example:
class Animal:
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return "Bark"
class Cat(Animal):
def make_sound(self):
return "Meow"
- Abstraction: This principle involves hiding the complex implementation details and showing only the essential features of the object. It helps in reducing complexity and allows focusing on interactions at a high level. For example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
Evaluating Responses: Candidates should demonstrate a clear understanding of each OOP principle and provide examples that showcase their practical application. Look for answers that reflect their experience in designing and implementing object-oriented solutions, as well as their ability to explain concepts clearly.
2. How do you approach debugging and resolving a critical issue in a production environment?
Question Explanation: Debugging is an essential skill for software engineers. This question assesses the candidate’s problem-solving abilities, their systematic approach to identifying and resolving issues, and their ability to handle high-pressure situations.
Expected Answer: When faced with a critical issue in a production environment, I follow a structured approach to debugging and resolution:
- Identify and Reproduce the Issue: The first step is to understand the problem by gathering as much information as possible. This may involve checking logs, monitoring system performance, and communicating with users experiencing the issue. Reproducing the problem in a controlled environment is crucial for effective debugging.
- Isolate the Cause: Once the issue is reproducible, I start isolating the root cause. This involves checking recent changes, examining code paths, and using debugging tools to trace the execution flow. I may use breakpoints, log statements, and other techniques to narrow down the problem area.
- Implement and Test a Fix: After identifying the cause, I develop a solution and test it thoroughly in a staging environment. It’s important to ensure that the fix resolves the issue without introducing new problems. I also consider edge cases and potential impacts on other parts of the system.
- Deploy and Monitor: Once the fix is tested and reviewed, I deploy it to the production environment, usually during a maintenance window to minimize disruption. Post-deployment, I closely monitor the system to ensure the issue is resolved and the system is stable.
- Review and Document: After resolving the issue, I conduct a post-mortem to understand what went wrong and how it can be prevented in the future. This involves documenting the problem, the solution, and any lessons learned. I also update any relevant documentation and processes to improve our response to similar issues in the future.
Evaluating Responses: Look for a structured approach to problem-solving, an understanding of debugging tools and techniques, and an ability to handle pressure. Candidates should demonstrate a balance between technical skills and practical experience in resolving production issues. Communication and documentation skills are also important, as they reflect the candidate’s ability to work effectively in a team.
3. Explain the differences between a process and a thread. How do you manage concurrency in your applications?
Question Explanation: Understanding the distinction between processes and threads is fundamental to managing concurrency in software development. This software engineer interview question evaluates the candidate’s knowledge of operating system concepts and their ability to design and implement concurrent applications.
Expected Answer: A process is an independent execution unit that has its own memory space, while a thread is a smaller execution unit that shares the memory space of its parent process. Here are the key differences:
- Isolation: Processes are isolated from each other, meaning they have their own memory and resources. Threads, on the other hand, share the same memory and resources within a process, allowing for more efficient communication but increasing the risk of synchronization issues.
- Overhead: Creating and managing processes involves more overhead than threads because processes require separate memory and resource allocation. Threads are lighter and more efficient because they share the same resources.
- Communication: Inter-process communication (IPC) is more complex and slower compared to inter-thread communication. Threads can easily communicate with each other by accessing shared variables, while processes need mechanisms like pipes, sockets, or shared memory.
To manage concurrency in applications, I use several techniques and tools:
- Threading: For tasks that can run concurrently within the same application, I use threading. In Python, for example, I might use the
threading
module:
import threading
def print_numbers():
for i in range(10):
print(i)
def print_letters():
for letter in 'abcdefghij':
print(letter)
t1 = threading.Thread(target=print_numbers)
t2 = threading.Thread(target=print_letters)
t1.start()
t2.start()
t1.join()
t2.join()
- Multiprocessing: For CPU-bound tasks that benefit from parallel execution, I use multiprocessing to take advantage of multiple CPU cores. In Python, this can be done using the
multiprocessing
module:
import multiprocessing
def worker(num):
print(f'Worker {num}')
if __name__ == '__main__':
processes = []
for i in range(5):
p = multiprocessing.Process(target=worker, args=(i,))
processes.append(p)
p.start()
for p in processes:
p.join()
- Asynchronous Programming: For I/O-bound tasks, such as network or file operations, I use asynchronous programming to improve performance and responsiveness. In Python, this can be achieved using the
asyncio
module:
import asyncio
async def fetch_data():
print('Fetching data...')
await asyncio.sleep(2)
print('Data fetched!')
async def main():
await asyncio.gather(fetch_data(), fetch_data())
asyncio.run(main())
Evaluating Responses: Candidates should clearly explain the differences between processes and threads, including their advantages and disadvantages. Look for practical examples and an understanding of when to use each approach. Knowledge of specific concurrency tools and techniques in the candidate’s preferred programming language is also important.
