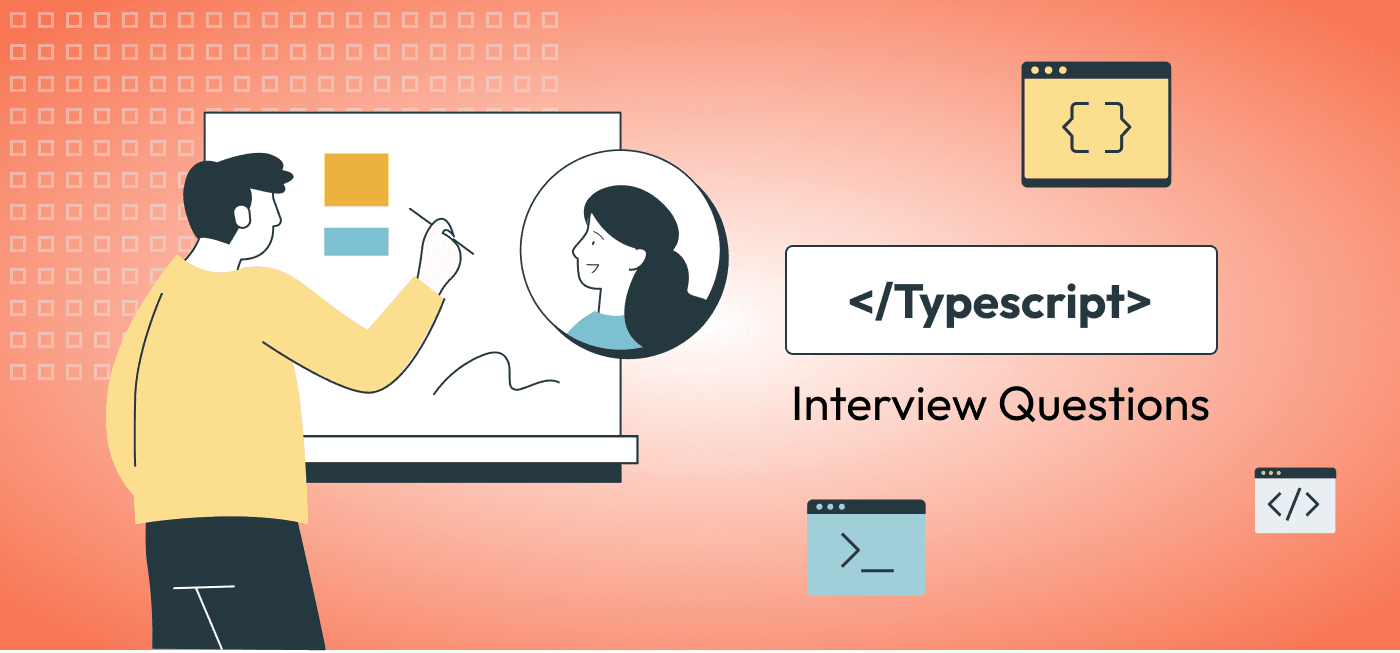
Hiring + recruiting | Blog Post
15 TypeScript Interview Questions for Hiring TypeScript Engineers
Todd Adams
Share this post
When hiring for technical roles that require expertise in TypeScript, it’s essential to evaluate candidates’ knowledge and practical skills in this language. TypeScript, a strongly typed superset of JavaScript, enhances code quality and maintainability, making it a crucial tool for modern web development. The following Typescript interview questions are designed to probe a candidate’s understanding of TypeScript and their ability to apply this knowledge in real-world scenarios.
Typescript Interview Questions
1. What are the main differences between TypeScript and JavaScript?
Question Explanation: Understanding the fundamental differences between TypeScript and JavaScript is crucial because TypeScript builds on JavaScript by adding static types, which can significantly impact how code is written and maintained.
Expected Answer:
TypeScript is a superset of JavaScript that adds static typing and other features to the language. Here are the main differences:
- Static Typing: TypeScript introduces a type system that allows developers to define types for variables, function parameters, and return values. This helps catch errors at compile time rather than at runtime.
- Compile-Time Checking: TypeScript code is compiled to JavaScript. The TypeScript compiler performs type checking and generates JavaScript code that can run in any JavaScript environment.
