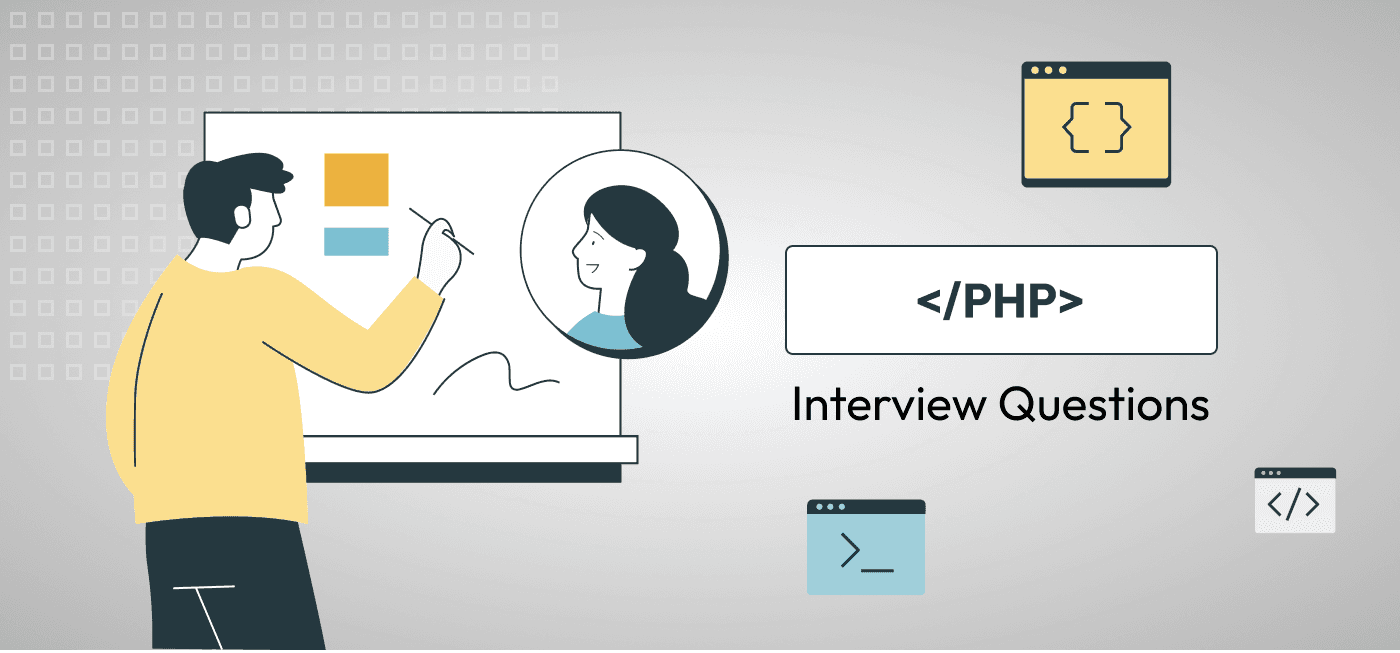
Hiring + recruiting | Blog Post
15 PHP Interview Questions for Hiring PHP Engineers
Todd Adams
Share this post
In the competitive field of web development, finding proficient PHP engineers is crucial for building robust and dynamic web applications. The following set of interview questions is designed to assess a candidate’s expertise in PHP, covering both fundamental concepts and advanced topics. These PHP interview questions will help you gauge a candidate’s ability to write clean, efficient, and secure PHP code, as well as their problem-solving skills and understanding of best practices in PHP development.
PHP Interview Questions
1. What are the key differences between PHP 5 and PHP 7?
Question Explanation:
Understanding the differences between PHP 5 and PHP 7 is essential as it highlights improvements in performance, features, and security. This PHP interview question tests a candidate’s knowledge of PHP’s evolution and their ability to work with newer versions.
Expected Answer:
PHP 7 introduced several significant changes and improvements over PHP 5. Some of the key differences include:
- Performance: PHP 7 is known for its performance enhancements, often being twice as fast as PHP 5.6 in executing code, thanks to the new Zend Engine 3.0.