4. Describe a time when you had to refactor a large piece of code. What approach did you take, and what were the results?
Question Explanation: Refactoring is a critical skill for maintaining and improving code quality. This software engineer interview question assesses the candidate’s ability to systematically improve existing code, their understanding of best practices, and their impact on the project.
Expected Answer: Refactoring a large piece of code requires a careful and methodical approach to avoid introducing new issues while improving the existing codebase. Here is an example from my experience:
I was working on a legacy application that had become difficult to maintain due to its monolithic structure and lack of modularization. The code was tightly coupled, making it hard to implement new features or fix bugs without affecting other parts of the system.
- Initial Assessment: I started by conducting a thorough assessment of the codebase. This involved identifying the main pain points, such as duplicated code, large classes, and functions with multiple responsibilities. I also reviewed any existing documentation and spoke with team members to understand the most critical areas that needed improvement.
- Planning and Prioritization: Based on the assessment, I created a refactoring plan that prioritized the most critical and impactful areas. The plan included breaking down the monolithic code into smaller, more manageable modules, improving code readability, and enhancing test coverage. I also ensured that we had a comprehensive suite of automated tests to catch any regressions.
- Incremental Refactoring: I approached the refactoring incrementally, focusing on one module or functionality at a time. This allowed me to make gradual improvements without disrupting the entire codebase. For example, I refactored a large class by applying the Single Responsibility Principle, breaking it into smaller classes, each with a specific responsibility. I also replaced duplicated code with reusable functions and applied design patterns where appropriate.
- Testing and Validation: After each refactoring step, I ran the automated tests to ensure that the changes did not introduce any new issues. I also performed manual testing for critical functionalities and sought feedback from team members to validate the improvements.
- Documentation and Review: Once the refactoring was complete, I updated the documentation to reflect the changes and conducted code reviews with the team to ensure that the new structure was well-understood and maintained best practices.
Results: The refactoring effort significantly improved the maintainability and scalability of the application. The codebase became more modular, making it easier to implement new features and fix bugs. The improved structure also facilitated better collaboration within the team, as developers could work on different modules independently. Overall, the refactoring led to a more robust and flexible system, reducing technical debt and enhancing the application’s long-term viability.
Evaluating Responses: Look for a structured and methodical approach to refactoring, including assessment, planning, incremental changes, testing, and documentation. The candidate should demonstrate an understanding of best practices and the ability to improve code quality systematically. Emphasize the impact of their refactoring efforts on the project and team collaboration.
5. How do you ensure the scalability and performance of an application? Can you provide an example from your past work?
Question Explanation: Scalability and performance are critical for applications that need to handle growing amounts of work or data. This question assesses the candidate’s understanding of strategies and best practices to ensure an application can scale efficiently and perform optimally.
Expected Answer: Ensuring scalability and performance involves several strategies and techniques. Here’s an example from my past work:
- Profiling and Monitoring: The first step is to profile the application to identify performance bottlenecks. Tools like New Relic, Datadog, or built-in profilers in development environments can help. Monitoring helps track performance metrics and resource usage in real-time.
- Optimizing Code: Writing efficient code is crucial. This involves choosing appropriate algorithms and data structures, minimizing unnecessary computations, and optimizing database queries. For instance, using indexing in databases to speed up query performance.
- Caching: Implementing caching mechanisms can drastically improve performance. Caching can be done at multiple levels, including database query caching (e.g., using Redis or Memcached), application-level caching, and content delivery networks (CDNs) for static assets.
- Load Balancing: Distributing the load across multiple servers helps handle increased traffic and provides redundancy. Load balancers, like Nginx, HAProxy, or cloud-based solutions from AWS or Azure, can distribute incoming requests efficiently.
- Horizontal and Vertical Scaling: Horizontal scaling involves adding more machines to handle the load, while vertical scaling involves adding more resources (CPU, RAM) to the existing machines. Depending on the architecture, one or both approaches can be applied.
- Database Sharding: For databases that handle large volumes of data, sharding can be used to split the data across multiple databases. This helps in managing the load and improving performance.
