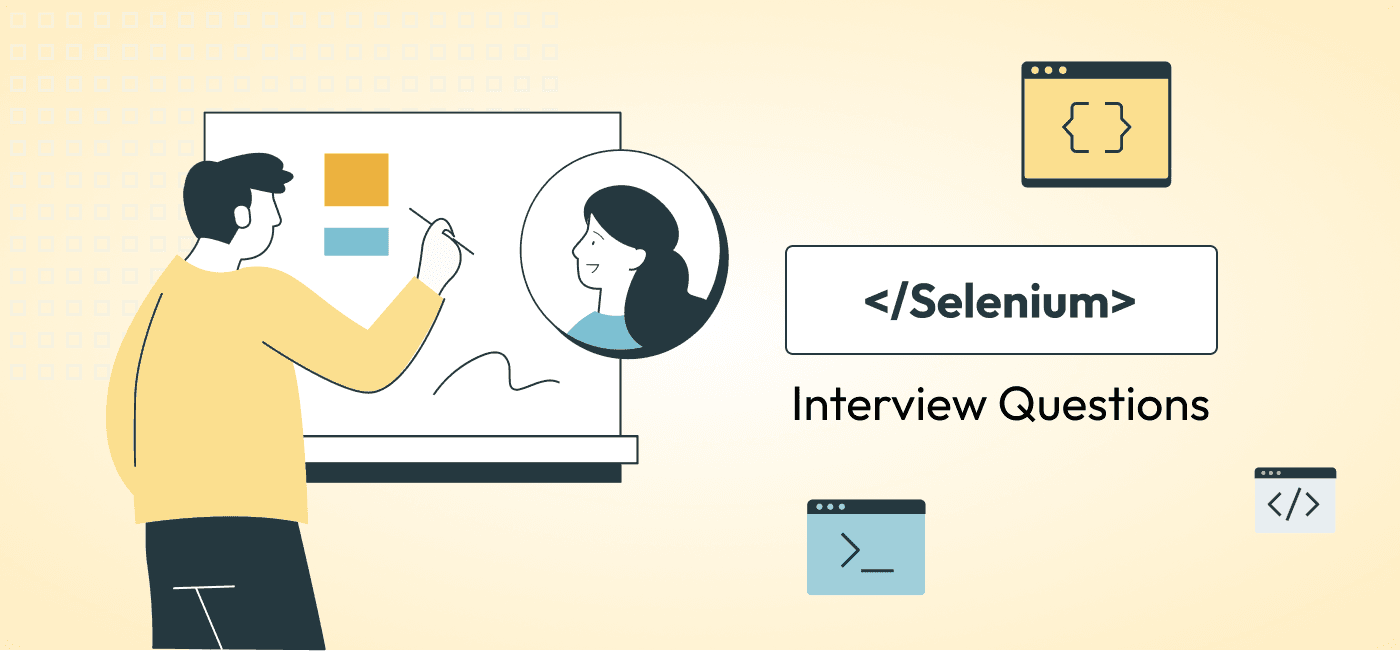
Hiring + recruiting | Blog Post
15 Selenium Interview Questions for Hiring Selenium Engineers
Todd Adams
Share this post
Hiring Selenium engineers requires a nuanced understanding of their technical prowess and practical experience. Selenium, a powerful framework for automating web applications for testing purposes, is a critical tool in the QA engineer’s toolkit. This document aims to assist hiring managers and technical interviewers in assessing candidates’ proficiency in Selenium. The Selenium interview questions listed below are designed to probe a candidate’s understanding of Selenium’s capabilities, its application in real-world scenarios, and their problem-solving skills in automated testing environments.
Selenium Interview Questions
Q1: What is Selenium, and can you explain the different components that make up the Selenium Suite?
Question Explanation:
Understanding Selenium and its components is fundamental for any Selenium engineer. Selenium is an open-source automation tool for web browsers, offering a range of solutions for different testing needs. The suite’s components are designed to provide a comprehensive toolset for testing web applications across various browsers and platforms.