- Type Declarations: PHP 7 introduced scalar type declarations (int, float, bool, string) and return type declarations, enabling stricter type checks and reducing bugs.
function addNumbers(int $a, int $b): int {
return $a + $b;
}
- Error Handling: PHP 7 replaced many fatal errors with exceptions, making it easier to handle errors gracefully using try-catch blocks.
try {
$result = 10 / 0;
} catch (DivisionByZeroError $e) {
echo "Caught exception: " . $e->getMessage();
}
- New Operators: New operators like the null coalescing operator (
??
) and the spaceship operator (<=>
) were added to simplify common coding patterns.
// Null coalescing operator
$value = $data['key'] ?? 'default';
// Spaceship operator
$comparison = 1 <=> 1; // Returns 0
- Anonymous Classes: PHP 7 introduced support for anonymous classes, allowing for quick and simple object creation without the need for named classes.
$obj = new class {
public function sayHello() {
return "Hello!";
}
};
- Deprecated and Removed Features: Several old and deprecated features were removed in PHP 7, including the
mysql_*
functions, which were replaced by MySQLi or PDO.
Evaluating Responses:
Look for answers that mention performance improvements, new language features like type declarations, changes in error handling, and the introduction of new operators. A thorough understanding of these differences indicates that the candidate is well-versed with PHP 7’s advancements.
2. How does PHP handle error reporting, and how can you configure error reporting settings?
Question Explanation:
Error handling is a critical aspect of development, as it helps in identifying and debugging issues efficiently. This PHP interview question evaluates a candidate’s knowledge of error reporting mechanisms in PHP and their ability to configure error settings.
Expected Answer:
PHP handles error reporting through a combination of configuration settings and built-in functions. Here are key points:
- Error Reporting Levels: PHP defines several levels of error reporting, such as E_ERROR, E_WARNING, E_NOTICE, and E_ALL. These constants represent different types of errors and warnings.
- Configuring Error Reporting:
- php.ini: Error reporting can be configured in the
php.ini
file using directives likeerror_reporting
,display_errors
, andlog_errors
. - Runtime Configuration: Error reporting can also be configured at runtime using the
error_reporting()
function and theini_set()
function.
- php.ini: Error reporting can be configured in the
error_reporting = E_ALL
display_errors = On
log_errors = On
error_log = /path/to/error.log
- Custom Error Handlers: PHP allows the creation of custom error handlers using the
set_error_handler()
function, enabling more sophisticated error handling strategies.
function customErrorHandler($errno, $errstr, $errfile, $errline) {
// Custom error handling logic
}
set_error_handler('customErrorHandler');
Evaluating Responses:
Look for responses that cover the different error reporting levels, methods of configuring error reporting both in php.ini
and at runtime, and the use of custom error handlers. A comprehensive answer indicates a solid understanding of PHP’s error handling mechanisms.
3. Explain the concept of sessions in PHP. How do you start a session and store session variables?
Question Explanation:
Sessions are crucial for maintaining state across multiple web pages in a PHP application. This question assesses a candidate’s understanding of how sessions work and their practical implementation in PHP.
Expected Answer:
Sessions in PHP allow you to store user information across multiple pages for the duration of a user’s visit. Here’s how you can start a session and store session variables:
- Starting a Session: To start a session, use the
session_start()
function. This function must be called at the beginning of the script, before any output is sent to the browser.
session_start();
- Storing Session Variables: Once a session is started, you can store session variables in the
$_SESSION
superglobal array.
$_SESSION['username'] = 'JohnDoe';
$_SESSION['email'] = 'john.doe@example.com';
- Retrieving Session Variables: To retrieve session variables, simply access the
$_SESSION
array.
echo $_SESSION['username']; // Outputs: JohnDoe
- Destroying a Session: To destroy a session and unset all session variables, use
session_unset()
andsession_destroy()
.
session_unset();
session_destroy();
Evaluating Responses:
Look for answers that include starting a session with session_start()
, storing and retrieving session variables using the $_SESSION
array, and properly destroying sessions. A detailed answer demonstrates practical knowledge of managing user sessions in PHP.
4. What are PHP Data Objects (PDO), and why should you use them over MySQLi?
Question Explanation:
PDO and MySQLi are two popular methods for interacting with databases in PHP. This PHP interview question evaluates a candidate’s understanding of PDO and its advantages over MySQLi.
