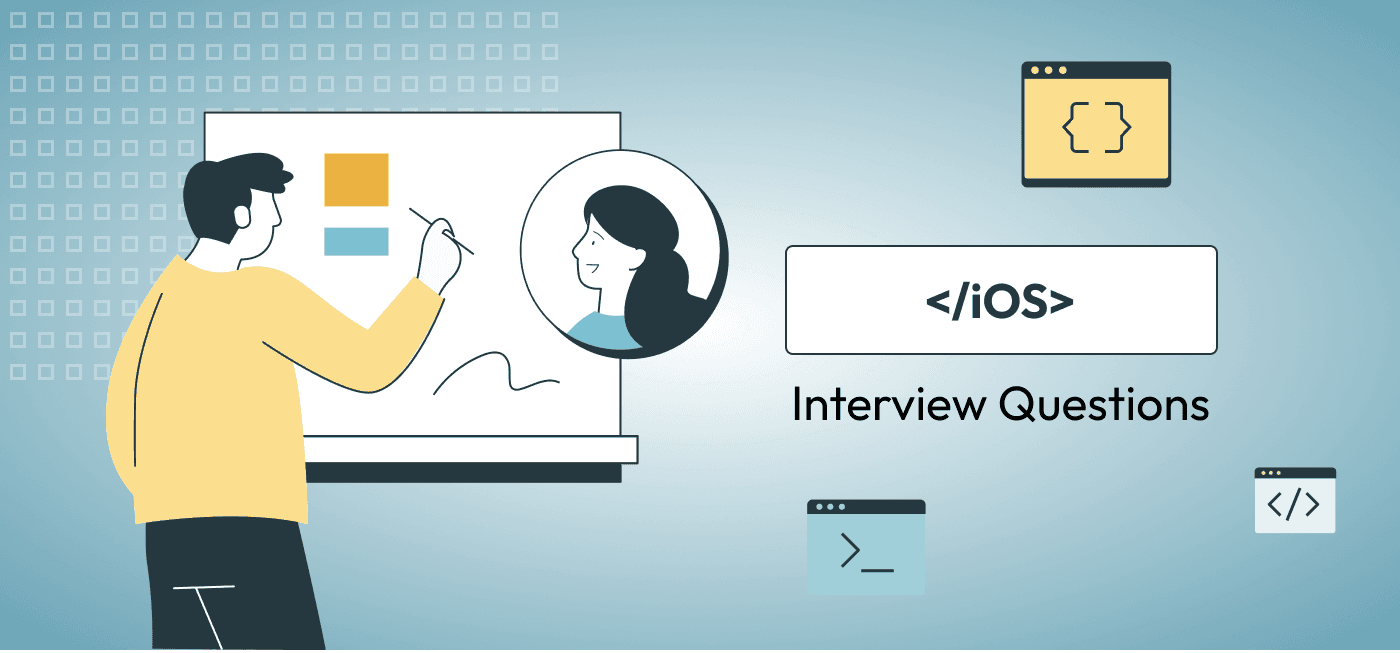
Hiring + recruiting | Blog Post
15 iOS Interview Questions for Hiring iOS Engineers
Todd Adams
Share this post
In today’s mobile-first world, iOS development has become an essential skill for building sleek, user-friendly applications that meet high performance and design standards. When hiring iOS engineers, it’s important to assess not only their knowledge of Swift and Objective-C but also their experience with core frameworks, design patterns, and Apple’s ecosystem. Below is a list of 15 insightful iOS interview questions aimed at helping you evaluate a candidate’s depth of knowledge, problem-solving abilities, and hands-on experience in iOS development.
iOS Interview Questions
1. What is the difference between frame
, bounds
, and center
in iOS layout?
Question Explanation:
Understanding view layout properties like frame
, bounds
, and center
is crucial for building and managing responsive UIs. Each property plays a distinct role in determining a view’s position and size on the screen, and confusion between them can lead to layout issues. This iOS interview question will assist with further understanding.
Expected Answer:
frame
defines the view’s origin and size relative to its superview’s coordinate system. It is expressed in terms of the superview’s coordinate space.
Example:
let frame = view.frame
print("Frame: \(frame)") // Outputs: (x: 50, y: 100, width: 200, height: 300)
bounds
refers to the view’s own internal coordinate system. It represents the origin and size of the view, where the origin is typically (0, 0), but it can change for transformations like scrolling or zooming.
