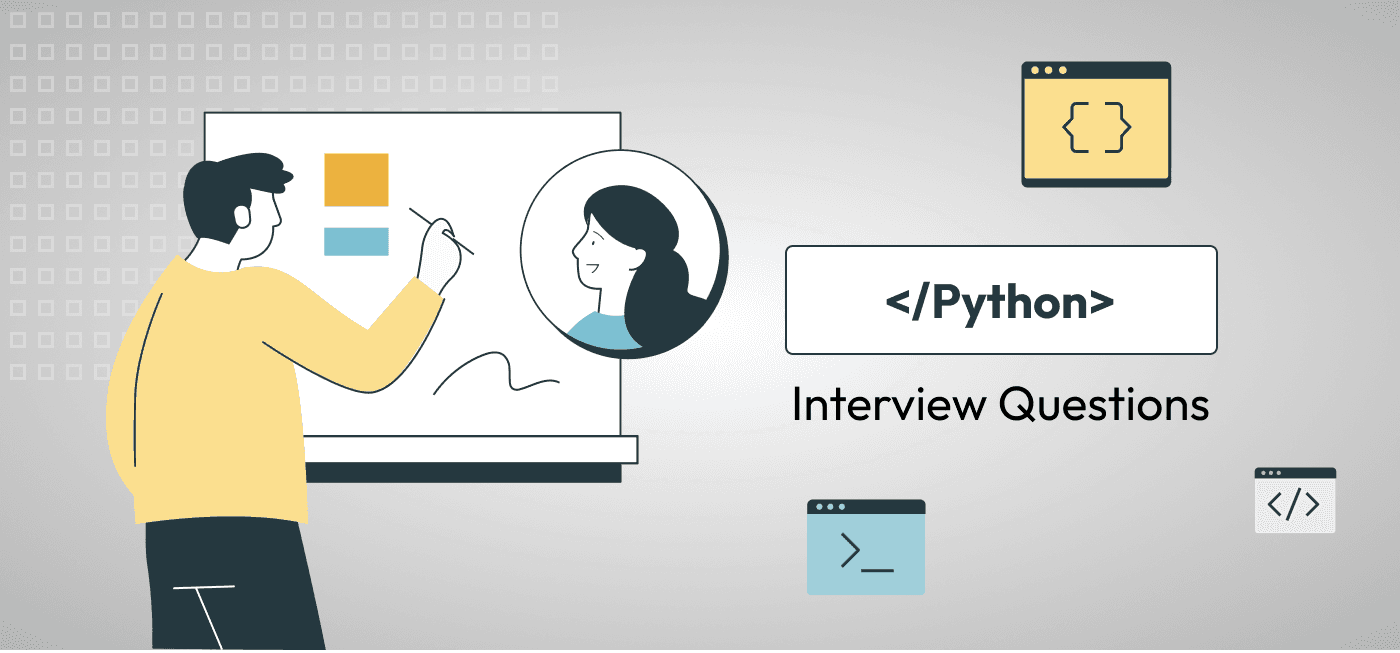
Hiring + recruiting | Blog Post
15 Python Interview Questions for Hiring Python Engineers
Todd Adams
Share this post
When hiring Python engineers, it’s essential to assess their understanding of core Python concepts, ability to write efficient and clean code, and their problem-solving capabilities. Below are 15 Python interview questions designed to evaluate a candidate’s proficiency with Python, covering a range of topics from basic syntax to more advanced features and best practices.
Python Interview Questions
1. What are Python’s key features, and how do they contribute to its popularity?
Question Explanation
This Python Interview question aims to assess a candidate’s understanding of Python’s core features that make it a popular language for developers. It reveals whether the candidate is aware of the advantages that Python offers over other languages.
Expected Answer
Python is known for several key features that have contributed to its popularity:
- Easy to Learn and Use: Python has a simple and clean syntax that closely resembles natural language, making it accessible for beginners and allowing experienced developers to write clear and maintainable code.
- Interpreted Language: Python does not need compilation, which allows for quicker development cycles and easier debugging, as errors can be caught at runtime.
- Versatile and Extensive Libraries: Python comes with a large standard library and a vibrant ecosystem of third-party packages, which makes it suitable for a wide range of applications, from web development to data science.