Expected Answer:
PHP Data Objects (PDO) is a database access layer providing a uniform method of accessing multiple databases. Here are the key advantages of using PDO over MySQLi:
- Database Agnosticism: PDO supports 12 different database drivers (e.g., MySQL, PostgreSQL, SQLite), allowing you to switch databases without changing your code, provided that you use the same SQL syntax.
$dsn = 'mysql:host=localhost;dbname=testdb';
$username = 'root';
$password = '';
$pdo = new PDO($dsn, $username, $password);
- Prepared Statements: PDO supports prepared statements, which help prevent SQL injection by separating SQL logic from data.
$stmt = $pdo->prepare('SELECT * FROM users WHERE email = :email');
$stmt->execute(['email' => 'john.doe@example.com']);
$user = $stmt->fetch();
- Exception Handling: PDO uses exceptions to handle errors, providing a consistent and robust error management mechanism.
try {
$pdo = new PDO($dsn, $username, $password);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
- Flexibility and Features: PDO provides various features like transaction management, named placeholders, and fetching modes, which offer greater flexibility in handling database operations.
$pdo->beginTransaction();
$stmt = $pdo->prepare('INSERT INTO users (name, email) VALUES (:name, :email)');
$stmt->execute(['name' => 'John Doe', 'email' => 'john.doe@example.com']);
$pdo->commit();
Evaluating Responses:
Look for responses that highlight PDO’s database-agnostic nature, support for prepared statements, robust exception handling, and additional features like transaction management. A thorough understanding of these points indicates a strong grasp of using PDO for database interactions in PHP.
5. Describe the use of namespaces in PHP. How do they help in organizing code?
Question Explanation:
Namespaces are a way to encapsulate and group related code, preventing name collisions and improving code organization. This PHP interview question evaluates a candidate’s understanding of how namespaces work and their benefits in PHP development.
Expected Answer:
Namespaces in PHP are used to organize code into logical groups and to prevent name conflicts between classes, functions, and constants. Here’s how namespaces help in organizing code:
- Defining Namespaces: Namespaces are defined using the
namespace
keyword at the beginning of a PHP file.
namespace MyProject\Utilities;
class Logger {
// Class code
}
- Using Namespaces: You can refer to classes, functions, or constants in a namespace by their fully qualified name, or by using the
use
keyword for easier reference.
use MyProject\Utilities\Logger;
$logger = new Logger();
- Preventing Name Collisions: Namespaces allow multiple libraries or code segments to coexist without conflict, even if they define classes, functions, or constants with the same name.
namespace LibraryA;
class Logger {
// Class code
}
namespace LibraryB;
class Logger {
// Class code
}
- Improving Code Organization: Namespaces logically group related code, making it easier to maintain and understand. This is particularly useful in large projects.
namespace MyApp\Controllers;
class UserController {
// Class code
}
namespace MyApp\Models;
class User {
// Class code
}
Evaluating Responses:
Look for answers that explain how to define and use namespaces, their role in preventing name collisions, and their benefits for code organization. A thorough understanding of namespaces indicates the candidate’s ability to manage complex codebases effectively.
6. What is Composer, and how does it facilitate PHP development?
Question Explanation:
Composer is a dependency manager for PHP, widely used to manage libraries and packages. This question assesses a candidate’s familiarity with Composer and its importance in modern PHP development.
Expected Answer:
Composer is a dependency management tool for PHP that simplifies the management of external libraries and packages. Here’s how it facilitates PHP development:
- Dependency Management: Composer allows developers to declare the libraries and dependencies their project requires, and it automatically handles the installation and updating of these dependencies.
// composer.json
{
"require": {
"monolog/monolog": "^2.0"
}
}
- Autoloading: Composer provides an autoloading mechanism for classes, which eliminates the need for manual
require
orinclude
statements. This autoloading is configured in thecomposer.json
file.
require 'vendor/autoload.php';
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
$log = new Logger('name');
$log->pushHandler(new StreamHandler('app.log', Logger::WARNING));
- Version Control: Composer uses version constraints to ensure compatibility with library dependencies, allowing for flexible version management.