- Advanced Features: TypeScript includes features not found in standard JavaScript, such as interfaces, enums, and generics, which help in writing more robust and maintainable code.
- Tooling and Editor Support: TypeScript provides better tooling and editor support thanks to type information, which enhances code navigation, autocompletion, and refactoring capabilities.
Evaluating Responses:
A strong response will clearly articulate the differences, emphasizing the benefits of static typing and compile-time checking. The candidate should demonstrate an understanding of how these features improve code quality and development efficiency.
2. How does TypeScript handle static type checking?
Question Explanation: Static type checking is a core feature of TypeScript that differentiates it from JavaScript. Understanding how it works is essential for leveraging TypeScript’s full potential.
Expected Answer:
TypeScript performs static type checking by analyzing the code at compile time to ensure that values conform to the types defined for variables, function parameters, and return values. This process involves:
- Type Annotations: Developers can specify types using type annotations, such as
let age: number = 25;
. - Type Inference: TypeScript can infer types when they are not explicitly provided. For example,
let age = 25;
will infer thatage
is of typenumber
. - Compiler Errors: When the TypeScript compiler detects type mismatches or potential type-related issues, it generates compile-time errors, preventing the code from running until the issues are resolved.
- Strict Mode: TypeScript can be configured in strict mode to enforce stricter type checking rules, catching more potential errors.
Evaluating Responses:
Look for a response that mentions both type annotations and type inference. The candidate should also explain the role of the TypeScript compiler and the benefits of catching errors at compile time. Mentioning strict mode is a plus.
3. Can you explain the concept of “interfaces” in TypeScript and how they differ from “types”?
Question Explanation: Interfaces and types are fundamental features in TypeScript for defining the shapes of objects and ensuring type safety. This Typescript interview question helps probe on how understanding their differences and uses is key to effectively using TypeScript.
Expected Answer:
In TypeScript, interfaces and types both define the shapes of objects, but they have some differences:
- Interfaces:
- Used to define the structure of an object, specifying property names and their types.
- Can be extended using the
extends
keyword to create new interfaces that build on existing ones. - Example:
interface Person {
name: string;
age: number;
}
interface Employee extends Person {
employeeId: number;
}
- Types:
- Can define the structure of objects similar to interfaces but can also be used for other types, such as union types, intersection types, and more.
- Cannot be extended but can use intersection to combine types.
- Example:
type Person = {
name: string;
age: number;
}
type Employee = Person & {
employeeId: number;
4. What is the purpose of “Generics” in TypeScript, and can you provide an example?
Question Explanation: Generics are a powerful feature that allows for the creation of reusable and flexible components. They enable developers to write functions, classes, and interfaces that work with different types without sacrificing type safety.
Expected Answer:
Generics in TypeScript allow developers to create components that can work with a variety of types while maintaining type safety. They enable the definition of functions, classes, and interfaces that are not restricted to a single type.
- Purpose: The main purpose of generics is to provide a way to create reusable components that can operate with different data types, ensuring type safety and reducing code duplication.
- Example:
function identity<T>(arg: T): T {
return arg;
}
// Usage:
let num = identity<number>(42); // T is number
let str = identity<string>('Hello'); // T is string
Evaluating Responses:
The candidate should clearly explain that generics allow for type-safe code that is reusable and adaptable. A good answer will include an example demonstrating the use of generics in a function or class, showing how the generic type parameter can be applied in different contexts.
5. How do you declare and use enums in TypeScript?
Question Explanation: Enums provide a way to define a set of named constants, which can make code more readable and maintainable. Understanding how to declare and use enums is important for structuring and organizing related values in TypeScript.
Expected Answer:
In TypeScript, enums (short for enumerations) allow developers to define a collection of related values that can be used as constants. Enums can be numeric or string-based.
- Declaration:
enum Direction {
Up = 1,
Down,
Left,
Right
}
enum Color {
Red = "RED",
Green = "GREEN",
Blue = "BLUE"
}
- Usage:
let move: Direction = Direction.Up;
console.log(move); // Output: 1
let favoriteColor: Color = Color.Green;
console.log(favoriteColor); // Output: "GREEN"
Evaluating Responses:
A good response will include both numeric and string enum examples, showing how to declare and use them. The candidate should explain the benefits of using enums for grouping related constants and enhancing code readability.