Example: In a past project, we had a web application that started experiencing performance issues as user traffic increased. Here’s how we tackled it:
- Identified Bottlenecks: Using New Relic, we identified that our database queries were taking a long time to execute and that certain parts of our code were inefficient.
- Optimized Database Queries: We added indexes to the most frequently queried columns and optimized complex joins. This reduced the query execution time significantly.
- Implemented Caching: We implemented Redis caching for frequently accessed data, reducing the load on our database.
- Load Balancing: We set up an AWS Elastic Load Balancer to distribute incoming traffic across multiple EC2 instances, ensuring that no single server was overwhelmed.
- Scalability: We adopted horizontal scaling by adding more instances as the traffic grew and used auto-scaling groups to handle traffic spikes dynamically.
Evaluating Responses: Candidates should demonstrate a comprehensive understanding of various techniques for improving scalability and performance. Look for practical examples and a clear explanation of the steps taken. Assess their ability to identify and resolve bottlenecks, implement efficient code, and use tools and techniques effectively.
6. What are design patterns, and which ones have you used in your projects? Provide specific examples.
Question Explanation: Design patterns are standard solutions to common problems in software design. This software engineer interview question evaluates the candidate’s familiarity with design patterns, their practical application, and their ability to choose the appropriate pattern for a given scenario.
Expected Answer: Design patterns are typical solutions to recurring problems in software design. They provide a template for how to solve a problem in various contexts. Here are a few design patterns I’ve used in my projects:
- Singleton Pattern: This pattern ensures that a class has only one instance and provides a global point of access to it. I used the Singleton pattern to manage a database connection pool in a Java application, ensuring that only one instance of the connection pool exists and is shared across the application.
public class DatabaseConnection {
private static DatabaseConnection instance;
private Connection connection;
private DatabaseConnection() {
// Initialize the connection
}
public static synchronized DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
public Connection getConnection() {
return connection;
}
}
- Observer Pattern: This pattern defines a one-to-many dependency between objects, so when one object changes state, all its dependents are notified. I used the Observer pattern in a notification system where multiple services needed to be updated when a user’s status changed.
class User:
def __init__(self):
self._observers = []
def add_observer(self, observer):
self._observers.append(observer)
def notify_observers(self):
for observer in self._observers:
observer.update(self)
def set_status(self, status):
self.status = status
self.notify_observers()
class NotificationService:
def update(self, user):
print(f"User status changed to {user.status}")
user = User()
notification_service = NotificationService()
user.add_observer(notification_service)
user.set_status("Online")
- Factory Pattern: This pattern provides an interface for creating objects in a super class but allows subclasses to alter the type of objects that will be created. I used the Factory pattern to create different types of users (Admin, Guest, Member) in a web application.
public abstract class User {
// Common properties and methods
}
public class Admin extends User {
// Admin-specific properties and methods
}
public class Guest extends User {
// Guest-specific properties and methods
}
public class UserFactory {
public User createUser(String userType) {
if (userType.equals("Admin")) {
return new Admin();
} else if (userType.equals("Guest")) {
return new Guest();
} else {
return new Member();
}
}
}
Evaluating Responses: Look for a clear understanding of design patterns and their practical application. The candidate should provide specific examples from their experience and explain why they chose a particular pattern. Assess their ability to describe the benefits and potential drawbacks of using design patterns in different scenarios.
7. Discuss the importance of unit testing and test-driven development (TDD). How have you implemented these practices in your work?
Question Explanation: Unit testing and test-driven development (TDD) are critical for ensuring code quality and reliability. This question evaluates the candidate’s understanding of these practices, their benefits, and their practical implementation in software development.
Expected Answer: Unit testing involves testing individual components of a software application to ensure they work as expected. Test-driven development (TDD) is a software development approach where tests are written before the code itself. Here’s why these practices are important and how I have implemented them:
- Unit Testing:
- Importance: Unit tests help catch bugs early in the development process, ensuring that each component works correctly in isolation. They make the codebase more maintainable and refactorable, as developers can modify code with confidence that existing functionality remains unaffected.
- Implementation: In my previous projects, I used unit testing frameworks like JUnit for Java, PyTest for Python, and Jest for JavaScript. I wrote unit tests for critical functions and classes, covering both typical and edge cases.