{
"require": {
"vendor/package": "^1.3"
}
}
- Community and Ecosystem: Composer is integrated with Packagist, a large repository of PHP packages, making it easy to find and use third-party libraries. This fosters a vibrant ecosystem and accelerates development.
Evaluating Responses:
Look for answers that explain Composer’s role in dependency management, autoloading, version control, and its integration with Packagist. A detailed understanding of Composer indicates that the candidate can efficiently manage dependencies and streamline development workflows.
7. Explain how to use the filter_var
function in PHP. Provide examples of common use cases.
Question Explanation:
The filter_var
function is used to validate and sanitize data. This PHP interview question assesses a candidate’s knowledge of data validation and sanitation in PHP, which is critical for security and data integrity.
Expected Answer:
The filter_var
function in PHP is used to both validate and sanitize data. Here are some common use cases and examples:
- Validating Email Addresses: Ensures the input is a valid email address.
$email = "test@example.com";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Valid email address.";
} else {
echo "Invalid email address.";
}
- Sanitizing Strings: Removes or encodes unwanted characters.
$string = "<h1>Hello, World!</h1>";
$sanitizedString = filter_var($string, FILTER_SANITIZE_STRING);
echo $sanitizedString; // Outputs: Hello, World!
- Validating URLs: Ensures the input is a valid URL.
$url = "https://www.example.com";
if (filter_var($url, FILTER_VALIDATE_URL)) {
echo "Valid URL.";
} else {
echo "Invalid URL.";
}
- Validating Integers: Checks if the input is a valid integer.
$int = "123";
if (filter_var($int, FILTER_VALIDATE_INT)) {
echo "Valid integer.";
} else {
echo "Invalid integer.";
}
Evaluating Responses:
Look for answers that demonstrate how to use filter_var
for common validation and sanitation tasks, such as validating email addresses, URLs, and integers, and sanitizing strings. A thorough understanding indicates the candidate’s ability to handle user input securely and correctly.
8. How can you implement dependency injection in PHP?
Question Explanation:
Dependency injection is a design pattern used to implement IoC (Inversion of Control), allowing for better modularity and testing. This PHP interview question assesses a candidate’s understanding and ability to implement dependency injection in PHP.
Expected Answer:
Dependency injection (DI) is a technique in which an object receives its dependencies from an external source rather than creating them itself. Here’s how you can implement DI in PHP:
- Constructor Injection: Dependencies are passed to the object via its constructor.
class Database {
// Database connection details
}
class UserRepository {
private $database;
public function __construct(Database $database) {
$this->database = $database;
}
}
$database = new Database();
$userRepository = new UserRepository($database);
- Setter Injection: Dependencies are provided through setter methods.
class UserRepository {
private $database;
public function setDatabase(Database $database) {
$this->database = $database;
}
}
$database = new Database();
$userRepository = new UserRepository();
$userRepository->setDatabase($database);
- Interface Injection: Dependencies are passed through an interface method, which the dependent class implements.
interface DatabaseAwareInterface {
public function setDatabase(Database $database);
}
class UserRepository implements DatabaseAwareInterface {
private $database;
public function setDatabase(Database $database) {
$this->database = $database;
}
}
$database = new Database();
$userRepository = new UserRepository();
$userRepository->setDatabase($database);
- Using a DI Container: A dependency injection container manages and injects dependencies automatically.
use Pimple\Container;
$container = new Container();
$container['database'] = function ($c) {
return new Database();
};
$container['userRepository'] = function ($c) {
return new UserRepository($c['database']);
};
$userRepository = $container['userRepository'];
Evaluating Responses:
Look for answers that explain different DI techniques, including constructor injection, setter injection, and interface injection, as well as the use of DI containers. A comprehensive understanding indicates that the candidate can apply DI to create modular, testable, and maintainable code.
9. Discuss the differences between GET and POST methods in PHP. When should each be used?
Question Explanation:
Understanding the differences between GET and POST methods is fundamental to web development, as these methods are commonly used for data transmission between client and server. This question assesses a candidate’s knowledge of HTTP methods and their appropriate use cases.
Expected Answer:
GET and POST are two of the most common HTTP methods used in web development. Here are the key differences and appropriate use cases for each:
- GET Method:
- Data Transmission: GET requests append data to the URL as query parameters, making it visible in the URL.