- Cross-Platform Compatibility: Python code runs on various platforms without modification, which is ideal for developing software that needs to be portable across different operating systems.
- Dynamic Typing: Python’s dynamic type system allows variables to be used without declaring their type, which simplifies code and allows more flexibility.
- Object-Oriented: Python supports object-oriented programming, which is useful for organizing complex software projects and promoting code reuse.
These features make Python a go-to language for many developers, particularly in fields like web development, data analysis, artificial intelligence, and automation.
Evaluating Responses
- Comprehensive Understanding: The candidate should mention most, if not all, of the above features, highlighting their importance.
- Clarity: The explanation should be clear, demonstrating the candidate’s ability to communicate technical concepts effectively.
- Contextual Examples: Strong answers may include examples of how these features benefit Python developers in real-world applications.
2. Explain the difference between ‘deepcopy
‘ and 'shallowcopy'
in Python.
Question Explanation
This Python Interview question tests the candidate’s understanding of Python’s memory management and object handling, specifically how different types of copies affect the objects being duplicated.
Expected Answer
In Python, ‘copy'
refers to creating a new object that is a replica of an existing object. The difference between ‘shallowcopy'
and ‘deepcopy'
lies in how the elements within the objects are copied:
- Shallow Copy: A shallow copy creates a new object, but it inserts references into the new object that point to the objects found in the original. In other words, the copied object contains references to the same elements as the original object. For example, if the original object is a list containing other lists, the shallow copy will include references to the same inner lists, not copies of them.
import copy
original = [[1, 2, 3], [4, 5, 6]]
shallow_copy = copy.copy(original)
shallow_copy[0][0] = 99
print(original) # [[99, 2, 3], [4, 5, 6]]
print(shallow_copy) # [[99, 2, 3], [4, 5, 6]]
- Deep Copy: A deep copy creates a new object and recursively copies all objects found within the original, creating fully independent copies. This means that changes to the deep-copied object do not affect the original object.
deep_copy = copy.deepcopy(original)
deep_copy[0][0] = 100
print(original) # [[99, 2, 3], [4, 5, 6]]
print(deep_copy) # [[100, 2, 3], [4, 5, 6]]
The ‘deepcopy'
is more computationally expensive because it creates copies of all nested objects, whereas 'shallowcopy'
only copies the outer structure.
Evaluating Responses
- Accuracy: The candidate must correctly explain the difference and the impact of using one over the other.
- Examples: The best responses will include code examples or clear explanations that demonstrate the difference in behavior.
- Understanding of Use Cases: A good candidate may also discuss when each type of copy is appropriate, such as using shallow copies for performance reasons when deep copies are not necessary.
3. How does Python manage memory? Describe the role of reference counting and garbage collection.
Question Explanation
This Python Interview question checks whether the candidate understands how Python handles memory management, which is crucial for writing efficient code and avoiding memory leaks.
Expected Answer
Python uses a combination of reference counting and garbage collection to manage memory:
- Reference Counting: Every object in Python maintains a reference count, which tracks the number of references pointing to that object. When an object is created, its reference count is set to one. Every time a new reference to that object is made, the reference count is incremented. When a reference to the object is deleted, the reference count is decremented. If the reference count drops to zero, the memory occupied by the object is deallocated immediately.
a = []
b = a
c = a
del b
del c
# The reference count for 'a' is now 1. Deleting 'a' will deallocate the memory.
del a
- Garbage Collection: While reference counting is effective, it cannot handle cyclic references (e.g., objects that reference each other). To address this, Python includes a cyclic garbage collector that periodically searches for groups of objects that are no longer accessible through any references but still reference each other. These cycles are then broken, and the objects are deallocated.
import gc
class Node:
def __init__(self, value):
self.value = value
self.next = None
a = Node(1)
b = Node(2)
a.next = b
b.next = a # Creates a cycle
del a
del b
# At this point, the garbage collector would need to collect 'a' and 'b'
gc.collect()
Python’s memory management system is automatic, reducing the likelihood of memory leaks, but understanding how it works allows developers to write more efficient and reliable code.
Evaluating Responses
- Depth of Understanding: The candidate should clearly explain both reference counting and garbage collection, including their roles and limitations.