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(0, 0) == 0
- Test-Driven Development (TDD):
- Importance: TDD ensures that the code is tested from the start, leading to better-designed, more reliable, and maintainable code. It encourages developers to think about the requirements and design before writing the implementation.
- Implementation: When following TDD, I start by writing a failing test that defines a desired improvement or new function. Then, I write the minimal code necessary to pass the test. Finally, I refactor the code while ensuring that all tests still pass.
import pytest
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
if __name__ == "__main__":
pytest.main()
Evaluating Responses: Candidates should demonstrate a clear understanding of the importance of unit testing and TDD, including their benefits for code quality and maintainability. Look for practical examples of how they have implemented these practices in their work. Assess their ability to write meaningful tests and follow the TDD process effectively.
8. Can you explain the concept of RESTful APIs and how you have utilized them in your projects?
Question Explanation: RESTful APIs are a common method for enabling communication between different software systems. This question evaluates the candidate’s understanding of REST principles and their practical experience in designing and consuming RESTful APIs.
Expected Answer: REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful APIs use HTTP requests to perform CRUD operations (Create, Read, Update, Delete) on resources. The key principles of REST include:
- Statelessness: Each request from a client to a server must contain all the information needed to understand and process the request. The server does not store any state about the client session on the server side.
- Client-Server Architecture: The client and server are separate entities. The client is responsible for the user interface, and the server handles the backend processing. This separation allows for independent development and scaling.
- Uniform Interface: RESTful APIs use standard HTTP methods (GET, POST, PUT, DELETE) and rely on a consistent set of URIs to access resources. This simplifies the API design and makes it easy to understand and use.
- Resource-Based: Everything in a RESTful API is considered a resource, identified by URIs. Resources can be represented in various formats, typically JSON or XML.
Utilization in Projects: In a previous project, I developed a RESTful API for an e-commerce platform. The API allowed clients to manage products, orders, and customers. Here’s an example of how I designed and implemented it:
- Designing Endpoints: I defined the endpoints for each resource, using clear and consistent URIs. For example:
- GET /products: Retrieve a list of products
- POST /products: Create a new product
- GET /products/{id}: Retrieve a specific product by ID
- PUT /products/{id}: Update a specific product by ID
- DELETE /products/{id}: Delete a specific product by ID
- Implementing the API: Using a framework like Flask for Python, I implemented the endpoints. For example:
from flask import Flask, request, jsonify
app = Flask(__name__)
products = []
@app.route('/products', methods=['GET'])
def get_products():
return jsonify(products)
@app.route('/products', methods=['POST'])
def create_product():
product = request.json
products.append(product)
return jsonify(product), 201
@app.route('/products/<int:id>', methods=['GET'])
def get_product(id):
product = next((p for p in products if p['id'] == id), None)
if product is None:
return jsonify({'error': 'Product not found'}), 404
return jsonify(product)
@app.route('/products/<int:id>', methods=['PUT'])
def update_product(id):
product = next((p for p in products if p['id'] == id), None)
if product is None:
return jsonify({'error': 'Product not found'}), 404
updated_product = request.json
product.update(updated_product)
return jsonify(product)
@app.route('/products/<int:id>', methods=['DELETE'])
def delete_product(id):
product = next((p for p in products if p['id'] == id), None)
if product is None:
return jsonify({'error': 'Product not found'}), 404
products.remove(product)
return '', 204
if __name__ == '__main__':
app.run(debug=True)
- Documentation: I documented the API using tools like Swagger or Postman to provide clear instructions on how to use the endpoints, including request and response formats, status codes, and examples.
Evaluating Responses: Look for a solid understanding of REST principles and practical experience in designing and implementing RESTful APIs. Candidates should be able to explain the rationale behind their design decisions and provide examples of how they have used RESTful APIs in their projects. Assess their ability to design clean, consistent, and well-documented APIs.
9. Describe your experience with version control systems, particularly Git. How do you manage branches and handle conflicts?
Question Explanation: Version control systems are essential for collaborative software development. This question assesses the candidate’s familiarity with version control, specifically Git, and their ability to manage branches and resolve conflicts.
Expected Answer: Version control systems like Git help manage changes to source code over time. Git provides powerful branching and merging capabilities, making it ideal for collaborative development. Here’s how I manage branches and handle conflicts:
- Branching Strategy:
- Feature Branches: Each new feature is developed in its own branch, typically created from the
main
ordevelop
branch. This isolates feature development and allows multiple features to be developed simultaneously without interference. - Release Branches: Before a release, a release branch is created from
develop
. This branch is used for final testing and bug fixing. Once the release is ready, the release branch is merged intomain
anddevelop
. - Hotfix Branches: For urgent bug fixes in the production environment, a hotfix branch is created from
main
. After the fix, the hotfix branch is merged back into bothmain
anddevelop
.
