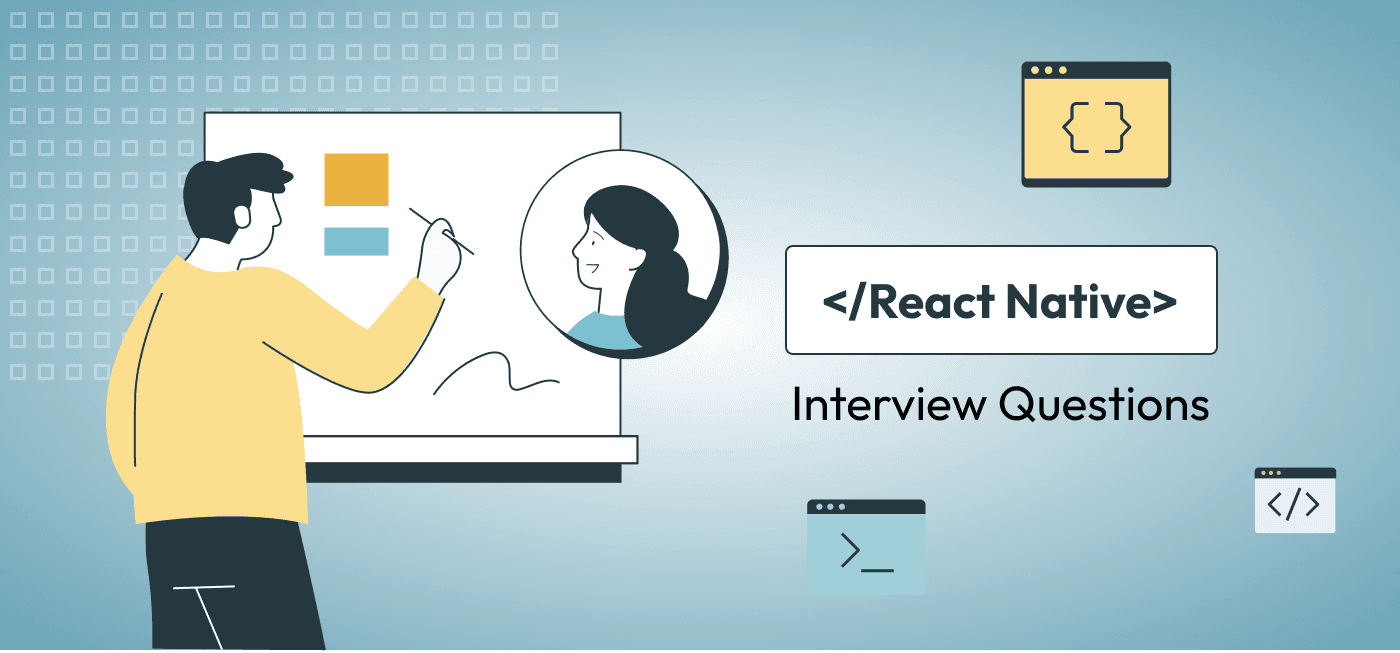
Hiring + recruiting | Blog Post
15 React Native Interview Questions for Hiring React Native Developers
Todd Adams
Share this post
React Native is a powerful framework for building cross-platform mobile applications using JavaScript and React. When hiring React Native developers, it’s essential to evaluate their understanding of the framework, ability to create efficient and maintainable code, and expertise in integrating platform-specific functionalities. This list of 15 React Native interview questions aims to help you assess candidates’ technical proficiency, problem-solving capabilities, and hands-on experience with the framework.
React Native Interview Questions
1. What are the key differences between React and React Native?
Question Explanation:
This React Native Developer interview question assesses the candidate’s understanding of how React Native extends React, emphasizing differences in their usage for web and mobile development. A strong answer shows familiarity with the core concepts shared by both frameworks and the unique aspects of React Native.
Expected Answer:
React is a library used for building user interfaces, primarily for web applications. It uses HTML and CSS for rendering UI components in the browser. React Native, on the other hand, is a framework for building cross-platform mobile applications. Instead of using HTML and CSS, it provides native components like <View>
, <Text>
, and <Image>
, which render directly to native platform components on Android and iOS.
React Native also includes a bridge that allows JavaScript to interact with native APIs, enabling the use of device-specific functionalities like camera access or push notifications. While React applications run in the browser, React Native apps run on mobile devices and are closer to native performance.
Key differences include:
- Rendering: React uses the DOM, while React Native uses native views.