Expected Answer:
- Selenium IDE (Integrated Development Environment): This is a simple, browser-based development tool for creating quick bug reproduction scripts and exploratory testing scripts. It allows tests to be recorded, edited, and debugged in a graphical interface. Selenium IDE supports rapid test development and is often used for creating test prototypes or learning Selenium syntax.
- Selenium WebDriver: WebDriver is a web automation framework that allows you to execute your tests against different browsers, like Chrome, Firefox, Internet Explorer, etc. It provides a programming interface to create and execute test cases. WebDriver directly communicates with the web browser and controls it at the OS level, offering robust and browser-specific testing.
- Selenium Grid: Grid allows you to run test scripts on multiple machines and browsers simultaneously. This component is used to speed up the execution of a test suite by leveraging parallel processing. It helps in testing under different environments and saves time by distributing tests across multiple machines.
- Selenium RC (Remote Control): Although deprecated and replaced by WebDriver, Selenium RC was the first integrated tool in the suite that allowed users to write automated web application UI tests in any programming language against any HTTP website using any mainstream JavaScript-enabled browser. RC served as a test domain-specific language (Selenese) interpreter.
Evaluating Responses:
Candidates should demonstrate a clear understanding of each Selenium suite component and its use cases. Look for responses that:
- Correctly identify and describe the purpose and functionalities of Selenium IDE, WebDriver, and Grid.
- Mention the deprecation of Selenium RC and its replacement by WebDriver, indicating up-to-date knowledge.
- Include examples or scenarios where each component could be particularly useful, showing practical understanding.
- Highlight the importance of using different components for specific testing needs, such as rapid prototyping, cross-browser testing, or parallel test execution.
Q2: Describe how you would set up a Selenium WebDriver environment for a web application testing project.
Question Explanation:
Setting up a Selenium WebDriver environment is a foundational step for any web application testing project. This process involves selecting and configuring the appropriate tools and frameworks to create a robust testing environment. A candidate’s ability to describe this setup process showcases their technical proficiency and practical experience in preparing for automated testing tasks.
Expected Answer:
- Choose a Programming Language: First, decide on a programming language to write your test scripts. Selenium supports multiple languages, including Java, C#, Python, Ruby, and JavaScript.
- Install a Programming Language Development Kit: Depending on the chosen language, install the necessary development kit, like JDK for Java.
- Download Selenium WebDriver: Obtain the WebDriver library for your programming language from the Selenium website or manage dependencies through a project management tool like Maven for Java or npm for JavaScript.
- Choose a Web Browser and Download WebDriver: Depending on the browser(s) you plan to test (e.g., Chrome, Firefox, Internet Explorer), download the corresponding WebDriver executable.
- Set Up an Integrated Development Environment (IDE): Install an IDE or text editor suitable for the chosen programming language, like IntelliJ IDEA for Java or Visual Studio Code for multiple languages.
- Configure Environment Variables: If necessary, configure environment variables for the WebDriver or development kit to facilitate the execution of scripts from any directory.
- Install Testing Frameworks (Optional): For structured testing and reporting, install a testing framework compatible with your programming language, like JUnit or TestNG for Java, or PyTest for Python.
- Write a Test Script: Create a simple test script to verify the setup. This script typically opens a web browser, navigates to a web page, performs a simple action, and closes the browser.
- Run the Test Script: Execute the test script to validate the environment setup, ensuring the chosen browser launches and performs the scripted actions.
Evaluating Responses:
- The candidate should detail each step in the setup process clearly and logically.
- Look for mentions of supporting tools and frameworks, indicating a comprehensive approach.
- Assess the candidate’s familiarity with different programming languages and their corresponding development environments.
- Successful responses will also cover the importance of choosing the right WebDriver for the browser(s) under test and the setup of environment variables for ease of script execution across different environments.
Q3: How do you handle different types of web elements in Selenium, such as buttons, text inputs, and drop-down menus?
Question Explanation:
Handling various web elements effectively is crucial for automating web application testing. Each element type, from buttons to drop-down menus, requires specific Selenium WebDriver methods for interaction. This Selenium interview question assesses the candidate’s knowledge and experience in manipulating these web elements to simulate user actions accurately.