Example:
let bounds = view.bounds
print("Bounds: \(bounds)") // Outputs: (x: 0, y: 0, width: 200, height: 300)
center
indicates the view’s position relative to its superview’s coordinate space. It represents the center point of the view.
Example:
let center = view.center
print("Center: \(center)") // Outputs: (x: 150, y: 250)
Evaluating Responses:
A good answer should clearly distinguish between the three properties and provide examples of their usage. The candidate should demonstrate an understanding of how frame
is based on the superview’s coordinate system, while bounds
is internal to the view itself. They should also explain how adjusting center
moves the view while keeping its size unchanged.
2. How does memory management work in iOS, and what is ARC (Automatic Reference Counting)?
Question Explanation:
Memory management ensures that applications do not leak memory and use resources efficiently. Developers should be familiar with ARC, including this iOS interview question focused on it, as improper memory management can lead to performance issues or app crashes.
Expected Answer:
ARC is Apple’s memory management system in iOS and macOS, which automatically tracks and manages the reference count of objects. Each time you create a reference to an object, ARC increases its reference count. When a reference is no longer needed, ARC decreases the count, and if it reaches zero, the object is deallocated.
- Strong references increase the reference count, and weak references do not affect it. A weak reference is used to avoid retain cycles, which occur when two objects hold strong references to each other, causing neither to be deallocated.
- ARC also uses unowned references, which are similar to weak references but do not become
nil
when the referenced object is deallocated. This is useful when the referenced object is guaranteed to outlive the reference.
Example of a retain cycle:
class A {
var b: B?
}
class B {
var a: A?
}
let a = A()
let b = B()
a.b = b
b.a = a // Retain cycle: both objects hold strong references to each other.
Evaluating Responses:
A strong candidate should explain ARC’s basic functionality and differentiate between strong, weak, and unowned references. They should also discuss retain cycles and how to prevent them using weak references, possibly with an example of a retain cycle in code.
3. Can you explain the difference between synchronous and asynchronous tasks in iOS?
Question Explanation:
Concurrency is a critical concept for improving app responsiveness by moving long-running tasks to background threads. Synchronous vs. asynchronous execution is fundamental to understanding concurrency.
Expected Answer:
- Synchronous tasks are blocking tasks that must complete before the next line of code can execute. The current thread is paused until the task is finished, which can lead to UI freezing if used on the main thread.
Example of a synchronous task:
print("Start")
doSomeTaskSynchronously() // Blocks the current thread
print("End") // Executes after the synchronous task completes
- Asynchronous tasks are non-blocking tasks that allow other operations to continue executing while the task runs in the background. When the asynchronous task completes, a callback or completion handler is triggered.
Example of an asynchronous task:
print("Start")
doSomeTaskAsynchronously {
print("Task completed")
}
print("End") // Executes immediately without waiting for the asynchronous task
In iOS, you can manage asynchronous tasks using GCD (Grand Central Dispatch) or NSOperationQueue. Asynchronous execution ensures the UI remains responsive while performing long-running tasks such as network calls or database operations.
Evaluating Responses:
Look for an understanding of how synchronous execution can block the main thread and degrade user experience, while asynchronous execution improves app responsiveness. A strong candidate should also demonstrate knowledge of how to manage async tasks using GCD or completion handlers, and they should emphasize avoiding long-running operations on the main thread.
4. How would you implement user authentication using Apple’s Authentication Services (Sign in with Apple)?
Question Explanation:
Sign in with Apple is a privacy-focused authentication method that integrates tightly with iOS applications. Knowledge of implementing secure authentication, including this iOS interview question on it, is essential for developing apps that need user login functionality.
Expected Answer:
Apple provides AuthenticationServices to implement “Sign in with Apple,” which offers users the ability to sign in without revealing personal information. It provides a secure, streamlined experience with support for two-factor authentication.
Steps to implement:
- Import AuthenticationServices and create an
ASAuthorizationAppleIDButton
to provide the sign-in option in the app.
Example:
let appleIDButton = ASAuthorizationAppleIDButton()
appleIDButton.addTarget(self, action: #selector(handleAuthorizationAppleIDButtonPress), for: .touchUpInside)
- Handle the sign-in request using
ASAuthorizationController
to manage the authentication flow.