- Feature Branches: Each new feature is developed in its own branch, typically created from the
- Managing Branches:
Creating a Branch: To start working on a new feature or bug fix, I create a new branch:
git checkout -b feature/new-feature
Switching Branches: To switch between branches, I use:
git checkout develop
Merging Branches: After completing work on a branch, I merge it back into the base branch (e.g., develop
):
git checkout develop
git merge feature/new-feature
- Handling Conflicts:
Identifying Conflicts: Conflicts occur when changes from different branches overlap. Git highlights conflicting files during a merge attempt.
git merge feature/new-feature
# Output: CONFLICT (content): Merge conflict in file.txt
Resolving Conflicts: I open the conflicting files and manually resolve conflicts by selecting the appropriate changes. After resolving conflicts, I add the resolved files and continue the merge.
# Open the file and resolve conflicts
git add file.txt
git commit
Using Tools: For complex conflicts, I use merge tools like KDiff3
or VSCode
‘s built-in merge tool to visualize differences and resolve conflicts more easily.
Example: In a previous project, we used Git for version control with a branching strategy similar to Git Flow. This helped us manage multiple features, releases, and hotfixes efficiently. During a merge, we encountered conflicts in several files. We resolved these conflicts by discussing the changes with team members, using merge tools to visualize differences, and thoroughly testing the merged code to ensure stability.
Evaluating Responses: Candidates should demonstrate a clear understanding of Git, including common commands and branching strategies. Look for experience with resolving conflicts and using tools to manage branches effectively. Assess their ability to explain their approach to version control and collaboration within a team.
10. How do you stay current with new technologies and programming languages? Can you give an example of a recent technology you’ve learned and how you applied it?
Question Explanation: Staying updated with new technologies is crucial for software engineers. This software engineer interview question assesses the candidate’s commitment to continuous learning and their ability to apply new knowledge in practical scenarios.
Expected Answer: To stay current with new technologies and programming languages, I follow a multi-faceted approach:
- Reading and Research: I regularly read technology blogs, articles, and research papers. Websites like Medium, Hacker News, and TechCrunch are valuable sources of information. I also follow thought leaders and industry experts on social media platforms like Twitter and LinkedIn.
- Online Courses and Tutorials: I take online courses on platforms like Coursera, Udemy, and edX to learn new technologies and programming languages. Interactive tutorials on websites like Codecademy and freeCodeCamp are also helpful.
- Community Engagement: Participating in developer communities, attending meetups, and joining online forums like Stack Overflow, Reddit, and GitHub help me learn from peers and stay updated with industry trends.
- Hands-On Projects: I believe in learning by doing. I build side projects, contribute to open-source projects, and participate in hackathons to apply new technologies in practical scenarios.
Example: Recently, I learned about Docker, a tool that allows for containerization of applications. Here’s how I applied it:
- Learning Docker: I started with online tutorials and courses to understand the basics of Docker, including container creation, management, and orchestration. I also read the official Docker documentation and followed along with examples.
- Applying Docker: I decided to containerize a web application I was working on. The application had multiple components, including a frontend, backend, and database. Using Docker, I created Dockerfiles for each component and defined a Docker Compose file to manage the multi-container application.
# Dockerfile for backend
FROM python:3.8-slim-buster
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
# docker-compose.yml
version: '3'
services:
db:
image: postgres
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
POSTGRES_DB: mydb
backend:
build: ./backend
ports:
- "5000:5000"
depends_on:
- db
frontend:
build: ./frontend
ports:
- "3000:3000"
- Benefits: Containerizing the application made it easier to manage dependencies, ensure consistency across development and production environments, and streamline the deployment process. I documented the setup process and shared it with my team, improving our overall development workflow.
Evaluating Responses: Candidates should show a proactive approach to learning and staying current with new technologies. Look for specific examples of how they have learned and applied new technologies in their projects. Assess their ability to articulate the benefits and challenges of adopting new tools and languages.
11. Explain the difference between relational and non-relational databases. When would you choose one over the other?
Question Explanation: Understanding the difference between relational and non-relational databases is fundamental for designing data storage solutions. This question assesses the candidate’s knowledge of database types and their ability to choose the appropriate database for different use cases.