Expected Answer:
- Buttons: To interact with buttons, you can use the
click()
method. First, locate the button element using locators likeid
,name
, orXPath
, and then apply theclick()
method to perform a click action.
driver.findElement(By.id("submit-button")).click();
- Text Inputs: For text input fields, use the
sendKeys()
method to simulate typing. Locate the text input and then clear it withclear()
before entering text withsendKeys()
.
WebElement inputField = driver.findElement(By.name("email"));
inputField.clear();
inputField.sendKeys("example@example.com");
- Drop-down Menus: Handling drop-down menus involves the
Select
class. After locating the drop-down element, create aSelect
object and use methods likeselectByVisibleText()
,selectByIndex()
, orselectByValue()
to choose an option.
Select dropdown = new Select(driver.findElement(By.id("dropdown")));
dropdown.selectByVisibleText("Option 1");
- Checkboxes and Radio Buttons: Use the
click()
method to select or deselect checkboxes. For radio buttons, locate the specific button you want to select and useclick()
.
driver.findElement(By.id("checkbox")).click();
driver.findElement(By.xpath("//input[@name='radioOption'][@value='value1']")).click();
Evaluating Responses:
- Look for detailed descriptions of methods used to interact with each element type, indicating practical experience.
- Successful answers will include code snippets showing how to locate and interact with elements, demonstrating familiarity with Selenium’s WebDriver API.
- Assess the candidate’s understanding of the
Select
class for drop-down menus, a common challenge in web automation. - The ability to articulate when to use specific methods like
clear()
beforesendKeys()
for text inputs reflects a nuanced understanding of web element behaviors.
Q4: Explain the concept of waits in Selenium. What is the difference between implicit wait and explicit wait?
Question Explanation:
Waits are a critical concept in Selenium for handling the asynchronous loading of web elements. Proper use of waits ensures that web elements are available for interaction, reducing the likelihood of test failures due to timing issues. This question probes the candidate’s understanding of wait mechanisms and their ability to implement them correctly.
Expected Answer:
- Implicit Wait: Tells the WebDriver to poll the DOM for a certain amount of time when trying to find an element or elements if they are not immediately available. The default setting is 0. Once set, the implicit wait is set for the life of the WebDriver object instance.
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
- Explicit Wait: Used to wait for a certain condition to occur before proceeding further in the code. It works with the
WebDriverWait
alongsideExpectedCondition
, providing more flexibility than implicit waits as it applies to specific elements with customizable conditions.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("visibleAfter")));
Evaluating Responses:
- Effective answers will clearly differentiate between implicit and explicit waits, highlighting the global versus conditional application.
- Look for examples demonstrating the implementation of both waits, showing practical application skills.
- The response should emphasize the flexibility and precision of explicit waits for handling elements that appear after complex interactions or delays.
- Understanding when to use each type of wait, considering their impact on the overall test execution time and reliability, is key.
Q5: Can you detail how you would automate a test case that requires file uploading using Selenium?
Question Explanation:
Automating file upload functionality is a common requirement in web application testing. This task can present challenges due to the need to interact with the file system, which is outside the browser’s domain. This Selenium interview question assesses the candidate’s ability to navigate these challenges and implement a robust solution.
Expected Answer:
- First, ensure that the file path is accessible to the test environment and correctly specified in the test script.
- Locate the file input element on the web page using Selenium’s WebDriver. This is typically done by identifying the element’s
id
,name
, or other attributes. - Use the
sendKeys()
method on the file input element to enter the file path. Unlike other uses ofsendKeys()
, this does not simulate typing but rather sets the file path directly on the input.
driver.findElement(By.id("file-upload")).sendKeys("/path/to/file.txt");
- After setting the file path, if the upload is initiated automatically upon file selection, the test should proceed to verify the upload’s success. If the upload requires clicking a “submit” button or similar action, use the
click()
method to perform this action.
Evaluating Responses:
- Successful answers will clearly outline the steps to automate file uploading, including locating the file input element and using
sendKeys()
to set the file path. - Look for mentions of handling file paths, especially in different environments, which can be a common source of errors.
- The ability to articulate subsequent steps, such as submitting the form or verifying the upload, demonstrates a comprehensive approach to test automation.