- Styling: React uses CSS or CSS-in-JS libraries, while React Native uses a CSS-like
StyleSheet
. - Platform-Specific Code: React Native allows you to write platform-specific code using modules like
Platform
.
Evaluating Responses:
Look for candidates who clearly explain how React Native builds on React and articulate differences in rendering and styling. Candidates with practical experience might also mention challenges or benefits of using React Native for mobile development.
2. How does React Native achieve cross-platform development?
Question Explanation:
This React Native Developer interview question evaluates the candidate’s understanding of React Native’s architecture and how it allows JavaScript to interact with native code on both Android and iOS.
Expected Answer:
React Native achieves cross-platform development by using a bridge between JavaScript and native code. The JavaScript thread handles the application logic and UI, while native threads manage rendering and interactions with platform-specific APIs. The bridge facilitates communication between these threads, allowing developers to write a single JavaScript codebase that runs on both Android and iOS.
For instance:
- UI components like
<View>
or<Button>
are abstracted in React Native, but under the hood, they are converted into platform-specific native components. - JavaScript communicates with native APIs using asynchronous batched messaging through the bridge, enabling high performance while maintaining platform consistency.
This architecture ensures developers can leverage a unified codebase for most functionalities while writing platform-specific code when needed.
Evaluating Responses:
Candidates should demonstrate an understanding of the bridge mechanism and how it connects JavaScript to native layers. Strong responses may include references to performance optimization and examples of using native modules for platform-specific tasks.
3. What are the core components of React Native? Can you explain their usage with examples?
Question Explanation:
Understanding core components is fundamental to React Native development. This React Native Developer interview question assesses whether the candidate knows and has practical experience with React Native’s building blocks.
Expected Answer:
React Native provides core components that serve as the building blocks for mobile apps. Examples include:
<View>
: A container component used for layout and styling.
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Hello, World!</Text>
</View>
<Text>
: Used for displaying text.
<Text style={{ fontSize: 16, color: 'blue' }}>Welcome to React Native!</Text>
<Image>
: Displays images, supporting local and remote sources.
<Image source={{ uri: 'https://example.com/image.png' }} style={{ width: 100, height: 100 }} />
<ScrollView>
: A container for vertically or horizontally scrolling content.<FlatList>
and<SectionList>
: Optimized components for rendering lists.
These components are styled using React Native’s StyleSheet
, which is similar to CSS.
Evaluating Responses:
A good answer will include examples and explanations of how these components fit into app development. Candidates who mention best practices or their personal experience with these components stand out.
4. How would you handle state management in a React Native app?
Question Explanation:
State management is a critical concept in React Native apps, especially for maintaining and updating app data efficiently. This React Native Developer interview question explores the candidate’s familiarity with state management tools and strategies.
Expected Answer:
React Native offers multiple approaches for state management:
- React’s Built-in State and Context API:
Small applications can use React’suseState
anduseContext
hooks for managing local and shared state.
const [count, setCount] = useState(0);
<Button onPress={() => setCount(count + 1)} title={`Count: ${count}`} />;
For shared state:
const AppContext = React.createContext();
const AppProvider = ({ children }) => {
const [user, setUser] = useState(null);
return <AppContext.Provider value={{ user, setUser }}>{children}</AppContext.Provider>;
};
- Third-Party Libraries (e.g., Redux, MobX):
For complex applications, libraries like Redux are used for predictable state management.
import { createStore } from 'redux';
const store = createStore(reducer);
<Provider store={store}>
<App />
</Provider>;
- Recoil or Zustand:
Modern libraries that simplify state management for specific use cases.
Evaluating Responses:
Look for candidates who can explain multiple approaches, articulate when to use each, and provide examples. Strong candidates might discuss trade-offs between simplicity, scalability, and performance in state management.
5. Can you explain the difference between functional and class components in React Native?
Question Explanation:
This React Native Developer interview question assesses the candidate’s understanding of modern React Native practices, especially the shift towards functional components and React Hooks.
Expected Answer:
Class components and functional components are two ways to define components in React Native.
- Class Components:
These were the primary way to define components before React Hooks. They use ES6 classes and support lifecycle methods likecomponentDidMount
,componentDidUpdate
, andcomponentWillUnmount
.
class MyComponent extends React.Component {
state = { count: 0 };
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<Button onPress={this.increment} title={`Count: ${this.state.count}`} />
);
}
}
- Functional Components:
These are simpler and allow the use of hooks likeuseState
anduseEffect
to manage state and side effects. Functional components are now the recommended approach due to better readability and performance optimizations.
const MyComponent = () => {
const [count, setCount] = useState(0);
return (
<Button onPress={() => setCount(count + 1)} title={`Count: ${count}`} />
);
};
Key differences:
- Functional components are more concise and easier to test.