Expected Answer: Relational and non-relational databases differ in their structure, use cases, and data management approaches.
- Relational Databases (RDBMS):
- Structure: Relational databases use tables to store data, with rows representing records and columns representing attributes. Tables can be linked using foreign keys, enabling complex queries and data integrity.
- Schema: They have a predefined schema that enforces data types and relationships between tables.
- Examples: MySQL, PostgreSQL, Oracle, SQL Server.
- Use Cases: Relational databases are suitable for applications requiring complex queries, transactions, and data integrity. Examples include financial systems, enterprise applications, and content management systems.
- Non-Relational Databases (NoSQL):
- Structure: Non-relational databases can store data in various formats, such as key-value pairs, documents, graphs, or wide-column stores. They are more flexible in terms of data structure.
- Schema: They have a dynamic schema, allowing for more flexible and scalable data models.
- Examples: MongoDB (document), Redis (key-value), Cassandra (wide-column), Neo4j (graph).
- Use Cases: Non-relational databases are suitable for applications with large volumes of unstructured or semi-structured data, real-time analytics, and flexible data models. Examples include social networks, big data applications, and IoT systems.
Choosing Between Them:
- Data Structure and Integrity: If the application requires complex relationships and data integrity, a relational database is preferred. For example, in an e-commerce platform where transactions and customer data integrity are crucial, an RDBMS like PostgreSQL would be suitable.
- Scalability and Flexibility: For applications with dynamic and scalable data models, a non-relational database is preferred. For example, in a real-time analytics system for monitoring IoT devices, a NoSQL database like MongoDB or Cassandra would be more appropriate due to their horizontal scalability and flexible schemas.
- Performance: For high read/write throughput and low latency, non-relational databases are often better. Redis, a key-value store, is ideal for caching and session management due to its in-memory data storage.
Example: In a project where we developed a social media platform, we used both relational and non-relational databases. User authentication and profile data were stored in PostgreSQL due to the need for data integrity and complex queries. For storing user-generated content like posts and comments, we used MongoDB because of its flexible schema and scalability. This hybrid approach allowed us to leverage the strengths of both database types.
Evaluating Responses: Candidates should demonstrate a clear understanding of the differences between relational and non-relational databases, including their structures, use cases, and benefits. Look for practical examples of when they have used each type of database and their rationale for choosing one over the other. Assess their ability to explain how different databases can be integrated within a single application to meet specific requirements.
12. What is your experience with cloud platforms such as AWS, Azure, or Google Cloud? Can you describe a project where you utilized cloud services?
Question Explanation: Cloud platforms provide a wide range of services for building, deploying, and managing applications. This software engineer interview question assesses the candidate’s experience with cloud platforms and their ability to leverage cloud services in real-world projects.
Expected Answer: I have extensive experience with cloud platforms, including AWS, Azure, and Google Cloud. Each platform offers a variety of services for computing, storage, databases, networking, and more. Here’s an overview of my experience and a specific project where I utilized cloud services:
- AWS (Amazon Web Services):
- Services Used: EC2 for compute, S3 for storage, RDS for relational databases, Lambda for serverless computing, and CloudFormation for infrastructure as code.
- Example: In a recent project, I used AWS to build a scalable web application. We utilized EC2 instances for the application servers, S3 for storing user-uploaded files, and RDS (PostgreSQL) for the database. AWS Lambda was used for processing background tasks, and CloudFormation was used to define and manage the infrastructure.
- Azure:
- Services Used: Azure App Services for web hosting, Azure SQL Database for relational data, Azure Functions for serverless computing, and Azure DevOps for CI/CD pipelines.
- Example: In a previous job, we migrated an on-premises application to Azure. We used Azure App Services to host the web application, Azure SQL Database for data storage, and Azure Functions to handle event-driven processing. Azure DevOps was used to automate the deployment pipeline, ensuring quick and reliable releases.
- Google Cloud:
- Services Used: Compute Engine for virtual machines, Cloud Storage for object storage, Firestore for NoSQL databases, and Cloud Run for deploying containerized applications.
- Example: In a side project, I built a microservices-based application using Google Cloud. We used Compute Engine to run the services, Cloud Storage to store media files, and Firestore as the database. Cloud Run was used to deploy and manage the containerized services, providing a scalable and efficient solution.