- Examples: The candidate may provide code examples or scenarios where these memory management techniques are relevant.
- Practical Knowledge: Strong candidates might also mention how to manage memory efficiently, such as using
weakref
to avoid cyclic references.
4. What is a Python decorator, and how would you use it? Provide an example.
Question Explanation
This Python Interview question assesses the candidate’s ability to work with decorators, which are a powerful feature in Python for modifying the behavior of functions or methods in a clean and reusable way.
Expected Answer
A decorator in Python is a design pattern that allows you to modify the behavior of a function or method without permanently modifying its code. Decorators are applied using the ‘@decorator_name'
syntax above the function definition.
Decorators are often used for tasks like logging, enforcing access control, instrumentation, or memoization.
Here’s a simple example of a decorator that logs the execution time of a function:
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} executed in {end_time - start_time} seconds")
return result
return wrapper
@timing_decorator
def slow_function():
time.sleep(2)
return "Finished"
print(slow_function())
In this example, ‘timing_decorator'
wraps the ‘slow_function
‘ and logs the time it takes to execute. When ‘slow_function
‘ is called, the wrapper function inside ‘timing_decorator
‘ is executed, which in turn calls the original ‘slow_function
‘ and adds the timing logic.
Evaluating Responses
- Correctness: The candidate should correctly explain what decorators are and how they work in Python.
- Code Example: The candidate should provide a clear, working example of a decorator.
- Understanding of Use Cases: The best responses will include an explanation of common use cases for decorators and how they improve code reusability and clarity.
5. Explain the concept of list comprehensions. How do they differ from regular loops in Python?
Question Explanation
This Python Interview question examines the candidate’s understanding of Python’s concise syntax, particularly list comprehensions, which are a powerful feature for creating lists in a more readable and efficient way compared to traditional loops.
Expected Answer
List comprehensions are a concise way to create lists in Python. They allow for the creation of a new list by applying an expression to each element in an existing iterable (such as a list or range) and can include optional filtering with an if
statement.
The syntax of a list comprehension is:
[expression for item in iterable if condition]
- Expression: The operation or transformation to be applied to each element.
- Item: Each element in the iterable.
- Iterable: The collection of elements you want to iterate over.
- Condition: (Optional) A filter that selects which elements to include.
For example, the following list comprehension creates a list of squares for numbers from 0 to 9:
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Compared to a regular ‘for'
loop:
squares = []
for x in range(10):
squares.append(x**2)
Differences from Regular Loops:
- Conciseness: List comprehensions provide a more compact and readable way to create lists compared to traditional loops.
- Performance: List comprehensions are often faster because they are optimized for creating lists in a single statement.
- Readability: While list comprehensions can improve readability, overly complex ones can become harder to understand than equivalent loops, especially when they include multiple conditions or nested comprehensions.
Evaluating Responses
- Clarity: The candidate should clearly explain the syntax and purpose of list comprehensions.
- Code Example: A correct, working example should be provided, demonstrating the creation of a list using a comprehension.
- Comparison: The candidate should correctly compare list comprehensions to traditional loops, highlighting their advantages and potential drawbacks.
6. How do you handle exceptions in Python? Provide an example of a custom exception.
Question Explanation
This Python Interview question tests the candidate’s understanding of Python’s error handling mechanisms, specifically how exceptions are managed, and their ability to implement custom exceptions for more granular control over error handling.
Expected Answer
Exception handling in Python is primarily done using the try
, except
, else
, and finally
blocks:
- try: The block of code that might raise an exception is placed here.
- except: If an exception occurs in the
try
block, the code in theexcept
block is executed. Multipleexcept
blocks can be used to handle different exceptions. - else: This block runs if no exceptions are raised in the
try
block. - finally: This block is executed no matter what, whether an exception was raised or not, typically used for cleanup actions.
Here’s a basic example of exception handling:
try:
result = 10 / 0
except ZeroDivisionError as e:
print("Error: Cannot divide by zero!")
else:
print("Division successful:", result)
finally:
print("This block runs no matter what.")
Custom Exceptions: Python allows you to create your own exceptions by subclassing the built-in Exception
class. Custom exceptions are useful for specific errors that are unique to your application.