- React Hooks allow managing state and lifecycle in functional components without needing a class.
- Functional components avoid issues related to
this
binding.
Evaluating Responses:
A good candidate will articulate the differences clearly and demonstrate knowledge of hooks like useState
and useEffect
. Bonus points for mentioning the performance benefits of functional components and the modern preference for them.
6. How would you optimize the performance of a React Native application?
Question Explanation:
Optimizing performance is crucial for mobile applications, especially those targeting multiple platforms. This React Native Developer interview question evaluates the candidate’s ability to recognize bottlenecks and implement solutions.
Expected Answer:
Performance optimization in React Native can be achieved using several techniques:
- Avoid Unnecessary Re-Renders:
UseReact.memo
for functional components orPureComponent
for class components to prevent re-renders when props haven’t changed.
const MemoizedComponent = React.memo(MyComponent);
- Use FlatList for Large Lists:
ReplaceScrollView
withFlatList
for rendering long lists, as it supports virtualization and only renders visible items. - Optimize Images:
Use tools likereact-native-fast-image
for optimized image loading and caching. - Reduce JavaScript Overhead:
Minimize complex calculations and leverage background threads using libraries likereact-native-reanimated
orworker_threads
. - Minimize App Size:
Remove unused dependencies and use code-splitting to keep the JavaScript bundle size small. - Optimize Animation Performance:
PreferuseNativeDriver
for animations to offload work to the native thread.
Evaluating Responses:
Candidates should mention at least three optimization techniques with examples. Bonus points for addressing both rendering performance (e.g., list virtualization) and load-time optimizations (e.g., bundle size reduction).
7. What is the role of Flexbox in React Native, and how does it differ from CSS Flexbox?
Question Explanation:
This React Native Developer interview question assesses the candidate’s understanding of layout systems in React Native and their ability to style mobile UIs effectively.
Expected Answer:
Flexbox is the primary layout system in React Native, enabling developers to create responsive and flexible UIs. The core Flexbox properties in React Native include:
flexDirection
: Determines the primary axis direction (row
orcolumn
, default iscolumn
).justifyContent
: Aligns children along the main axis (e.g.,flex-start
,center
,space-between
).alignItems
: Aligns children along the cross-axis (e.g.,stretch
,flex-start
,center
).
Example usage:
<View style={{ flexDirection: 'row', justifyContent: 'space-between', alignItems: 'center' }}>
<Text>Item 1</Text>
<Text>Item 2</Text>
</View>
Key differences between React Native Flexbox and CSS Flexbox:
- The default
flexDirection
in React Native iscolumn
, whereas in CSS, it’srow
. - Units like
px
or%
aren’t used in React Native; dimensions are unitless. - React Native lacks some CSS-specific properties like
float
orz-index
.
Evaluating Responses:
Look for clarity on Flexbox properties and how they differ in React Native. Strong candidates might provide practical examples or share experiences solving layout challenges.
8. What are some common challenges when working with React Native, and how do you overcome them?
Question Explanation:
This React Native Developer interview question gauges the candidate’s practical experience with React Native and their ability to resolve typical development issues.
Expected Answer:
Some common challenges in React Native development include:
- Debugging and Error Tracking:
React Native apps can be harder to debug due to issues like async bridge errors. Tools like Flipper and Sentry can help troubleshoot and monitor errors. - Dependency Compatibility:
Third-party libraries sometimes have compatibility issues with specific React Native versions. Usingreact-native upgrade
or manually updating libraries often resolves this. - Platform-Specific Behaviors:
UI and functionality might behave differently on iOS and Android. Using thePlatform
module or writing platform-specific code helps manage these differences.
import { Platform } from 'react-native';
const platformSpecificStyle = Platform.OS === 'ios' ? styles.iosStyle : styles.androidStyle;
- Performance Bottlenecks:
Overloading the JavaScript thread can degrade performance. Moving heavy computations to native code or background threads improves efficiency. - Testing and Deployment:
Managing multiple environments and ensuring consistent performance across devices can be tricky. Tools like Appium for end-to-end testing and CodePush for OTA updates streamline the process.
Evaluating Responses:
Strong candidates will describe specific challenges they’ve faced, provide solutions, and explain what they learned. Look for real-world examples and their approach to debugging or resolving issues.