- Idempotence: GET requests are idempotent, meaning multiple identical requests should have the same effect as a single request.
- Caching: GET requests can be cached by browsers and intermediate servers.
- Length Limitations: URLs have length limitations (usually around 2048 characters), which restricts the amount of data that can be sent.
- Use Cases: GET should be used for retrieving data without causing any side effects on the server, such as fetching a web page or searching for a query.
// Fetching data using GET
$searchQuery = $_GET['query'];
- POST Method:
- Data Transmission: POST requests send data in the request body, which is not visible in the URL.
- Non-Idempotence: POST requests are not idempotent, meaning multiple identical requests can have different effects.
- Caching: POST requests are not cached by default.
- No Length Limitations: POST does not have strict length limitations, making it suitable for sending large amounts of data.
- Use Cases: POST should be used for operations that modify server state or submit data, such as creating a new record, submitting a form, or uploading a file.
// Submitting data using POST
$username = $_POST['username'];
$password = $_POST['password'];
Evaluating Responses:
Look for answers that clearly differentiate between GET and POST methods in terms of data transmission, caching, idempotence, and appropriate use cases. A detailed understanding of these differences indicates the candidate’s ability to choose the correct method for various web development scenarios.
10. How do you handle file uploads in PHP securely?
Question Explanation:
Handling file uploads securely is crucial to prevent security vulnerabilities such as code injection and unauthorized access. This PHP interview question evaluates a candidate’s knowledge of secure file handling practices in PHP.
Expected Answer:
Handling file uploads securely in PHP involves several steps to ensure the uploaded files do not pose a security risk. Here are the key practices:
- Check File Upload Errors: Always check for errors during the file upload process using the
$_FILES['file']['error']
array.
if ($_FILES['file']['error'] !== UPLOAD_ERR_OK) {
die("File upload failed with error code " . $_FILES['file']['error']);
}
- Validate File Type: Validate the file type by checking the MIME type and file extension. This helps prevent the upload of malicious files.
$allowedTypes = ['image/jpeg', 'image/png', 'application/pdf'];
$fileType = mime_content_type($_FILES['file']['tmp_name']);
if (!in_array($fileType, $allowedTypes)) {
die("Invalid file type.");
}
- Limit File Size: Set a maximum file size limit to prevent large files from being uploaded, which can exhaust server resources.
$maxFileSize = 2 * 1024 * 1024; // 2 MB
if ($_FILES['file']['size'] > $maxFileSize) {
die("File size exceeds the limit of 2 MB.");
}
- Rename Files: Rename the uploaded files to prevent potential overwriting of existing files and avoid revealing the original file name.
$uploadDir = 'uploads/';
$newFileName = uniqid() . '-' . basename($_FILES['file']['name']);
$uploadFilePath = $uploadDir . $newFileName;
- Move Uploaded File: Use
move_uploaded_file()
to move the file from the temporary directory to the desired destination.
if (!move_uploaded_file($_FILES['file']['tmp_name'], $uploadFilePath)) {
die("Failed to move uploaded file.");
}
- Set Proper Permissions: Ensure the uploaded file has appropriate permissions to prevent execution of malicious scripts.
chmod($uploadFilePath, 0644);
Evaluating Responses:
Look for answers that include checking for errors, validating file types, limiting file size, renaming files, securely moving files, and setting proper permissions. A comprehensive understanding of these practices indicates the candidate’s ability to handle file uploads securely in PHP.
11. Explain how to create and use a PHP trait. What are the benefits of using traits?
Question Explanation:
Traits in PHP provide a mechanism for code reuse in single inheritance languages like PHP. This question assesses a candidate’s understanding of traits and their practical application in PHP.