Example:
class CustomError(Exception):
def __init__(self, message):
self.message = message
def check_value(x):
if x < 0:
raise CustomError("Negative value not allowed!")
return x
try:
value = check_value(-5)
except CustomError as e:
print(e.message)
In this example, CustomError
is a user-defined exception that is raised if a negative value is encountered.
Evaluating Responses
- Correct Usage: The candidate should demonstrate a clear understanding of Python’s exception handling syntax and flow.
- Custom Exception: The candidate should correctly implement a custom exception, demonstrating how and when it would be used.
- Practical Application: Strong candidates may discuss when to use custom exceptions, such as for application-specific error conditions.
7. What is the Global Interpreter Lock (GIL) in Python, and how does it affect multithreading?
Question Explanation
This Python Interview question evaluates the candidate’s understanding of Python’s concurrency model, specifically the Global Interpreter Lock (GIL), which has significant implications for multithreaded programs in Python.
Expected Answer
The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, ensuring that only one thread executes Python bytecode at a time, even on multi-core systems. The GIL simplifies memory management by making it easier to implement reference counting for garbage collection, which is crucial for Python’s dynamic typing and object management.
Implications of the GIL:
- Multithreading: The GIL effectively serializes the execution of threads in Python, meaning that even in a multi-threaded program, only one thread can execute Python code at any given moment. This can severely limit the performance of CPU-bound multi-threaded programs, as they cannot take full advantage of multiple cores.
- I/O-bound vs. CPU-bound: The GIL is less of an issue for I/O-bound programs (e.g., network or file I/O), where threads spend much of their time waiting for external events. In these cases, Python can still achieve concurrency by switching between threads during I/O operations. However, for CPU-bound tasks that require heavy computation, the GIL can be a bottleneck.
- Workarounds: There are several strategies to mitigate the effects of the GIL, including:
- Multiprocessing: Instead of using threads, the
multiprocessing
module can be used to create separate processes, each with its own Python interpreter and GIL. This allows full utilization of multiple CPU cores. - C Extensions: Writing performance-critical code in C or using libraries that release the GIL during long-running operations can help.
- Alternative Python Implementations: Implementations like Jython or IronPython do not have a GIL, but they come with their own trade-offs.
- Multiprocessing: Instead of using threads, the
Evaluating Responses
- Understanding of the GIL: The candidate should clearly explain what the GIL is and why it exists.
- Impact on Multithreading: The response should include a discussion of how the GIL affects multi-threaded programs, particularly the difference between I/O-bound and CPU-bound tasks.
- Mitigation Strategies: A good answer will mention at least one strategy for working around the GIL’s limitations.
8. Describe how Python’s with
statement works and provide an example of its usage.
Question Explanation
This Python Interview question assesses the candidate’s familiarity with Python’s context management and the with
statement, which is essential for writing clean, reliable code that properly manages resources.
Expected Answer
The ‘with'
statement in Python is used for resource management and cleanup. It ensures that resources such as files or network connections are properly acquired and released, even if an error occurs during their use. The with
statement simplifies the try/finally pattern by automatically handling resource management.
The ‘with'
statement is commonly used with objects that support the context management protocol, which consists of two special methods: ‘__enter__
‘ and ‘__exit__'
:
- ‘
__enter__
‘: This method is executed when the execution flow enters the context of thewith
statement. It typically acquires the resource and returns it, which can then be assigned to a variable. - ‘
__exit__
‘: This method is executed when the execution flow exits the context, whether it does so normally or via an exception. It typically releases the resource and handles any cleanup.
Example with a file operation:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
# No need to explicitly close the file, as it is done automatically.
In this example, the file is automatically closed when the with
block is exited, regardless of whether an exception occurred.
Another example, using a custom context manager:
class ManagedResource:
def __enter__(self):
print("Resource acquired")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Resource released")
with ManagedResource() as resource:
print("Using resource")
This custom context manager prints messages when the resource is acquired and released.