Example:
@objc func handleAuthorizationAppleIDButtonPress() {
let request = ASAuthorizationAppleIDProvider().createRequest()
request.requestedScopes = [.fullName, .email]
let authorizationController = ASAuthorizationController(authorizationRequests: [request])
authorizationController.delegate = self
authorizationController.performRequests()
}
- Handle the response in the
ASAuthorizationControllerDelegate
to retrieve the user’s credentials securely.
Evaluating Responses:
Candidates should demonstrate an understanding of how to use AuthenticationServices to implement “Sign in with Apple.” Look for knowledge of proper error handling, managing authentication tokens, and integrating this feature securely with other backend systems. A good answer should cover privacy concerns, like how Apple minimizes the personal data shared with apps during authentication.
5. Describe how UITableView
works. How would you optimize the performance of a table with many rows?
Question Explanation:
UITableView
is one of the most widely used UI components in iOS development. Understanding how it works and knowing how to optimize it for performance is essential, especially when handling large data sets. This iOS interview question will assist in further knowledge.
Expected Answer:
- How
UITableView
works:UITableView
is used to display a list of items in a single-column, vertically scrolling interface. It relies on reusable cells to optimize performance. Instead of creating a new cell for each row,UITableView
uses thedequeueReusableCell(withIdentifier:)
method to reuse cells that are no longer visible on the screen. Cells are populated with data via a data source, which provides the content for each row through thetableView(_:cellForRowAt:)
method.
Example:
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = dataArray[indexPath.row]
return cell
}
- Optimizing performance: To optimize performance for tables with many rows, consider these techniques:
- Cell Reuse: Ensure you use cell reuse identifiers correctly to minimize memory consumption.
- Lazy Loading of Images: When displaying images in a table, use lazy loading techniques or asynchronous image loading to avoid blocking the main thread.
- Batch Data Loading: Load data in chunks (pagination) instead of loading all the data at once.
- Cell Height Caching: If dynamic heights are used, cache cell heights to avoid recalculating them each time a row is displayed
- Avoid Complex Layouts: Use simple view hierarchies in cells, as complex layouts can degrade scrolling performance.
Evaluating Responses:
A strong candidate should explain the basics of UITableView
, including how to properly use reusable cells. They should also discuss practical optimizations such as lazy image loading, cell height caching, and efficient data loading techniques like pagination. The candidate should show an understanding of how to prevent UI freezes and enhance smooth scrolling performance.
6. What are some key differences between GCD
(Grand Central Dispatch) and NSOperationQueue
?
Question Explanation:
Concurrency is critical in modern iOS applications to ensure smooth user experiences. Both GCD and NSOperationQueue are used to manage concurrent tasks, but they differ in flexibility, ease of use, and advanced features.
Expected Answer:
- Grand Central Dispatch (GCD): GCD is a low-level C-based API for managing concurrent tasks. It offers a lightweight interface for submitting blocks of code to different dispatch queues. You can use GCD for both synchronous and asynchronous tasks, and it is often used when you need maximum performance with minimal configuration.
Example of GCD usage:
DispatchQueue.global(qos: .background).async {
// Background task
DispatchQueue.main.async {
// Update UI on the main thread
}
}
- NSOperationQueue:
NSOperationQueue
is a higher-level abstraction built on top of GCD. It usesNSOperation
objects to represent tasks, offering more control over dependencies, priorities, and cancellations.NSOperationQueue
is useful when you need to manage complex task relationships or when tasks depend on the completion of other tasks.
Example of NSOperationQueue usage:
let operationQueue = OperationQueue()
let operation = BlockOperation {
// Background task
}
operationQueue.addOperation(operation)
- Key Differences:
- Ease of Use: GCD is simpler for small, straightforward tasks.
NSOperationQueue
provides more advanced control. - Task Dependencies:
NSOperationQueue
allows you to define dependencies between operations, which is not as easy in GCD. - Cancellation and Suspension:
NSOperationQueue
allows you to cancel, suspend, or prioritize tasks, while GCD lacks direct mechanisms for task control. - Customization:
NSOperationQueue
can be customized with properties such as maximum concurrent operations and operation priorities, while GCD is less customizable.
- Ease of Use: GCD is simpler for small, straightforward tasks.