- The inclusion of example code snippets not only validates the candidate’s familiarity with Selenium’s API but also their practical experience in automating complex user interactions.
Q6: Discuss the Page Object Model (POM) in Selenium. Why is it important, and how do you implement it?
Question Explanation:
The Page Object Model (POM) is a design pattern in Selenium and other web automation frameworks that promotes better test maintenance and reduces code duplication. Understanding and implementing POM is crucial for creating scalable, maintainable, and reusable test scripts. This Selenium interview question evaluates the candidate’s knowledge of POM, its benefits, and their experience in applying it to test automation projects.
Expected Answer:
- Importance of POM: POM helps organize the code in a way that separates the representation of web pages from the test scripts. This separation allows for a single repository of page elements and interactions, making the test scripts cleaner and easier to maintain. It reduces duplication of code and simplifies the maintenance of tests, especially when web UI changes occur.
- Implementation Steps:
- Identify the Web Pages: Break down the application under test into logical units or pages.
- Create Page Classes: For each page identified, create a class that represents the page. This class includes locators for elements on the page and methods for interactions that can be performed on those elements.
public class LoginPage {
private WebDriver driver;
private By username = By.id("username");
private By password = By.id("password");
private By loginButton = By.id("loginButton");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String user) {
driver.findElement(username).sendKeys(user);
}
public void enterPassword(String pass) {
driver.findElement(password).sendKeys(pass);
}
public void clickLogin() {
driver.findElement(loginButton).click();
}
}
- Utilize Page Objects in Tests: In the test scripts, instantiate the page object classes and use their methods to perform actions on the web page.
Evaluating Responses:
- Look for a clear explanation of the POM’s advantages, such as code reusability, maintenance, and separation of concerns.
- The candidate should articulate the steps to implement POM, from identifying web pages to creating page classes and utilizing them in tests.
- Successful answers will include example code snippets showing the definition of page classes and their usage in test scripts, demonstrating practical application skills.
- The ability to describe the structural organization of tests using POM indicates a comprehensive understanding of test automation best practices.
Q7: How do you ensure cross-browser compatibility in your Selenium tests?
Question Explanation:
Ensuring that web applications function correctly across different browsers is a fundamental aspect of web development and testing. Cross-browser testing involves executing the same set of tests on multiple browsers to verify this compatibility. This question seeks to uncover the candidate’s strategies for implementing effective cross-browser testing using Selenium.
Expected Answer:
- Leverage WebDriver for Multiple Browsers: The first step is to configure Selenium WebDriver to work with the specific browsers you want to test. This involves downloading the driver executables for each browser (e.g., ChromeDriver for Google Chrome, GeckoDriver for Firefox) and setting system properties to point to these executables.
- Use a Testing Framework for Parameterized Tests: Utilize testing frameworks that support parameterized tests (e.g., TestNG or JUnit in Java) to easily run the same test cases with different configurations, including different browsers.
- Implement Selenium Grid: For larger scale testing, Selenium Grid can be used to run tests in parallel across different machines and browsers, significantly reducing the time required for cross-browser testing.
- Responsive Design Testing: Incorporate tests that adjust screen sizes and resolutions to ensure the application’s UI is responsive across different devices and browsers.
- Continuous Integration (CI) Pipeline Integration: Integrate cross-browser tests into a CI pipeline to automatically run them as part of the build process, ensuring that compatibility is checked regularly.
Evaluating Responses:
- Effective answers will detail the setup and use of WebDriver for multiple browsers, showcasing the candidate’s technical capabilities.
- Look for mentions of utilizing testing frameworks for parameterized tests, indicating an understanding of efficient test execution strategies.
- The implementation of Selenium Grid for parallel testing reflects knowledge of advanced testing methodologies.
- Consideration of responsive design testing demonstrates awareness of comprehensive testing strategies beyond functional correctness.
- Integration with CI pipelines indicates an advanced level of testing maturity and automation.
Q8: What strategies do you use to debug failing Selenium tests?