Project Example: In a recent project, we developed a serverless data processing pipeline on AWS. The goal was to process and analyze large volumes of data in real-time. Here’s how we utilized AWS services:
- Data Ingestion: We used AWS Kinesis Data Streams to ingest data from various sources. Kinesis allowed us to process data streams in real-time with low latency.
- Data Processing: AWS Lambda functions were triggered by Kinesis events to process the incoming data. Lambda’s serverless architecture enabled us to scale the processing automatically based on the volume of data.
- Data Storage: Processed data was stored in Amazon S3 for long-term storage and Amazon Redshift for analytics. S3 provided a cost-effective and durable storage solution, while Redshift enabled us to run complex queries and generate insights from the data.
- Infrastructure Management: We used AWS CloudFormation to define and deploy the entire infrastructure as code. This approach ensured consistency and made it easy to replicate the environment in different regions.
Evaluating Responses: Candidates should demonstrate familiarity with major cloud platforms and their services. Look for specific examples of how they have used cloud services in projects, focusing on the architecture, tools, and outcomes. Assess their ability to explain the benefits of using cloud platforms, such as scalability, reliability, and cost-efficiency. Evaluate their understanding of best practices in cloud computing, including security, automation, and monitoring.
13. How do you handle security concerns in your software development process? Provide specific measures you take to ensure security.
Question Explanation: Security is a critical aspect of software development. This question evaluates the candidate’s understanding of security best practices and their ability to implement measures to protect applications and data.
Expected Answer: Handling security concerns in software development involves adopting best practices and implementing specific measures throughout the development lifecycle. Here are the steps I take to ensure security:
- Secure Coding Practices:
- Input Validation: Validate and sanitize all user inputs to prevent injection attacks, such as SQL injection and cross-site scripting (XSS).
- Output Encoding: Encode data before rendering it to the user to prevent XSS attacks.
- Authentication and Authorization: Implement strong authentication mechanisms, such as multi-factor authentication (MFA), and enforce least privilege access control.
- Encryption:
- Data Encryption: Use encryption to protect sensitive data at rest and in transit. For example, use HTTPS to encrypt data transmitted over the network and AES for encrypting data stored in databases.
- Password Storage: Store passwords using strong hashing algorithms, such as bcrypt or Argon2, with a unique salt for each password.
- Dependency Management:
- Vulnerability Scanning: Regularly scan third-party libraries and dependencies for known vulnerabilities using tools like Snyk or OWASP Dependency-Check.
- Updating Dependencies: Keep dependencies up-to-date and apply security patches promptly.
- Security Testing:
- Static Code Analysis: Use static analysis tools, such as SonarQube or ESLint, to identify potential security issues in the code.
- Penetration Testing: Conduct regular penetration testing to identify and address security vulnerabilities from an attacker’s perspective.
- Automated Security Tests: Integrate security tests into the CI/CD pipeline to catch security issues early in the development process.
- Monitoring and Incident Response:
- Logging and Monitoring: Implement comprehensive logging and monitoring to detect and respond to security incidents in real-time. Use tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk.
- Incident Response Plan: Develop and maintain an incident response plan to handle security breaches effectively and minimize damage.
Example: In a recent project, I was responsible for developing a web application that handled sensitive user data. Here are the specific security measures I implemented:
- Input Validation: I used a validation library to ensure all user inputs were properly validated and sanitized.
- Encryption: I enforced HTTPS using SSL/TLS certificates and encrypted sensitive data stored in the database with AES-256 encryption.
- Dependency Management: I regularly scanned dependencies with Snyk and promptly updated any vulnerable packages.
- Security Testing: I integrated static code analysis into our CI/CD pipeline and conducted quarterly penetration tests to identify and address security vulnerabilities.
- Logging and Monitoring: I set up comprehensive logging using the ELK Stack and created alerts for suspicious activities, enabling rapid response to potential security incidents.
Evaluating Responses: Candidates should demonstrate a solid understanding of security best practices and specific measures to protect applications and data. Look for practical examples of how they have implemented these measures in their projects. Assess their ability to explain the importance of security at different stages of development and their familiarity with security tools and techniques.
14. Describe a challenging bug you encountered and how you resolved it. What did you learn from the experience?
Question Explanation: Debugging is a crucial skill for software engineers. This software engineer interview question assesses the candidate’s problem-solving abilities, their approach to diagnosing and resolving complex issues, and their ability to learn from challenging experiences.