Evaluating Responses:
A strong candidate should explain the core concepts behind GCD and NSOperationQueue
and outline scenarios where each is preferable. They should emphasize that GCD is better for lightweight, simple concurrency needs, while NSOperationQueue
is more suited for complex task management with dependencies and cancellation.
7. How do you persist data in iOS applications? Discuss options like Core Data, UserDefaults, and SQLite.
Question Explanation:
Persisting data locally is a common requirement in iOS apps, whether it’s saving user preferences, offline data, or managing large datasets. Candidates need to know the different persistence options and when to use each one.
Expected Answer:
- UserDefaults: This is a simple key-value store used for storing small amounts of user data, like preferences and settings. It is not meant for large or complex data structures. Data stored here persists across app launches.
Example:
UserDefaults.standard.set("username123", forKey: "username")
let username = UserDefaults.standard.string(forKey: "username")
- Core Data: Core Data is an object graph and persistence framework, ideal for managing complex data models and relationships. It supports undo/redo functionality, data migration, and offers tools for querying data efficiently. Core Data abstracts the underlying storage mechanism, which can be SQLite, binary, or in-memory.
Example:
// Creating a new entity in Core Data
let entity = NSEntityDescription.insertNewObject(forEntityName: "Person", into: managedObjectContext)
entity.setValue("John", forKey: "name")
- SQLite: SQLite is a lightweight relational database that offers more control over raw SQL queries. It’s ideal for developers who want to work with relational data but need low-level access to the database. While Core Data abstracts much of the complexity, SQLite gives you direct control over SQL statements.
Example:
var db: OpaquePointer?
sqlite3_open(filePath, &db)
let query = "SELECT * FROM Users"
- File Storage: For saving files such as images, documents, or videos, you can use the File System APIs in iOS. Files can be saved to directories like
Documents
orCaches
.
Evaluating Responses:
A strong candidate should demonstrate a clear understanding of each storage method, including its strengths and limitations. They should be able to choose the correct persistence option based on the app’s requirements (e.g., using UserDefaults for small settings, Core Data for complex object models, and SQLite for relational data). Practical knowledge of when to use each option is key.
8. What is the Model-View-Controller (MVC) pattern, and how is it used in iOS development?
Question Explanation:
MVC is a foundational design pattern in iOS development that separates concerns into three distinct layers: Model, View, and Controller. Candidates should be familiar with how it’s used to structure iOS applications and its potential pitfalls, including this iOS interview question focused on it.
Expected Answer:
- Model: The model represents the data and business logic of the application. It is responsible for managing data, whether from a local database or a network source, and for informing the controller when data changes.
Example:
struct User {
var name: String
var age: Int
}
- View: The view is responsible for displaying the data to the user. It is typically a subclass of
UIView
, and it knows how to present the data but not how to fetch or manipulate it.
Example:
class UserView: UIView {
var nameLabel: UILabel
// Responsible for displaying user data
}
- Controller: The controller (often a
UIViewController
) acts as an intermediary between the view and model. It handles user input, updates the model, and refreshes the view when the model changes. The controller often contains the business logic, which can lead to it becoming bloated (known as the “Massive View Controller” problem).
Example:
class UserController: UIViewController {
var user: User?
func viewDidLoad() {
user = fetchUserData()
updateView()
}
func updateView() {
userView.nameLabel.text = user?.name
}
}
Evaluating Responses:
The candidate should be able to explain the roles of the Model, View, and Controller in the MVC pattern. They should be aware of potential pitfalls, such as the tendency for controllers to become bloated with business logic. A strong response might mention alternatives like MVVM (Model-View-ViewModel) or MVP (Model-View-Presenter) to address the shortcomings of MVC in complex apps.
9. Explain the lifecycle of a UIViewController
.
Question Explanation:
The lifecycle of a UIViewController
is fundamental to managing view loading, updating, and cleaning up resources in an iOS application. Understanding each stage of the lifecycle allows developers to control how and when views and data are managed and updated, including this iOS interview question focused on it.
Expected Answer:
The UIViewController
lifecycle consists of several key methods that are called as the view controller’s views are loaded, presented, and destroyed. The most important lifecycle methods are:
viewDidLoad
: Called after the view controller’s view is loaded into memory. This method is used for initial setup such as initializing data, setting up UI components, and configuring the view. This method is only called once when the view is first loaded.