Question Explanation:
Debugging failing Selenium tests is an essential skill for identifying and resolving issues in automated test scripts. This process can involve analyzing error messages, reviewing test code, and understanding the application’s behavior at the time of failure. This Selenium interview question explores the candidate’s approach to troubleshooting and resolving issues in Selenium tests.
Expected Answer:
- Review Error Messages and Logs: Start by carefully examining the error messages and logs generated during the test execution. These can provide clues about what went wrong.
- Use Breakpoints and Step Through the Code: Employ an IDE to set breakpoints and step through the test code to observe the application’s state and behavior at various points.
- Inspect the Application’s State: Utilize browser developer tools to inspect the state of the web application, checking for changes in the DOM or JavaScript errors that may interfere with Selenium’s ability to interact with web elements.
- Verify Locators and Web Element Interactions: Double-check the locators used to identify web elements and ensure they are accurate and up-to-date. Also, review the sequences of interactions with web elements to ensure they mimic user behavior accurately.
- Increase Wait Times Temporarily: Temporarily increasing wait times can help determine if timing issues are causing the test failures, though this is not a long-term solution.
- Review Application Changes: Collaborate with developers to identify any recent changes in the application that could affect the test scripts, requiring updates to the tests.
Evaluating Responses:
- Successful responses will include a systematic approach to debugging, starting with error analysis and progressing through code review and application inspection.
- The use of development tools, such as IDEs and browser developer tools, indicates a hands-on, practical approach to troubleshooting.
- Highlighting the importance of collaboration with development teams reflects an understanding of the broader software development process.
- Awareness of the impact of application changes on test scripts underscores the importance of maintaining alignment between test automation and application development.
Q9: Explain how Selenium Grid works and how you have used it to improve testing efficiency.
Question Explanation:
Selenium Grid allows for the parallel execution of Selenium tests across multiple machines and browsers, optimizing the testing process for speed and coverage. This question assesses the candidate’s understanding of Selenium Grid and their experience leveraging it to enhance testing efficiency.
Expected Answer:
- How Selenium Grid Works: Selenium Grid consists of a central hub that receives test commands and multiple nodes that execute these tests in various browsers and environments. By distributing tests across these nodes, Grid enables parallel execution, reducing the time required to run extensive test suites.
- Setting Up Selenium Grid: To use Selenium Grid, set up a central hub and register multiple nodes with different browser capabilities to the hub. This setup can be done manually or through containerization tools like Docker for easier scalability.
- Improving Testing Efficiency:
- Parallel Execution: By running tests in parallel on multiple nodes, I significantly reduced the test execution time, making it possible to receive feedback faster and accelerate the development cycle.
- Cross-Browser Testing: Utilized Grid to run the same set of tests on different browsers simultaneously, ensuring application compatibility across a wider range of browsers without additional time.
- Resource Optimization: Leveraged Grid to use testing resources more efficiently, running tests on various configurations without the need for a large number of physical machines.
- Real-world Application: In a previous project, I implemented Selenium Grid to manage a suite of over 500 tests. By distributing these tests across 10 nodes with different browser and OS combinations, we cut down the execution time from several hours to under an hour, significantly speeding up the CI/CD pipeline.
Evaluating Responses:
- Look for a detailed explanation of Selenium Grid’s architecture, including the roles of the hub and nodes, indicating a solid understanding of the tool.
- Effective answers will describe specific benefits realized from using Selenium Grid, such as reduced execution time and improved cross-browser testing.
- Real-world examples of how the candidate has used Selenium Grid to enhance testing efficiency provide evidence of practical experience and the ability to apply theoretical knowledge to solve testing challenges.
- Discussion of setup strategies, especially the mention of modern tools like Docker for scalability and ease of management, reflects a candidate’s up-to-date technical skills and innovative approach to testing infrastructure.
Q10: Describe a scenario where you optimized Selenium test scripts for better performance. What techniques did you use?
Question Explanation:
Optimizing Selenium test scripts for better performance is crucial for efficient testing processes, especially in continuous integration and delivery pipelines. This Selenium interview question probes the candidate’s ability to identify performance bottlenecks in Selenium tests and their skill in applying effective optimization techniques.