Expected Answer: One of the most challenging bugs I encountered was in a distributed system where intermittent data loss occurred during peak usage. Here’s how I approached and resolved the issue:
- Symptom Identification:
- Users reported missing data in certain reports generated by our system. The issue was not consistently reproducible and only occurred under high load conditions.
- Investigation:
- I started by examining the logs from different components of the system to identify any anomalies or error messages. This revealed that some data processing jobs were timing out during peak load.
- I also reviewed the code for data ingestion and processing to identify potential bottlenecks or race conditions that could lead to data loss.
- Reproduction and Isolation:
- To reproduce the issue, I set up a load testing environment that simulated peak usage scenarios. By gradually increasing the load, I was able to consistently trigger the data loss issue.
- Using profiling tools, I identified that the data processing component was experiencing thread contention, causing timeouts and data loss.
- Resolution:
- I refactored the data processing code to improve concurrency handling. Specifically, I optimized the use of locks to minimize contention and implemented a more efficient batching mechanism to process data in chunks.
- I also increased the timeout settings for data processing jobs to allow more time for completion during peak loads.
- Testing and Deployment:
- After making the changes, I conducted extensive load testing to ensure the issue was resolved and the system could handle peak loads without data loss.
- The fix was then deployed to production, and I continued to monitor the system closely to confirm the issue was resolved.
Learnings:
- Importance of Detailed Logging: Comprehensive logging was crucial in diagnosing the issue and identifying the root cause.
- Effective Use of Profiling Tools: Profiling tools helped pinpoint performance bottlenecks and thread contention issues.
- Concurrency Management: Proper management of concurrency is essential in distributed systems to prevent data loss and ensure reliability.
- Load Testing: Simulating real-world usage scenarios through load testing is vital for identifying and resolving issues that only occur under stress.
Evaluating Responses: Candidates should demonstrate a methodical approach to diagnosing and resolving complex bugs. Look for their ability to identify symptoms, investigate the root cause, implement a fix, and validate the solution. Assess their problem-solving skills, attention to detail, and ability to learn from challenging experiences.
15. What is your approach to code reviews, both when reviewing others’ code and when your code is being reviewed?
Question Explanation: Code reviews are essential for maintaining code quality and fostering team collaboration. This question evaluates the candidate’s understanding of the code review process, their ability to provide constructive feedback, and their openness to receiving feedback.
Expected Answer: Code reviews are an integral part of the development process that help ensure code quality, identify potential issues, and facilitate knowledge sharing. Here’s my approach to code reviews:
- Reviewing Others’ Code:
- Understand the Context: Before reviewing the code, I take the time to understand the purpose and context of the changes. I read through the related documentation, requirements, and any comments provided by the author.
- Review for Functionality and Quality: I check if the code meets the requirements and functions as intended. I also evaluate the code for readability, maintainability, and adherence to coding standards and best practices.
- Provide Constructive Feedback: I provide specific, actionable, and constructive feedback. I focus on the code and avoid personal comments. I highlight both the strengths and areas for improvement.
- Encourage Discussion: I encourage open discussion and collaboration to address any concerns or suggestions. This helps in reaching a consensus and improving the overall code quality.
- When My Code is Being Reviewed:
- Provide Context: I ensure that my code changes are well-documented, with clear commit messages and comments explaining the purpose and functionality. This helps reviewers understand the context and rationale behind the changes.
- Be Open to Feedback: I approach code reviews with an open mind and a willingness to learn. I appreciate constructive feedback and use it as an opportunity to improve my skills and the quality of my code.
- Clarify and Discuss: If I receive feedback that I don’t fully understand or agree with, I seek clarification and engage in a constructive discussion. This helps in gaining different perspectives and finding the best solutions.
- Iterate and Improve: I promptly address the feedback by making necessary changes and improvements to the code. I also update the reviewers on the changes and seek final approval.
Evaluating Responses: Candidates should demonstrate a thoughtful and constructive approach to code reviews. Look for their ability to provide clear and actionable feedback, their openness to receiving feedback, and their commitment to maintaining code quality. Assess their communication skills and their ability to collaborate effectively with team members during the review process.
Conclusion
These software engineer interview questions are crafted to evaluate a candidate’s technical acumen, problem-solving skills, and ability to apply best practices in software development. By focusing on these areas, interviewers can identify individuals who are not only proficient in coding but also capable of maintaining high standards of quality, performance, and collaboration in their work.