Example:
override func viewDidLoad() {
super.viewDidLoad()
// Initial setup
print("View did load")
}
viewWillAppear(_:)
: Called right before the view is about to appear on the screen. This is the perfect place to make final adjustments to the UI, such as updating labels or refreshing data. It gets called every time the view appears, including after being pushed or popped from the navigation stack.
Example:
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
print("View will appear")
}
viewDidAppear(_:)
: Called right after the view has appeared on the screen. It’s often used to trigger animations or start certain tasks that should only begin after the view is fully visible to the user.
Example:
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
print("View did appear")
}
viewWillDisappear(_:)
: Called just before the view is about to be removed from the screen. This method is used to stop ongoing tasks, such as saving state, pausing timers, or cancelling network requests.
Example:
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
print("View will disappear")
}
viewDidDisappear(_:)
: Called immediately after the view is removed from the screen. This method is typically used for cleanup.
Example:
override func viewDidDisappear(_ animated: Bool) {
super.viewDidDisappear(animated)
print("View did disappear")
}
Evaluating Responses:
A good answer should clearly describe the major lifecycle methods (viewDidLoad
, viewWillAppear
, viewDidAppear
, viewWillDisappear
, viewDidDisappear
) and explain when each is called. The candidate should also provide practical use cases for each method, like initializing data in viewDidLoad
or stopping tasks in viewWillDisappear
. Advanced candidates might mention other lifecycle methods like viewWillLayoutSubviews
or viewDidLayoutSubviews
for more precise layout adjustments.
10. How would you handle push notifications in an iOS app?
Question Explanation:
Push notifications are a key feature in modern apps for engaging users, alerting them to updates, and encouraging interaction. Handling notifications properly requires knowledge of both server-side configuration and client-side implementation in iOS. This iOS interview question will assist in further knowledge.
Expected Answer:
To handle push notifications in an iOS app, you need to implement both server-side and client-side components.
- Registering for Notifications: The first step is to request permission from the user to receive notifications. This is done using the UserNotifications framework.
Example:
import UserNotifications
UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { granted, error in
if granted {
DispatchQueue.main.async {
UIApplication.shared.registerForRemoteNotifications()
}
}
}
- Receiving the Device Token: Once the user grants permission, the app will receive a unique device token, which needs to be sent to your server to register the device for notifications.
Example:
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
// Send device token to server
let token = deviceToken.map { String(format: "%02.2hhx", $0) }.joined()
print("Device token: \(token)")
}
- Handling Incoming Notifications: When a push notification is received, you can handle it by implementing the
UNUserNotificationCenterDelegate
methods.
Example:
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
let userInfo = response.notification.request.content.userInfo
// Handle the notification
completionHandler()
}
- Server-Side Setup: On the server side, you need to send push notifications via Apple Push Notification Service (APNs). The server will use the device token to send notifications to specific devices.
Example (using a server API):
{
"aps": {
"alert": "New message received!",
"sound": "default"
}
}
Evaluating Responses:
A good answer should mention the steps to request notification permissions, handle device tokens, and process incoming notifications. The candidate should also discuss how to send notifications via APNs and might mention background tasks, silent notifications, or deep linking as more advanced concepts. They should show an understanding of user experience considerations, such as explaining the app’s need for notifications to the user.
11. What is the difference between a strong reference and a weak reference in Swift?
Question Explanation:
Memory management is a critical aspect of iOS development, and avoiding memory leaks is crucial for building performant apps. Understanding the difference between strong and weak references helps prevent retain cycles, especially when working with closures or delegate patterns, including this iOS interview question focused on it.
Expected Answer:
- Strong Reference: By default, every property or variable in Swift holds a strong reference to the object it points to. A strong reference increases the reference count of an object, ensuring that it stays in memory as long as at least one strong reference exists. This can sometimes lead to retain cycles, where two objects hold strong references to each other, preventing either from being deallocated.
Example:
class A {
var b: B?
}
class B {
var a: A?
}
let a = A()
let b = B()
a.b = b
b.a = a // Retain cycle: A and B hold strong references to each other.
- Weak Reference: A weak reference does not increase the reference count of the object. Weak references are automatically set to
nil
when the object they point to is deallocated, which helps prevent retain cycles.