Expected Answer:
- Scenario: In a past project, our Selenium test suite’s execution time was significantly long, causing delays in the feedback loop for developers. The primary issue was redundant navigation and excessive use of explicit waits.
- Optimization Techniques Used:
- Reduced Redundant Browser Navigation: I identified and removed unnecessary navigations to the same web page within multiple test cases, which cut down the overall test execution time.
- Optimized Use of Waits: Switched from a heavy reliance on explicit waits to more strategic use, only where necessary. Implemented fluent waits with custom conditions for more dynamic wait scenarios, which are more efficient than static thread sleeps or long explicit waits.
- Parallel Execution: Utilized Selenium Grid and TestNG’s parallel execution feature to run tests simultaneously across different browsers and environments, significantly reducing the time needed to complete the suite.
- Element Locators Optimization: Reviewed and optimized the locators used to find elements, preferring efficient locators like ID and class over XPath, which improved the speed of element identification.
- Leveraged Headless Browsers: For tests that did not require UI validation, I used headless browsers, which execute tests faster than traditional browsers by not rendering UI elements.
Evaluating Responses:
- Successful responses will outline a specific performance issue within a Selenium testing scenario and detail the optimization strategies applied.
- Look for mentions of techniques like reducing redundant actions, optimizing waits, parallel testing, efficient locator strategies, and the use of headless browsers, indicating a comprehensive approach to performance optimization.
- The inclusion of before-and-after metrics, such as execution time, can help illustrate the effectiveness of the optimizations.
- An understanding of the balance between test speed and reliability is crucial, as overly aggressive optimizations can lead to flaky tests.
Q11: How do you use Selenium to test dynamic content on web pages, such as AJAX or JavaScript-generated content?
Question Explanation:
Testing dynamic content, which changes without the page reloading (e.g., AJAX or JavaScript modifications), presents unique challenges. These elements may not be present immediately or might change state after the page loads. This question assesses the candidate’s strategies for effectively testing dynamic content with Selenium.
Expected Answer:
- Strategies for Testing Dynamic Content:
- Explicit Waits: Use explicit waits to wait for a specific condition to be met before interacting with elements. This is essential for elements that appear or change due to AJAX calls.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
- Fluent Waits: Implement fluent waits for more complex conditions, where you might need to check for an element’s presence or state at regular intervals until a timeout is reached. This approach provides flexibility in handling elements that might take variable times to load or become interactive.
- JavaScript Executor: Use Selenium’s JavaScript Executor to interact with elements that are dynamically generated by JavaScript. This can be particularly useful when standard Selenium methods are not effective.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("arguments[0].click();", element);
- Repeated Attempts: In scenarios where elements are extremely volatile, try to interact with the element multiple times within a loop, coupled with short waits, to accommodate for the dynamic nature of the content.
Evaluating Responses:
- Effective answers should highlight an understanding of Selenium’s explicit and fluent waits, demonstrating knowledge of when and how to use them for dynamic content.
- The use of JavaScript Executor as a workaround for challenging dynamic elements indicates a deep understanding of Selenium’s capabilities and web technologies.
- Mention of repeated attempts strategy shows practical experience with extremely dynamic or unpredictable web elements.
- Look for a detailed description of the problem-solving process, indicating a methodical approach to handling dynamic content.
Q12: What is XPath, and how do you use it in Selenium to locate elements?
Question Explanation:
XPath (XML Path Language) is a query language for selecting nodes from an XML document, which, in the context of Selenium, is used to navigate through elements and attributes in the HTML document of a web page. This question examines the candidate’s knowledge of XPath and its application in creating robust and precise locators for web elements in Selenium tests.
Expected Answer:
- XPath Fundamentals: XPath allows for navigating the HTML structure of a web page using path expressions, enabling testers to locate elements based on their hierarchical position, attributes, or text content. It’s particularly useful for locating elements that do not have unique IDs or class names.
- Using XPath in Selenium:
- To locate elements using XPath, the
findElement(By.xpath("xpath_expression"))
method is used. - Absolute XPath: Starts with the root node and follows the path down to the desired element. It’s not recommended for most cases as it’s brittle and prone to breaking if the HTML structure changes.