Evaluating Responses
- Correct Explanation: The candidate should correctly explain how the
with
statement works, including its use of the ‘__enter__
‘ and ‘__exit__'
methods. - Examples: The candidate should provide a clear example of using the
with
statement, ideally with both a built-in context manager (like a file) and a custom one. - Understanding of Benefits: A strong response may include a discussion of why the ‘
with
‘ statement is preferred for resource management, particularly for avoiding resource leaks and ensuring proper cleanup.
9. How do you optimize the performance of a Python program? Mention some techniques or tools you would use.
Question Explanation
This Python Interview question tests the candidate’s understanding of performance bottlenecks in Python and their familiarity with techniques and tools for optimizing Python code. It also assesses their ability to identify when and how to optimize code effectively.
Expected Answer
Optimizing the performance of a Python program can involve several strategies, depending on the nature of the performance issues:
- Profiling the Code:
- Identify Bottlenecks: Before optimizing, it’s crucial to know where the bottlenecks are. Tools like
cProfile
,line_profiler
, andtimeit
can help identify slow parts of the code. - cProfile Example:
- Identify Bottlenecks: Before optimizing, it’s crucial to know where the bottlenecks are. Tools like
import cProfile
cProfile.run('your_function()')
- Optimizing Algorithms:
- Use Efficient Data Structures: Choose the right data structures (e.g.,
list
,set
,dict
) for the task. For instance, using aset
for membership tests instead of alist
can significantly improve performance. - Algorithmic Improvements: Improve the time complexity of algorithms. For example, replacing an
O(n^2)
algorithm with anO(n log n)
algorithm can drastically improve performance on large datasets.
- Use Efficient Data Structures: Choose the right data structures (e.g.,
- Built-in Functions and Libraries:
- Use Built-in Functions: Python’s built-in functions (e.g.,
sum()
,map()
,filter()
) are implemented in C and are generally faster than equivalent code written in pure Python. - Leverage Libraries: Use optimized libraries like
NumPy
for numerical operations, which are implemented in C and provide significant performance improvements.
- Use Built-in Functions: Python’s built-in functions (e.g.,
- Avoiding Global Variables:
- Local Variables: Accessing local variables is faster than global variables because Python optimizes local variable access. Refactor code to reduce reliance on global variables.
- Reducing Function Call Overhead:
- Inline Code: In performance-critical sections, consider reducing the number of function calls, which can add overhead.
- Use Generators: For large datasets, use generators instead of lists to save memory and potentially improve speed when dealing with large iterations.
- Concurrency and Parallelism:
- Multiprocessing: For CPU-bound tasks, use the
multiprocessing
module to parallelize work across multiple cores. - Asyncio: For I/O-bound tasks, use
asyncio
to handle asynchronous operations efficiently.
- Multiprocessing: For CPU-bound tasks, use the
- Memory Management:
- Use Generators: Generators use less memory than lists and can improve performance when dealing with large sequences of data.
- Weak References: Use weak references to objects that might be cleared from memory when not in use, which can help with memory management.
- JIT Compilers:
- Use PyPy: PyPy is a Just-In-Time (JIT) compiled implementation of Python that can significantly speed up execution of Python code.
Evaluating Responses
- Understanding of Profiling: The candidate should emphasize the importance of profiling code before optimization to ensure that efforts are focused on the real bottlenecks.
- Broad Knowledge: The answer should cover a range of techniques, showing that the candidate understands different aspects of optimization, from algorithmic efficiency to memory management.
- Practical Examples: Strong candidates will provide specific examples of tools and techniques, possibly even referencing past experiences with optimizing code.
10. Explain the difference between ‘__init__
‘ and ‘__new__
‘ methods in Python classes.
Question Explanation
This Python Interview question examines the candidate’s knowledge of Python’s object-oriented programming model, particularly the object creation process. Understanding the difference between ‘__init__
‘ and ‘__new__
‘ is important for working with custom object instantiation and subclasses.
Expected Answer
In Python, ‘__init__
‘ and ‘__new__
‘ are special methods involved in object creation and initialization, but they serve different purposes:
- ‘
__new__
‘Method:- Purpose: ‘
__new__
‘ is the method responsible for creating a new instance of a class. It is the first step in the instance creation process and is called before ‘__init__
‘. - Usage: ‘
__new__
‘ is a static method that takes the class itself as its first argument (cls
) and returns a new instance of that class. - Customization: It’s typically overridden in immutable classes like ‘
int
‘, ‘str
‘, or ‘tuple
‘, where modifying the instance after creation is not possible.