Example:
class A {
weak var b: B?
}
In this example, the weak reference ensures that A
does not hold a strong reference to B
, breaking the retain cycle.
- Unowned Reference: An unowned reference is similar to a weak reference in that it does not increase the reference count. However, unlike weak references, unowned references are not set to
nil
when the object is deallocated. Use an unowned reference when the referenced object is guaranteed to exist for the lifetime of the reference.
Example:
class A {
unowned var b: B?
}
Evaluating Responses:
A strong candidate should clearly explain the differences between strong, weak, and unowned references, including how they affect an object’s lifecycle and memory management. The candidate should give an example of a retain cycle and show how using weak references can prevent it. Bonus points if the candidate discusses when to use weak vs. unowned references.
12. Describe how Swift’s Result
type can be used for error handling.
Question Explanation:
Swift’s Result
type is a powerful tool for simplifying error handling, especially in asynchronous operations. Knowing how to use Result
demonstrates that a candidate is familiar with modern Swift patterns for safe and expressive error handling. This iOS interview question will assist with further knowledge.
Expected Answer:
Swift’s Result
type is an enum that represents either a success or failure of an operation. It is used to model the outcome of functions that can either return a value or throw an error. The Result
type has two cases:
.success(Value)
: Indicates that the operation was successful and holds the returned value..failure(Error)
: Indicates that the operation failed and holds an error.
The Result
type helps avoid traditional error throwing and catching, making it especially useful in closures and asynchronous tasks.
Example of Result
usage:
enum NetworkError: Error {
case invalidURL
case noData
}
func fetchData(from url: String, completion: (Result<Data, NetworkError>) -> Void) {
guard let url = URL(string: url) else {
completion(.failure(.invalidURL))
return
}
// Simulate a network call
completion(.success(Data()))
}
Using Result
in a function:
fetchData(from: "https://example.com") { result in
switch result {
case .success(let data):
print("Data received: \(data)")
case .failure(let error
13. How would you implement offline data storage and syncing in an iOS app?
Question Explanation:
In many apps, users expect access to data even when they are offline. Implementing offline data storage and sync mechanisms is essential in ensuring that users can interact with the app without connectivity. This feature requires knowledge of local data storage and strategies for syncing data with a remote server. This iOS interview question will assist with further knowledge.
Expected Answer:
To implement offline data storage and syncing in an iOS app, there are several key components:
- Local Data Storage: Use Core Data or SQLite to store data locally on the device. Core Data is preferred for complex object graphs, while SQLite is a good option for direct SQL queries. You can also use simple storage methods like Realm or UserDefaults for less complex data.
Example:
// Storing data in Core Data
let entity = NSEntityDescription.insertNewObject(forEntityName: "Note", into: managedObjectContext)
entity.setValue("Note Title", forKey: "title")
entity.setValue("Note Content", forKey: "content")
- Background Syncing: Use NSURLSession to synchronize data with a remote server in the background. Implement background tasks to ensure data syncing continues when the app is not active.
Example:
let backgroundTask = UIApplication.shared.beginBackgroundTask { [weak self] in
self?.endBackgroundTask()
}
let session = URLSession(configuration: .background(withIdentifier: "com.example.sync"))
- Conflict Resolution: Handle data conflicts by implementing a merging strategy. You can use techniques such as:
- Last-Write-Wins (LWW), where the most recent change is accepted.
- Versioning, where changes are versioned and merged.
- User Interventions, where the user manually resolves conflicts.
- Sync Triggers: Sync data at specific times, like when the app moves from background to foreground, periodically in the background, or manually by the user (e.g., a “Pull to Refresh” gesture).
- Handling Sync Failures: Implement retry logic with exponential backoff in case of network failures, ensuring the app can gracefully recover from intermittent connectivity issues.
Evaluating Responses:
A good answer will include details about choosing an appropriate local storage method (Core Data, SQLite), handling background tasks for syncing, and conflict resolution strategies. Candidates should also discuss how to handle network failures gracefully and ensure data consistency between the local storage and remote server.
14. What are some techniques to debug and improve the performance of an iOS app?
Question Explanation:
App performance is a key factor for user experience. Debugging and optimizing an iOS app ensures smooth interactions, fast load times, and resource efficiency. Understanding how to use tools like Instruments and best practices for optimizing code is essential for any iOS developer. This iOS interview question will assist with further knowledge.