9. How do you implement navigation in a React Native application?
Question Explanation:
This React Native Developer interview question evaluates the candidate’s knowledge of navigation solutions in React Native, especially their experience with React Navigation, the most widely used library.
Expected Answer:
React Native uses libraries like React Navigation for implementing navigation between screens. React Navigation provides several types of navigators, such as:
- Stack Navigator:
Manages screens in a stack, allowing users to navigate back and forth.
import { createStackNavigator } from '@react-navigation/stack';
import { NavigationContainer } from '@react-navigation/native';
const Stack = createStackNavigator();
const App = () => (
<NavigationContainer>
<Stack.Navigator initialRouteName="Home">
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
- Tab Navigator:
Implements bottom or top tab navigation.
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
const Tab = createBottomTabNavigator();
const App = () => (
<NavigationContainer>
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeScreen} />
<Tab.Screen name="Settings" component={SettingsScreen} />
</Tab.Navigator>
</NavigationContainer>
);
- Drawer Navigator:
Provides a side drawer menu for navigation.
React Navigation also supports nested navigators, deep linking, and passing parameters between screens.
Evaluating Responses:
Candidates should mention React Navigation, demonstrate how to configure navigators, and discuss scenarios where different navigation types are appropriate. Bonus points for mentioning performance considerations, deep linking, or integration with Redux.
10. What is a native module, and when would you use one in React Native?
Question Explanation:
This React Native Developer interview question assesses the candidate’s understanding of extending React Native functionality by writing platform-specific native code.
Expected Answer:
A native module is a way to extend React Native functionality by writing code in platform-specific languages like Java (for Android) or Swift/Objective-C (for iOS). Native modules are useful when:
- A feature is not available in React Native or its libraries (e.g., advanced camera features).
- Platform-specific APIs need to be accessed directly.
- Performance-critical tasks like heavy computations or animations are required.
Example use case: Creating a custom native module to access a unique hardware feature:
- Android (Java):
@ReactMethod
public void customMethod(String message) {
Log.d("CustomModule", message);
}
- iOS (Objective-C):
RCT_EXPORT_METHOD(customMethod:(NSString *)message) {
NSLog(@"%@", message);
}
To integrate the module in JavaScript:
import { NativeModules } from 'react-native';
NativeModules.CustomModule.customMethod('Hello Native!');
Evaluating Responses:
Candidates should demonstrate a clear understanding of when to use native modules, with examples. Strong candidates will discuss the process of creating, integrating, and maintaining native modules.
11. How do you handle platform-specific code in React Native?
Question Explanation:
This React Native Developer interview question tests the candidate’s ability to write and manage code tailored to specific platforms while maintaining a unified codebase.
Expected Answer:
React Native offers several ways to handle platform-specific code:
Platform
Module:
Use thePlatform
module to conditionally render or execute platform-specific code.
import { Platform } from 'react-native';
const styles = Platform.OS === 'ios'
? { backgroundColor: 'blue' }
: { backgroundColor: 'green' };
- File Extensions (
.ios.js
and.android.js
):
Create separate files for platform-specific implementations.
Component.ios.js
Component.android.js
React Native automatically picks the correct file based on the platform.
- Platform-Specific Libraries:
Use platform-specific libraries (e.g.,react-native-gesture-handler
for Android touch gestures). - Third-Party Libraries:
Many libraries provide abstractions for platform-specific behavior (e.g.,react-native-device-info
).
Evaluating Responses:
Candidates should demonstrate an understanding of all three methods and provide examples. Strong responses will include best practices for maintaining a clean and manageable codebase.
12. Can you explain the purpose of the Metro bundler in React Native?
Question Explanation:
This React Native Developer interview question explores the candidate’s understanding of the React Native development environment, specifically the role of the Metro bundler.
Expected Answer:
The Metro bundler is the default JavaScript bundler for React Native. It is responsible for:
- Transpiling Code: Converts modern JavaScript (ES6/ES7) and JSX into code that can be executed by the JavaScript engine.
- Packaging: Bundles all the JavaScript files, assets, and dependencies into a single bundle or split bundles for efficient delivery.
- Hot Reloading: Supports fast refresh to speed up development by reloading only the modified code.
- Source Maps: Generates source maps to aid in debugging.
Metro is optimized for React Native, providing fast build times and support for custom module resolutions.
Evaluating Responses:
Candidates should demonstrate familiarity with Metro’s purpose and functionality. Bonus points for mentioning configuration options, troubleshooting, or comparing Metro with alternatives like Webpack or Vite.