- To locate elements using XPath, the
WebElement element = driver.findElement(By.xpath("/html/body/div[1]/section/div"));
- Relative XPath: Uses double slashes (
//
) to skip intermediate elements, making it more flexible and resilient to changes in the document structure. You can also use predicates and operators to refine your selection.
WebElement loginButton = driver.findElement(By.xpath("//button[@id='login']"));
- Advanced XPath Expressions: XPath supports functions and operators for advanced queries, such as selecting elements based on partial attribute values, indexing, or conditions like the presence of child elements.
Evaluating Responses:
- Look for a clear distinction between absolute and relative XPath, highlighting the advantages of using relative XPath for its flexibility and resilience to changes in the HTML structure.
- Successful responses will include examples of basic and advanced XPath expressions, demonstrating the candidate’s ability to craft precise locators.
- The mention of XPath functions and operators indicates an advanced level of understanding, suitable for complex web element identification scenarios.
- Responses should emphasize the strategic use of XPath, considering its power and potential pitfalls, such as performance implications when used excessively or with overly complex expressions.
Q13: How do you manage data-driven testing in Selenium?
Question Explanation:
Data-driven testing involves running a set of tests multiple times with different input values. It is an effective way to increase the coverage of test cases by systematically varying the test data. This Selenium interview question seeks to understand the candidate’s approach to implementing data-driven testing in Selenium, ensuring comprehensive test coverage and efficient testing processes.
Expected Answer:
- Approach to Data-driven Testing:
- Identify Test Cases for Data-driven Testing: Not all tests are suitable for data-driven approaches. Identify which tests could benefit from multiple data sets, such as form submissions or login functionality.
- Prepare Test Data: Collect the test data in a manageable format, such as Excel spreadsheets, CSV files, or databases. Ensure the data is clean, relevant, and covers a wide range of scenarios, including edge cases.
- Use a Testing Framework: Leverage testing frameworks that support data-driven testing, such as TestNG for Java or PyTest for Python. These frameworks offer annotations or decorators to easily feed multiple sets of data into the same test case.
- Implementing with Selenium:
- With TestNG, you can use the
@DataProvider
annotation to specify a method that returns an array of objects containing the test data. Then, link this data provider to your test method with thedataProvider
attribute.
- With TestNG, you can use the
@DataProvider(name = "loginData")
public Object[][] getData() {
return new Object[][] {{"user1", "password1"}, {"user2", "password2"}};
}
@Test(dataProvider = "loginData")
public void testLogin(String username, String password) {
// Code to perform login
}
- Handling Test Data: Ensure proper management and confidentiality of test data, especially when dealing with sensitive information. Use data masking or anonymization techniques if necessary.
Evaluating Responses:
- Effective answers should outline a structured approach to data-driven testing, from the selection of test cases to the preparation of test data.
- Look for familiarity with testing frameworks and specific features or annotations used to implement data-driven testing, as this demonstrates practical knowledge and experience.
- The inclusion of code snippets showing how to use data providers or similar mechanisms indicates the candidate’s technical capability to implement data-driven tests with Selenium.
- An awareness of test data management and security considerations reflects a mature approach to testing practices and concerns beyond just the technical execution.
Q14: Discuss how Selenium integrates with other tools and frameworks, such as Jenkins for CI/CD or TestNG for test management.
Question Explanation:
Integration of Selenium with other tools and frameworks amplifies its capabilities, enabling automated testing within broader development and deployment workflows. This question aims to explore the candidate’s experience and knowledge about leveraging Selenium in conjunction with other software development tools, focusing on continuous integration/continuous deployment (CI/CD) systems like Jenkins and test frameworks like TestNG.
Expected Answer:
- Integration with Jenkins for CI/CD:
- Automating Test Execution: Selenium tests can be integrated into Jenkins pipelines to automate the execution of tests as part of the build and deployment process. This ensures that new changes are tested before being deployed to production.
- Configuring Jenkins: Use Jenkins plugins, such as the HTML Publisher plugin, to display Selenium test reports. Configure Jenkins to trigger Selenium test suites upon code commits, scheduled times, or manually.