Expected Answer:
Several techniques can be used to debug and improve the performance of an iOS app:
- Using Instruments: Instruments is a powerful tool provided by Xcode for profiling app performance. Key Instruments include:
- Time Profiler: Used to find bottlenecks in CPU usage. It identifies which methods are taking up the most time and helps optimize long-running tasks.
- Leaks: Identifies memory leaks in the app, helping reduce excessive memory usage and crashes.
- Allocations: Shows memory allocation and helps track down excessive memory usage that could lead to poor performance.
Example:
// Time Profiler shows that a method is taking too long
// Use Instruments to optimize performance by refactoring the code
- Efficient UI Updates:
- Use lazy loading to only load data and views when needed, rather than upfront.
- Avoid unnecessary view updates by making sure you only update the UI when required.
- Leverage offscreen rendering optimizations to reduce overdraw, where the same pixel is rendered multiple times.
- Memory Management:
- Avoid retain cycles by using weak references where necessary.
- Optimize image handling by compressing images or using UIImage(named:) appropriately
- Reduce memory usage by limiting the number of large objects held in memory.
- Concurrency:
- Offload heavy operations (e.g., network calls or data processing) to background threads using GCD or NSOperationQueue to prevent blocking the main thread and causing UI freezes.
- Use DispatchQueue.async to handle non-UI tasks in the background.
- Network Performance:
- Minimize network requests by batching multiple requests or caching data locally.
- Use URLSession to implement efficient network calls and download data asynchronously.
Evaluating Responses:
A good candidate should demonstrate familiarity with Xcode’s debugging tools like Instruments, and they should provide specific techniques for optimizing memory, UI updates, and concurrency. They should also be able to discuss real-world scenarios where these techniques can improve performance and user experience.
15. Can you explain the concept of Protocol-Oriented Programming in Swift?
Question Explanation:
Protocol-Oriented Programming (POP) is a core principle in Swift that encourages developers to focus on defining behavior using protocols rather than class inheritance. Understanding POP allows developers to build modular, reusable, and flexible code. This iOS interview question will assist with further understanding.
Expected Answer:
- Protocol-Oriented Programming (POP) is a paradigm in Swift that emphasizes the use of protocols to define blueprints of methods, properties, and other requirements. Instead of relying on class inheritance, Swift encourages developers to define behavior using protocols and extensions.
Example:
protocol Drivable {
var speed: Double { get }
func drive()
}
extension Drivable {
func drive() {
print("Driving at \(speed) km/h")
}
}
struct Car: Drivable {
var speed: Double
}
let myCar = Car(speed: 120)
myCar.drive() // Outputs: Driving at 120 km/h
- Key Concepts of POP:
- Protocols: Define a set of methods and properties that conforming types must implement. Protocols can be used by both structs and classes.
- Extensions: Allow developers to add functionality to types (e.g., classes, structs, and enums) even when you don’t have access to the original source code. This supports code reuse and prevents code duplication.
- Value Types vs. Reference Types: Swift’s POP works well with value types (structs, enums), which are more efficient and safer to use than reference types (classes).
- Advantages:
- Composition over Inheritance: Instead of inheriting behavior from a superclass, protocols allow developers to compose complex behaviors from multiple protocols.
- Type Safety and Reusability: By using protocols, developers can define generic behavior that works with multiple types, increasing code reusability and type safety.
Example of protocol composition:
protocol Flyable {
func fly()
}
protocol Swimmable {
func swim()
}
struct Duck: Flyable, Swimmable {
func fly() { print("Duck is flying") }
func swim() { print("Duck is swimming") }
}
Evaluating Responses:
The candidate should clearly explain the core principles of Protocol-Oriented Programming, such as the use of protocols, extensions, and the advantages over class-based inheritance. The answer should include practical examples of how protocols and extensions can improve code flexibility and reusability. Advanced answers might touch on how POP fits into Swift’s overall design philosophy, focusing on safety and performance.
iOS Interview Questions Conclusion
When interviewing iOS engineers, the questions above will help you assess not only a candidate’s technical proficiency but also their ability to solve real-world problems in mobile app development. These questions cover core iOS frameworks, app architecture, design patterns, and performance optimization, ensuring you identify candidates who can build high-quality, scalable iOS applications.