Expected Answer:
Traits are a way to include reusable methods in multiple classes, allowing code reuse without the limitations of single inheritance. Here’s how to create and use a trait:
- Creating a Trait: Define a trait using the
trait
keyword.php
trait Logger {
public function log($message) {
echo "[LOG]: $message";
}
}
- Using a Trait in a Class: Use the
use
keyword inside a class to include the trait’s methods.
class User {
use Logger;
public function createUser($name) {
$this->log("Creating user: $name");
// Additional code to create user
}
}
$user = new User();
$user->createUser('John Doe'); // Outputs: [LOG]: Creating user: John Doe
- Resolving Conflicts: If a class uses multiple traits with methods of the same name, use the
insteadof
andas
operators to resolve conflicts.
trait Logger {
public function log($message) {
echo "[LOG]: $message";
}
}
trait FileLogger {
public function log($message) {
echo "[FILE LOG]: $message";
}
}
class User {
use Logger, FileLogger {
FileLogger::log insteadof Logger;
Logger::log as logInfo;
}
public function createUser($name) {
$this->log("Creating user: $name"); // Outputs: [FILE LOG]: Creating user: $name
$this->logInfo("User created"); // Outputs: [LOG]: User created
}
}
Evaluating Responses:
Look for answers that explain how to define and use traits, and how to resolve conflicts when multiple traits are used. A thorough understanding of traits indicates that the candidate can effectively utilize them for code reuse and manage potential method conflicts.
12. What are some common security vulnerabilities in PHP applications, and how can you mitigate them?
Question Explanation:
Security is critical in web development. This PHP interview question evaluates a candidate’s knowledge of common security vulnerabilities in PHP applications and their ability to implement measures to mitigate these risks.
Expected Answer:
Some common security vulnerabilities in PHP applications include SQL injection, cross-site scripting (XSS), cross-site request forgery (CSRF), and file inclusion vulnerabilities. Here’s how to mitigate them:
- SQL Injection: Use prepared statements and parameterized queries to prevent SQL injection attacks.
$stmt = $pdo->prepare('SELECT * FROM users WHERE email = :email');
$stmt->execute(['email' => $email]);
$user = $stmt->fetch();
- Cross-Site Scripting (XSS): Sanitize user input and encode output to prevent XSS attacks.
$input = htmlspecialchars($_POST['input'], ENT_QUOTES, 'UTF-8');
echo $input;
- Cross-Site Request Forgery (CSRF): Use CSRF tokens to protect against CSRF attacks.
// Generate CSRF token
$token = bin2hex(random_bytes(32));
$_SESSION['csrf_token'] = $token;
// Include CSRF token in form
echo '<input type="hidden" name="csrf_token" value="' . $token . '">';
// Validate CSRF token
if ($_POST['csrf_token'] !== $_SESSION['csrf_token']) {
die('Invalid CSRF token');
}
- File Inclusion Vulnerabilities: Use whitelisting to validate file paths and avoid using user input directly in file inclusion functions.
$allowedFiles = ['file1.php', 'file2.php'];
if (in_array($_GET['file'], $allowedFiles)) {
include $_GET['file'];
} else {
die('Invalid file');
}
- Secure Configuration: Disable dangerous PHP functions and configure PHP securely.
disable_functions = "exec,passthru,shell_exec,system"
Evaluating Responses:
Look for answers that include specific examples of vulnerabilities like SQL injection, XSS, CSRF, and file inclusion, along with concrete mitigation strategies such as prepared statements, input sanitization, CSRF tokens, and secure configuration. A detailed understanding of these vulnerabilities and their mitigations indicates the candidate’s ability to develop secure PHP applications.
13. How does PHP’s garbage collection mechanism work?
Question Explanation:
Understanding PHP’s garbage collection mechanism is important for optimizing memory usage and performance. This question evaluates a candidate’s knowledge of how PHP handles memory management and garbage collection.
Expected Answer:
PHP’s garbage collection mechanism is responsible for managing memory by cleaning up unused or “garbage” objects to free up memory. Here’s how it works:
- Reference Counting: PHP uses a reference counting mechanism to track the number of references to a variable. When the reference count drops to zero, the memory occupied by the variable is deallocated.