- Feedback Loop: The integration provides immediate feedback on the impact of recent changes, facilitating quick bug identification and resolution.
pipeline {
agent any
stages {
stage('Test') {
steps {
script {
// Commands to run Selenium tests
}
}
}
}
post {
always {
// Publish Selenium test reports
}
}
}
- Using TestNG for Test Management:
- Structured Testing: TestNG offers annotations and XML configuration files to structure Selenium tests more effectively, allowing for grouping, sequencing, and parameterization of tests.
- Data-driven Testing: As mentioned earlier, TestNG’s
@DataProvider
feature facilitates data-driven testing, essential for thorough testing strategies. - Reporting and Logging: Integration with TestNG also enhances Selenium’s reporting capabilities, providing detailed test execution reports and logs for better debugging and analysis.
Evaluating Responses:
- Successful responses will detail the process and benefits of integrating Selenium with Jenkins and TestNG, demonstrating an understanding of the value these integrations bring to automated testing and CI/CD pipelines.
- Look for specifics on configuring Jenkins to automate Selenium test execution and the use of TestNG features to enhance test structuring and execution.
- The inclusion of example code or configuration snippets, especially for Jenkins pipelines or TestNG XML files, indicates practical experience with these integrations.
- Awareness of the feedback mechanisms and reporting capabilities these integrations offer reflects an understanding of the importance of quick feedback and thorough analysis in agile development environments.
Q15: Share a challenging issue you encountered while using Selenium and how you resolved it.
Question Explanation:
This question seeks to uncover a candidate’s problem-solving skills and their ability to navigate technical challenges in test automation with Selenium. It provides insight into the candidate’s experience with real-world issues, their diagnostic process, and their capability to implement effective solutions.
Expected Answer:
- Issue: One of the most challenging issues I encountered was dealing with a complex, dynamically generated web table that was not consistently identifiable with standard Selenium locators. The table’s structure would change based on the data it displayed, making it difficult to select specific cells or rows reliably.
- Diagnostic Process: Initially, attempts to use XPath and CSS selectors failed to consistently locate elements within the table. I utilized browser developer tools to observe the patterns in the table’s HTML structure and JavaScript console logs to understand how and when the table was being generated.
- Resolution:
- Custom XPath Expressions: Developed a set of custom XPath expressions that accounted for the variability in the table’s structure. This involved using XPath functions like
contains()
,starts-with()
, and sibling/ancestor axes to navigate the DOM more flexibly. - JavaScript Executor: For parts of the table where XPath was still unreliable, I used Selenium’s JavaScript Executor to directly manipulate the DOM and retrieve the necessary data or perform actions on the elements.
- Wait Adjustments: Implemented a combination of explicit and fluent waits to account for the dynamic loading of table data, ensuring that actions were performed only when the data was fully loaded and the DOM was stable.
- Custom XPath Expressions: Developed a set of custom XPath expressions that accounted for the variability in the table’s structure. This involved using XPath functions like
- Outcome: These solutions collectively addressed the issue, enabling reliable interaction with the dynamic web table. This approach not only solved the immediate problem but also improved my ability to deal with similar issues in the future, enhancing overall test reliability and efficiency.
Evaluating Responses:
- Effective answers will clearly describe a specific technical challenge, including enough detail to understand the issue’s complexity.
- Look for a thorough diagnostic process that demonstrates methodical problem-solving skills and a deep understanding of web technologies and Selenium’s capabilities.
- The resolution should involve creative and technically sound solutions, indicating the candidate’s ability to adapt and innovate in the face of challenging automation tasks.
- Reflection on the outcome and the lessons learned shows an ability to apply experience to future challenges, a key trait for advancing automation expertise.
Conclusion
The questions outlined above are crafted to unearth a candidate’s depth of knowledge and practical experience with Selenium. Each question targets critical areas of Selenium automation, from setup and configuration to advanced testing scenarios and optimization strategies. In assessing the answers, interviewers should look for detailed understanding, practical application, and problem-solving skills. These questions are pivotal in identifying candidates who are not only proficient in Selenium but also capable of leveraging its full potential to enhance testing efficacy and efficiency.