- Purpose: ‘
Example:
class MyClass:
def __new__(cls, *args, **kwargs):
print("Creating instance")
instance = super().__new__(cls)
return instance
def __init__(self, value):
print("Initializing instance")
self.value = value
obj = MyClass(10)
# Output:
# Creating instance
# Initializing instance
- ‘
__init__
‘ Method:- Purpose: ‘
__init__
‘ is the initializer method that sets up the initial state of the object after it has been created. It is called immediately after ‘__new__
‘and is where instance attributes are typically defined. - Usage: ‘
__init__
‘ takes the newly created instance (self
) as its first argument, along with any other arguments passed to the class constructor. It does not return anything (implicitly returns ‘None
‘).
- Purpose: ‘
Example:
class MyClass:
def __init__(self, value):
self.value = value
obj = MyClass(10)
Key Differences:
- ‘
__new__
‘ is involved in creating the object, while ‘__init__
‘ is used to initialize it. - ‘
__new__
‘ is a static method and is rarely overridden unless custom behavior is needed during object creation. In contrast, ‘__init__
‘ is commonly used to set up the object’s initial state.
Evaluating Responses
- Understanding of Roles: The candidate should clearly differentiate between the roles of ‘
__new__
‘ and ‘__init__
‘ in object creation and initialization. - Correct Examples: The candidate should provide code examples that correctly demonstrate how and when to use these methods.
- Advanced Understanding: Strong answers may include scenarios where overriding ‘
__new__
‘ is necessary, such as in singleton patterns or when working with immutable types.
11. How would you implement a Singleton pattern in Python?
Question Explanation
This Python Interview question evaluates the candidate’s understanding of design patterns and their ability to apply the Singleton pattern in Python. The Singleton pattern ensures that a class has only one instance and provides a global point of access to it.
Expected Answer
The Singleton pattern can be implemented in Python in several ways. Below are two common approaches:
- Using ‘
__new__
‘ Method:- Explanation: The ‘
__new__
‘ method is overridden to ensure that only one instance of the class is created. If an instance already exists, it returns the existing instance instead of creating a new one.
- Explanation: The ‘
Example:
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self, value):
self.value = value
obj1 = Singleton(10)
obj2 = Singleton(20)
print(obj1.value) # Output: 20
print(obj1 is obj2) # Output: True
- Using a Class Decorator:
- Explanation: A decorator can be used to wrap the class and control its instantiation, ensuring that only one instance is created.
Example:
def singleton(cls):
instances = {}
def get_instance(*args, **kwargs):
if cls not in instances:
instances[cls] = cls(*args, **kwargs)
return instances[cls]
return get_instance
@singleton
class Singleton:
def __init__(self, value):
self.value = value
obj1 = Singleton(10)
obj2 = Singleton(20)
print(obj1.value) # Output: 10
print(obj1 is obj2) # Output: True
Key Points:
- In both approaches, the Singleton ensures that only one instance of the class is created, and subsequent attempts to instantiate the class will return the same object.
- The ‘
__new__
‘ method is a more traditional way to implement the Singleton pattern, while the decorator approach is more Pythonic and leverages Python’s first-class function capabilities.
Evaluating Responses
- Correctness: The candidate should demonstrate a clear understanding of the Singleton pattern and provide a correct implementation.
- Flexibility: The response should include at least one approach, with the best responses covering multiple methods.
- Practical Understanding: The candidate may also discuss the pros and cons of using Singletons, such as global state management and potential issues with testing or scalability.
12. What are metaclasses in Python, and how would you use them?
Question Explanation
This Python Interview question tests the candidate’s understanding of Python’s class system, particularly metaclasses, which allow customization of class creation and behavior. Metaclasses are an advanced feature that is often used in frameworks and libraries to enforce patterns or inject behavior across multiple classes.
Expected Answer
A metaclass in Python is a class of a class, meaning it defines how a class behaves. A class in Python is an instance of its metaclass. By default, the metaclass for all classes in Python is ‘type
‘. Metaclasses are responsible for creating classes just as classes create instances.