$a = new stdClass();
$b = $a;
unset($a);
// $b still holds a reference to the object, so it's not garbage collected
unset($b);
// Now the object is garbage collected
- Cyclic Garbage Collection: PHP 5.3 introduced a cyclic garbage collector to handle circular references (i.e., objects that reference each other), which reference counting alone cannot manage.
class Node {
public $reference;
}
$a = new Node();
$b = new Node();
$a->reference = $b;
$b->reference = $a;
// Circular reference
unset($a, $b);
// Cyclic garbage collector will clean up the circular references
- Garbage Collection Cycles: The cyclic garbage collector runs periodically to detect and clean up circular references. The
gc_collect_cycles()
function can be used to trigger a garbage collection cycle manually.
gc_enable(); // Enable garbage collection
gc_collect_cycles(); // Force garbage collection
Evaluating Responses:
Look for answers that explain reference counting, cyclic garbage collection, and the purpose of the gc_collect_cycles()
function. A thorough understanding of these concepts indicates that the candidate can manage memory efficiently in PHP applications.
14. Describe how to use PHP’s cURL library to make HTTP requests.
Question Explanation:
The cURL library is commonly used in PHP for making HTTP requests. This PHP interview question assesses a candidate’s ability to use cURL for interacting with external APIs and services.
Expected Answer:
PHP’s cURL library provides a powerful way to make HTTP requests. Here’s how you can use it:
- Initializing cURL Session: Use
curl_init()
to initialize a new cURL session.
$ch = curl_init();
- Setting Options: Use
curl_setopt()
to set various options for the cURL session, such as the URL and request method.
curl_setopt($ch, CURLOPT_URL, "https://api.example.com/data");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
- Executing the Request: Use
curl_exec()
to execute the cURL session and fetch the response.
$response = curl_exec($ch);
- Error Handling: Use
curl_errno()
andcurl_error()
to handle any errors that occur during the request.
if (curl_errno($ch)) {
echo 'Error: ' . curl_error($ch);
}
- Closing the Session: Use
curl_close()
to close the cURL session and free up resources.
curl_close($ch);
- Example – GET Request:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://api.example.com/data");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error: ' . curl_error($ch);
} else {
echo $response;
}
curl_close($ch);
- Example – POST Request:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://api.example.com/data");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, "key1=value1&key2=value2");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error: ' . curl_error($ch);
} else {
echo $response;
}
curl_close($ch);
Evaluating Responses:
Look for answers that explain the basic steps of using cURL: initializing the session, setting options, executing the request, handling errors, and closing the session. A detailed understanding of these steps indicates the candidate’s ability to use cURL for HTTP requests effectively.
15. What is the purpose of the __autoload
function and how does it differ from spl_autoload_register
?
Question Explanation:
Autoloading is an essential feature for automatically loading classes. This question assesses a candidate’s understanding of PHP’s autoloading mechanisms and best practices.
Expected Answer:
The __autoload
function and spl_autoload_register
are both used for automatically loading classes in PHP, but they differ in flexibility and best practices.
__autoload
Function:- The
__autoload
function is a magic function that PHP calls automatically when an undefined class is instantiated. - It can only define a single autoloader function, which makes it less flexible.
- The
function __autoload($class_name) {
include $class_name . '.php';
}
spl_autoload_register
Function:
spl_autoload_register
allows you to register multiple autoload functions, providing more flexibility.- It is the preferred method for autoloading classes in modern PHP development.
- It supports anonymous functions and class methods as autoloaders.
spl_autoload_register(function ($class_name) {
include $class_name . '.php';
});
// Registering multiple autoloaders
spl_autoload_register('myAutoloader1');
spl_autoload_register('myAutoloader2');
- Advantages of
spl_autoload_register
:- Multiple Autoloaders: You can register multiple autoload functions, allowing for better modularization.
- Compatibility: It is compatible with namespaces and can handle more complex class loading scenarios.
- Best Practice: Using
spl_autoload_register
aligns with modern PHP practices and standards.
Evaluating Responses:
Look for answers that explain the differences between __autoload
and spl_autoload_register
, emphasizing the flexibility and advantages of spl_autoload_register
. A comprehensive understanding indicates the candidate’s ability to implement efficient and maintainable autoloading in PHP applications.
Conclusion
The PHP interview questions listed above are crafted to delve into a candidate’s knowledge and experience with PHP. They cover a range of topics from basic syntax and error handling to advanced concepts like dependency injection and security practices. By posing these questions, you can better assess a candidate’s technical abilities, problem-solving skills, and understanding of best practices in PHP development, ensuring that you hire the most competent and reliable PHP engineers for your team.