6. What are the benefits of using TypeScript’s “Union” and “Intersection” types?
Question Explanation: Union and intersection types enhance TypeScript’s type system by allowing more flexible and expressive type definitions. This Typescript interview questions questions how understanding their benefits helps in creating robust and adaptable type-safe code.
Expected Answer:
Union and intersection types in TypeScript offer powerful ways to define and work with types.
- Union Types: Allow a value to be one of several types.
- Benefits: Union types provide flexibility in accepting multiple types, making functions and variables more versatile.
type ID = number | string;
let userId: ID;
userId = 123; // valid
userId = "abc"; // valid
- Intersection Types: Combine multiple types into one, requiring a value to satisfy all specified types.
interface Person {
name: string;
}
interface Employee {
employeeId: number;
}
type Staff = Person & Employee;
let staffMember: Staff = {
name: "Alice",
employeeId: 101
};
- Benefits: Intersection types ensure that an object satisfies multiple type requirements, enhancing type safety and ensuring more comprehensive type definitions.
Evaluating Responses:
The candidate should explain the syntax and usage of union and intersection types, highlighting their benefits in terms of flexibility and type safety. Examples demonstrating how to declare and use these types are essential.
7. How does TypeScript support “Decorators”? Can you give an example of their usage?
Question Explanation: Decorators are a feature that allows for the modification of classes and their members. Understanding decorators is important for leveraging advanced TypeScript capabilities, particularly in frameworks like Angular.
Expected Answer:
Decorators in TypeScript provide a way to add annotations and metadata to classes and their members. They are a form of syntactic sugar that simplifies adding functionality to classes, methods, accessors, properties, or parameters.
- Enabling Decorators: To use decorators, the
experimentalDecorators
option must be enabled in thetsconfig.json
file.
{
"compilerOptions": {
"experimentalDecorators": true
}
}
- Usage Example:
function Log(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function(...args: any[]) {
console.log(`Calling ${propertyKey} with arguments: ${args}`);
return originalMethod.apply(this, args);
};
return descriptor;
}
class Calculator {
@Log
add(a: number, b: number): number {
return a + b;
}
}
const calculator = new Calculator();
calculator.add(2, 3); // Output: Calling add with arguments: 2,3
Evaluating Responses:
A good answer will include an explanation of how to enable decorators, a simple example of a decorator, and how it is applied to a class or method. The candidate should demonstrate an understanding of the decorator’s purpose and functionality.
8. Explain the concept of “Type Inference” in TypeScript.
Question Explanation: this Typescript interview questions evaluates type inference, a key feature of TypeScript that helps reduce the need for explicit type annotations. Type inferences makes code easier to write and maintain while still benefiting from type safety.
Expected Answer:
Type inference in TypeScript refers to the compiler’s ability to automatically deduce the types of variables and expressions without explicit type annotations.
- Basic Example:
let age = 25; // TypeScript infers the type as number
let name = "Alice"; // TypeScript infers the type as string
- Function Example:
function add(a: number, b: number) {
return a + b; // TypeScript infers the return type as number
}
- Complex Example:
let user = {
name: "Alice",
age: 25
};
// TypeScript infers the type as { name: string; age: number; }
Benefits:
- Less Boilerplate: Reduces the need for explicit type annotations, making the code more concise.
- Type Safety: Maintains type safety by ensuring that inferred types are checked at compile time.
- Improved Readability: Enhances code readability by reducing unnecessary type declarations.
Evaluating Responses:
Look for explanations of how TypeScript infers types from variable assignments and function returns. Examples should illustrate basic and complex cases of type inference. The candidate should articulate the benefits of type inference in terms of reducing boilerplate and maintaining type safety.
9. How does TypeScript handle “Modules” and “Namespaces”?
Question Explanation: Modules and namespaces are important features in TypeScript for organizing and managing code. Understanding their differences and how to use them is crucial for maintaining a well-structured codebase.
Expected Answer:
Modules:
- Definition: Modules are files in TypeScript that contain code and export functions, objects, or primitives. They use the
import
andexport
keywords to include or expose functionalities. Example:
// math.ts
export function add(a: number, b: number): number {
return a + b;
}
// app.ts
import { add } from './math';
console.log(add(2, 3)); // Output: 5
Namespaces:
- Definition: Namespaces are used to group related code under a single identifier, preventing global scope pollution and potential naming conflicts. Example:
namespace Geometry {
export function areaOfCircle(radius: number): number {
return Math.PI * radius * radius;
}
}
console.log(Geometry.areaOfCircle(5)); // Output: 78.53981633974483
Differences:
- Modules:
- Are file-based.