Use Cases for Metaclasses:
- Enforcing Class Constraints: Metaclasses can be used to enforce certain constraints across a class hierarchy, such as ensuring that all classes have certain methods or attributes.
- Automatic Registration: Metaclasses can be used to automatically register classes in a global registry or with a factory.
- Custom Class Creation Logic: Metaclasses can modify the creation process of classes, such as adding methods, altering class attributes, or logging class creation.
How to Define and Use a Metaclass:
- To define a metaclass, you create a class that inherits from
type
and override its ‘__new__
‘ or ‘__init__
‘ methods.
Example:
class MyMeta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name}")
# Add a new method to the class
dct['greet'] = lambda self: f"Hello from {self.__class__.__name__}"
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
obj = MyClass()
print(obj.greet()) # Output: Hello from MyClass
In this example, ‘MyMeta
‘ is a metaclass that adds a ‘greet
‘ method to any class it creates. The class ‘MyClass
‘ is then created with MyMeta
as its metaclass, and when an instance of ‘MyClass
‘ is created, it has the ‘greet
‘ method.
Key Points:
- Metaclasses are a powerful feature for controlling how classes are created and can be used for metaprogramming tasks where you need to modify or enforce patterns across multiple classes.
- Use with Caution: Metaclasses add complexity and should be used sparingly. They are useful in frameworks or libraries where such control is necessary.
Evaluating Responses
- Understanding of Concepts: The candidate should demonstrate a solid understanding of what metaclasses are and why they might be used.
- Correct Example: The candidate should provide an example that clearly shows how to define and use a metaclass.
- Practical Application: Strong candidates might also discuss the scenarios where metaclasses are appropriate and the potential downsides, such as increased complexity.
13. Explain the difference between mutable and immutable types in Python. Provide examples.
Question Explanation
This Python Interview question assesses the candidate’s understanding of Python’s data types, particularly the difference between mutable and immutable objects, which is fundamental to how data is managed and manipulated in Python.
Expected Answer
In Python, data types can be classified as mutable or immutable based on whether their state (i.e., the content of the object) can be changed after they are created.
- Mutable Types:
- Definition: Mutable objects can be changed after they are created. This means you can alter their contents without creating a new object.
- Examples: Lists, dictionaries, sets, and bytearrays are mutable types.
Example of a mutable type (list):
my_list = [1, 2, 3]
my_list[0] = 10 # Modifies the list in place
print(my_list) # Output: [10, 2, 3]
- Immutable Types:
- Definition: Immutable objects cannot be changed once they are created. Any attempt to modify the object will result in a new object being created.
- Examples: Integers, floats, strings, tuples, and frozensets are immutable types.
Example of an immutable type (string):
my_string = "hello"
new_string = my_string.replace("h", "j")
print(my_string) # Output: hello (original string is unchanged)
print(new_string) # Output: jello (a new string is created)
Key Differences:
- In-place Modification: Mutable objects can be modified in place, whereas immutable objects require the creation of a new object to represent any change.
- Performance Considerations: Because immutable objects cannot be altered, they can be more efficient in certain contexts, such as when used as dictionary keys or in sets. Mutable objects require more careful handling to avoid unintended side effects from shared references.
Why This Matters: Understanding the difference is crucial when working with functions, as mutable objects passed as arguments can be altered within the function, leading to side effects, while immutable objects cannot.
Evaluating Responses
- Clarity of Explanation: The candidate should clearly define and differentiate between mutable and immutable types.
- Correct Examples: The candidate should provide accurate examples that demonstrate the difference.
- Practical Understanding: A strong response might also include a discussion of how mutability affects function arguments, performance, or data structure choices.
14. How do you use the *args
and **kwargs
syntax in Python functions? Provide examples.
Question Explanation
This Python Interview question evaluates the candidate’s understanding of Python’s flexible argument passing mechanisms, specifically the use of ‘*args
‘ and ‘**kwargs
‘ for handling variable numbers of arguments in functions.
Expected Answer
In Python, ‘*args
‘ and ‘**kwargs
‘ are used to pass a variable number of arguments to a function:
- ‘
*args
‘:- Purpose: ‘
*args
‘ is used to pass a variable number of positional arguments to a function. The arguments are captured as a tuple. - Usage: When you use
'*args
‘ in a function definition, it allows the function to accept any number of positional arguments.