13. How would you integrate third-party libraries into a React Native project?
Question Explanation:
This React Native Developer interview question evaluates the candidate’s familiarity with dependency management in React Native and their ability to handle third-party libraries, including native module integration.
Expected Answer:
Integrating third-party libraries into a React Native project typically involves these steps:
- Install the Library:
Use a package manager like npm or Yarn to install the library.
npm install react-native-some-library
- Link the Native Dependencies (React Native CLI):
For libraries with native code, link the dependencies:
npx react-native link react-native-some-library
If using autolinking (React Native 0.60+), this step is often unnecessary.
- Manual Linking (if needed):
- For Android, modify
MainApplication.java
andsettings.gradle
/build.gradle
files. - For iOS, add the library to the project via Xcode.
- Configure the Library:
Follow the library’s documentation to set up any necessary permissions or configurations. For example, adding keys toAndroidManifest.xml
orInfo.plist
. - Import and Use the Library:
import SomeLibrary from 'react-native-some-library';
SomeLibrary.doSomething();
- Test the Integration:
Verify the library works as intended on both platforms.
Evaluating Responses:
A good candidate will discuss both simple JavaScript libraries and those requiring native modules. Bonus points for mentioning potential issues, such as library compatibility, debugging, or resolving linking problems.
14. How do you ensure your React Native app is responsive across different screen sizes and devices?
Question Explanation:
This React Native Developer interview question tests the candidate’s ability to create mobile apps that look and perform well on a variety of devices with different screen sizes and resolutions.
Expected Answer:
To ensure responsiveness in a React Native app, you can use the following techniques:
- Percentage-Based Dimensions:
Use relative dimensions (width: '50%'
) instead of absolute values to make components scale dynamically. Dimensions
API:
Retrieve screen dimensions to adjust layouts.
import { Dimensions } from 'react-native';
const { width, height } = Dimensions.get('window');
- Flexbox:
Leverage Flexbox for responsive layouts, which adapt well to varying screen sizes.
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }} />
- Pixel Density (DP):
UsePixelRatio
to scale assets or text based on screen pixel density. - Third-Party Libraries:
Use libraries likereact-native-responsive-screen
orreact-native-size-matters
for scaling elements. - Platform-Specific Styles:
Use thePlatform
module to fine-tune styles for Android or iOS. - Testing on Multiple Devices:
Regularly test on different devices, simulators, and emulators to ensure consistent behavior
Evaluating Responses:
Candidates should demonstrate familiarity with the techniques above, particularly Flexbox and responsive libraries. Strong responses might include examples or mention the challenges of scaling fonts or handling dynamic layouts.
15. Can you explain the differences between Expo and React Native CLI? When would you use one over the other?
Question Explanation:
This React Native Developer interview question evaluates the candidate’s understanding of the two major ways to create and manage React Native projects.
Expected Answer:
Expo and React Native CLI are two tools for building React Native apps, but they differ significantly:
- Expo:
- Advantages:
- Provides an out-of-the-box development environment with minimal configuration.
- Offers a rich set of prebuilt APIs (e.g., camera, geolocation) without needing native code.
- Ideal for rapid prototyping or projects without custom native requirements.
- Disadvantages:
- Limited flexibility; you cannot modify native code directly.
- Dependency on the Expo ecosystem, which can restrict advanced customizations.
- Advantages:
- React Native CLI:
- Advantages:
- Full control over the native code, allowing custom module creation and integration.
- More suited for production apps requiring advanced native features.
- Disadvantages:
- Requires manual configuration and linking, which can increase complexity.
- Development setup might take longer compared to Expo.
- Advantages:
When to use:
- Expo: Use for quick prototypes, small apps, or when you don’t need native code customizations.
- React Native CLI: Use for production apps requiring extensive customization or third-party libraries with native code.
Evaluating Responses:
Candidates should articulate the differences clearly and provide scenarios where each is appropriate. Bonus points for sharing personal experience or examples of when they switched from Expo to React Native CLI (or vice versa).
React Native Interview Questions Conclusion
These questions provide a comprehensive overview of a candidate’s expertise in React Native development, covering fundamental concepts, advanced topics, and practical applications. Using this list, you can assess the candidate’s ability to deliver high-quality, efficient, and maintainable mobile applications. A strong React Native developer should demonstrate a deep understanding of the framework, problem-solving skills, and experience in building scalable apps tailored to user needs.