- Recommended for use in modern TypeScript and JavaScript applications.
- Supports asynchronous module loading.
- Namespaces:
- Are mainly used for organizing code within a single file or a set of related files.
- Are considered less favorable compared to modules for large applications.
Evaluating Responses: A comprehensive answer should include definitions, syntax, and examples of both modules and namespaces. The candidate should highlight the differences and mention that modules are generally preferred in modern applications.
10. Can you discuss the use of “Type Assertions” in TypeScript and provide an example?
Question Explanation: Type assertions allow developers to override the type inferred by TypeScript, which can be useful when the developer knows more about the type than the compiler. Understanding this concept is important for handling complex type scenarios.
Expected Answer:
Definition: Type assertions are used to tell the TypeScript compiler to treat an entity as a different type than the one it inferred. This can be helpful in situations where the developer has more context about the type than the compiler.
Syntax:
- Using the
as
keyword:
let value: any = "this is a string";
let strLength: number = (value as string).length;
- Using angle brackets (not recommended in JSX):
Example:
interface User {
name: string;
age: number;
}
let user = {} as User;
user.name = "Alice";
user.age = 25;
console.log(user); // Output: { name: 'Alice', age: 25 }
Evaluating Responses: The candidate should clearly explain both syntaxes and provide practical examples. The explanation should cover why and when to use type assertions, emphasizing that they should be used cautiously as they override the compiler’s type checking.
11. What is the difference between “interface” and “class” in TypeScript?
Question Explanation: Interfaces and classes are fundamental constructs in TypeScript, serving different purposes in defining object shapes and creating object instances. Understanding their differences is essential for effective TypeScript programming.
Expected Answer:
Interface:
- Definition: An interface defines the shape of an object by specifying property names and their types. It is purely a type declaration and does not generate any JavaScript code. Example:
interface Person {
name: string;
age: number;
}
let person: Person = {
name: "Alice",
age: 25
};
Class:
- Definition: A class defines a blueprint for creating objects with properties and methods. It can include implementation details and can be instantiated to create object instances. Example:
class Person {
name: string;
age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name}`);
}
}
let person = new Person("Alice", 25);
person.greet(); // Output: Hello, my name is Alice
Differences:
- Usage: Interfaces are used to define the structure of an object, while classes are used to create objects and encapsulate implementation details.
- Inheritance: Interfaces can be extended using the
extends
keyword, while classes can extend other classes and implement interfaces using theimplements
keyword. - Output: Interfaces are removed during compilation and do not generate JavaScript code, whereas classes are transpiled to JavaScript.
Evaluating Responses: A strong answer will include definitions, examples, and differences between interfaces and classes. The candidate should emphasize the use cases for each and how they contribute to TypeScript’s type system and object-oriented programming.
12. How do you use “Mapped Types” in TypeScript?
Question Explanation: this Typescript interview question evaluates mapped types, which allow for the transformation of existing types into new types according to specified rules. Understanding how to use them is important for advanced type manipulation and creating flexible, reusable types.
Expected Answer:
Definition: Mapped types in TypeScript enable the creation of new types by transforming properties of existing types. This is done using type operators and generic types.
Example:
- Basic Mapped Type:
type Readonly<T> = {
readonly [P in keyof T]: T[P];
};
interface User {
name: string;
age: number;
}
type ReadonlyUser = Readonly<User>;
let user: ReadonlyUser = {
name: "Alice",
age: 25
};
// user.name = "Bob"; // Error: Cannot assign to 'name' because it is a read-only property.
- Partial Mapped Type:
type Partial<T> = {
[P in keyof T]?: T[P];
};
type PartialUser = Partial<User>;
let user: PartialUser = {
name: "Alice"
};
Benefits:
- Reusability: Mapped types allow for the creation of reusable and flexible types that can adapt to changes in the underlying types.
- Consistency: They help maintain consistency by ensuring that type transformations follow a specific pattern.