- Purpose: ‘
Example:
def my_function(*args):
for arg in args:
print(arg)
my_function(1, 2, 3)
# Output:
# 1
# 2
# 3
- ‘
**kwargs
‘:- Purpose: ‘
**kwargs
‘ is used to pass a variable number of keyword arguments to a function. The arguments are captured as a dictionary. - Usage: When you use
'**kwargs
‘ in a function definition, it allows the function to accept any number of keyword arguments.
- Purpose: ‘
Example:
def my_function(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
my_function(name="Alice", age=30)
# Output:
# name: Alice
# age: 30
Combining ‘*args
‘ and ‘**kwargs
‘:
- You can use both ‘
*args
‘ and ‘**kwargs
‘ in the same function to handle both positional and keyword arguments.
Example:
def my_function(*args, **kwargs):
print("Positional arguments:", args)
print("Keyword arguments:", kwargs)
my_function(1, 2, 3, name="Alice", age=30)
# Output:
# Positional arguments: (1, 2, 3)
# Keyword arguments: {'name': 'Alice', 'age': 30}
Practical Uses:
- Function Wrappers/Decorators: ‘
*args
‘ and ‘**kwargs
‘ are often used in decorators or higher-order functions to pass arguments to the wrapped function. - Flexible APIs: They are useful in functions that need to accept a flexible number of parameters, such as utility functions or library APIs.
Evaluating Responses
- Understanding of Concepts: The candidate should clearly explain what ‘
*args
‘ and ‘**kwargs
‘ are and how they work. - Correct Examples: The candidate should provide working examples demonstrating their use.
- Practical Application: Strong responses might include examples of where ‘
*args
‘ and ‘**kwargs
‘ are particularly useful, such as in decorators or when building flexible APIs.
15. What are Python generators, and how do they differ from regular functions? Provide an example.
Question Explanation
This Python Interview question examines the candidate’s understanding of Python generators, which are a special type of function that can be paused and resumed, allowing for more memory-efficient iteration over large datasets.
Expected Answer
Generators are a special type of function in Python that return an iterator. Unlike regular functions, which return a single value and terminate, generators use the yield
statement to produce a sequence of values lazily, meaning they generate values on the fly and can be paused and resumed.
Key Differences from Regular Functions:
- Yield vs. Return: In a regular function,
return
sends a value back to the caller and terminates the function. In a generator,yield
sends a value back to the caller but preserves the function’s state, allowing it to resume where it left off. - Memory Efficiency: Generators are more memory-efficient than functions that return a list because they generate values one at a time and do not store the entire sequence in memory.
- Lazy Evaluation: Generators produce items only as needed (lazily), which is particularly useful when dealing with large datasets or streams of data.
Example of a Generator:
def count_up_to(max):
count = 1
while count <= max:
yield count
count += 1
counter = count_up_to(5)
print(next(counter)) # Output: 1
print(next(counter)) # Output: 2
for num in counter:
print(num)
# Output:
# 3
# 4
# 5
In this example, the generator ‘count_up_to
‘ yields numbers from 1 up to ‘max
‘. Each call to ‘next()
‘ retrieves the next value, and the loop continues from where it last left off.
Practical Uses:
- Handling Large Data: Generators are useful for processing large datasets or streams of data where loading everything into memory would be impractical.
- Pipelining: Generators can be used to create data pipelines where each step processes a stream of data incrementally.
Evaluating Responses
- Understanding of Generators: The candidate should clearly explain how generators work and how they differ from regular functions.
- Correct Example: The candidate should provide an example that correctly demonstrates the use of
yield
and the benefits of using generators. - Practical Application: A strong answer might also discuss scenarios where generators are particularly advantageous, such as in data processing pipelines or when dealing with infinite sequences.
Python Interview Questions Conclusion
These questions are designed to probe a candidate’s understanding of Python, covering a range of topics from basic syntax to more advanced concepts. By assessing the responses to these questions, interviewers can gauge a candidate’s proficiency in Python and their ability to apply this knowledge to solve real-world problems effectively.