Evaluating Responses: The candidate should explain what mapped types are and provide examples of common mapped types like Readonly
and Partial
. A strong response will also discuss the benefits of using mapped types for type transformation and reusability.
13. Can you explain how “Optional Chaining” works in TypeScript?
Question Explanation: Optional chaining is a feature that allows for safe access to nested properties, methods, or array elements even if an intermediate property is null
or undefined
. Understanding this helps prevent runtime errors and simplifies code.
Expected Answer:
Definition: Optional chaining is an operator (?.
) that lets you safely access deeply nested properties without having to check for the existence of each property in the chain. If any part of the chain is null
or undefined
, the entire expression returns undefined
.
Example:
interface User {
name: string;
address?: {
street?: string;
city?: string;
};
}
let user: User = { name: "Alice" };
// Without optional chaining:
let street = user.address ? user.address.street : undefined;
// With optional chaining:
let streetWithOptionalChaining = user.address?.street;
console.log(streetWithOptionalChaining); // Output: undefined
Benefits:
- Safety: Prevents runtime errors by returning
undefined
if any part of the property chain isnull
orundefined
. - Readability: Simplifies the code by reducing the need for repetitive null checks.
- Maintenance: Makes the code easier to maintain by clearly indicating optional properties.
Evaluating Responses: A good response will clearly explain the syntax and purpose of the optional chaining operator. The candidate should provide an example demonstrating how it simplifies code and prevents errors. Emphasize the benefits of using optional chaining for code safety and readability.
14. What are “Conditional Types” in TypeScript, and how do they work?
Question Explanation: Conditional types allow for type selection based on a condition, enabling more dynamic and flexible type definitions. Understanding conditional types is essential for advanced type manipulation.
Expected Answer:
Definition: Conditional types in TypeScript are a way to select one of two possible types based on a condition. They follow the syntax T extends U ? X : Y
, where T
is the type being checked, U
is the type to compare against, and X
and Y
are the resulting types.
Example:
type IsString<T> = T extends string ? "Yes" : "No";
type A = IsString<string>; // "Yes"
type B = IsString<number>; // "No"
Advanced Example:
type Flatten<T> = T extends any[] ? T[number] : T;
type A = Flatten<string[]>; // string
type B = Flatten<number>; // number
Benefits:
- Flexibility: Conditional types enable more flexible and dynamic type definitions, adapting based on the given type.
- Type Safety: They enhance type safety by allowing precise type manipulations and ensuring that types conform to specific conditions.
Evaluating Responses: A strong response should include basic and advanced examples of conditional types. The candidate should explain how they work and their benefits, emphasizing the increased flexibility and type safety they provide.
15. How does TypeScript enhance the development workflow and code maintainability compared to JavaScript?
Question Explanation: This Typescript interview question assesses the candidate’s understanding of TypeScript’s overall benefits in a development environment, focusing on productivity and long-term code quality.
Expected Answer:
Enhanced Development Workflow:
- Type Safety: TypeScript’s static type checking catches errors at compile time, reducing runtime errors and making the code more reliable.
- Intelligent Code Completion: TypeScript provides better tooling support with intelligent code completion, navigation, and refactoring capabilities in editors like VSCode, improving developer productivity.
- Documentation: Type annotations serve as a form of documentation, making the code easier to understand and maintain.
Improved Code Maintainability:
- Refactoring: TypeScript makes refactoring safer and easier by ensuring that changes in types propagate correctly throughout the codebase.
- Modularity: Encourages the use of modules and interfaces, leading to a more modular and organized code structure.
- Scalability: TypeScript’s features like interfaces, generics, and type aliases help in building scalable and maintainable large codebases.
Evaluating Responses: Look for answers that highlight specific features of TypeScript and how they contribute to a better development experience and code maintainability. The candidate should discuss both immediate productivity gains and long-term benefits in maintaining a codebase. Practical examples of how TypeScript improves these aspects are a plus.
Conclusion
These TypeScript interview questions are designed to evaluate a candidate’s depth of knowledge and practical experience with the language. By asking these questions, interviewers can gain insights into the candidate’s understanding of TypeScript’s advanced features, type system, and their ability to apply this knowledge to write robust and maintainable code. These questions help ensure that the candidate is well-equipped to leverage TypeScript effectively in a professional development environment.