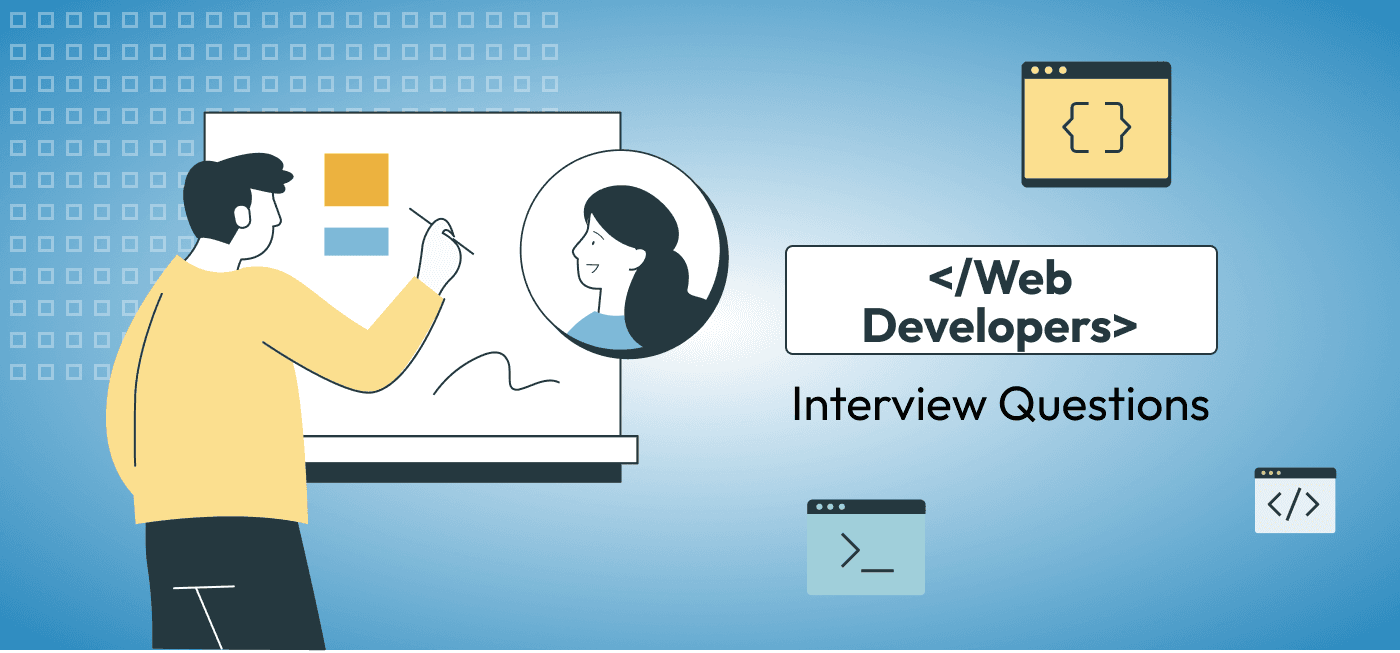
Hiring + recruiting | Blog Post
15 Web Development Interview Questions for Hiring Web Developers
Todd Adams
Share this post
The hiring process for web developers requires assessing a range of skills, from front-end technologies to back-end development and a solid grasp of web performance and security best practices. This document provides a comprehensive list of interview questions designed to evaluate a candidate’s technical expertise, problem-solving ability, and understanding of web development principles. These questions will help you identify qualified professionals who can contribute to building high-quality web applications.
Web Development Interview Questions
1. What are the core differences between HTTP and HTTPS?
Question Explanation:
This web development interview question evaluates a candidate’s understanding of basic web protocols, particularly in the context of web security. HTTP and HTTPS are foundational concepts that affect data transmission, security, and user trust.
Expected Answer:
HTTP (Hypertext Transfer Protocol) is a protocol for transferring data over the web. It is not encrypted, which means data is transmitted in plain text, making it vulnerable to interception by attackers. HTTPS (Hypertext Transfer Protocol Secure) adds a layer of security by using SSL/TLS encryption to secure the communication between the client and the server.
Key differences include:
- Encryption: HTTP transmits data in plain text, while HTTPS encrypts the data.
- Authentication: HTTPS uses SSL/TLS certificates to authenticate the server and sometimes the client, ensuring that users are communicating with the intended website.
- Performance: HTTPS may involve slightly higher latency due to encryption overhead, but HTTP/2 and optimizations mitigate this.
- SEO and Trust: HTTPS improves search engine rankings and provides a padlock icon in the browser, increasing user trust.
Evaluating Responses:
Look for candidates who demonstrate a clear understanding of security implications, can explain SSL/TLS in simple terms, and understand HTTPS’s benefits for user trust and SEO.
2. How does the browser render a webpage from the moment a URL is entered?
Question Explanation:
This web development interview question tests a candidate’s knowledge of the browser’s rendering pipeline, a critical process in delivering a smooth and efficient user experience.
Expected Answer:
When a URL is entered, the browser follows these steps:
- DNS Resolution: Resolves the domain name to an IP address.
- HTTP Request and Response: Sends an HTTP(S) request to the server and receives a response, typically containing HTML, CSS, and JavaScript files.
- Parsing HTML: The browser parses the HTML to create the Document Object Model (DOM).
- Parsing CSS: CSS is parsed to create the CSSOM (CSS Object Model).
- Rendering Tree: The DOM and CSSOM are combined to create the Render Tree.
- Layout: Determines the size and position of elements on the page.
- Painting: Renders visual elements on the screen.
- JavaScript Execution: Executes JavaScript, potentially modifying the DOM and triggering reflows or repaints.
Evaluating Responses:
A strong candidate should articulate this process step-by-step, possibly including performance optimization tips like reducing render-blocking resources and lazy-loading assets.
3. Can you explain the differences between inline, block, and inline-block elements in CSS?
Question Explanation:
This web development interview question examines a candidate’s grasp of CSS fundamentals and their ability to structure web pages using appropriate display properties.
Expected Answer:
- Inline:
- Inline elements do not start on a new line.
- Their width and height are determined by their content.
- Examples:
<span>
,<a>
,<strong>
. - Inline elements cannot have margin/padding affect surrounding elements vertically.
- Block:
- Block elements start on a new line and take up the full width of their parent container.
- Examples:
<div>
,<p>
,<h1>
through<h6>
. - Can have margin/padding affect surrounding elements both horizontally and vertically.
- Inline-block:
- Combines properties of both inline and block elements.
- Does not start on a new line, but width and height can be set.
- Examples:
<img>
,<button>
(default display behavior in many browsers).
Evaluating Responses:
Look for clear examples and distinctions between the display types. Candidates should also demonstrate understanding of practical use cases for each (e.g., inline-block for layouts without floats or flex).
4. How would you optimize a web application’s performance?
Question Explanation:
This web development interview question assesses a candidate’s ability to identify and apply performance optimization techniques that enhance user experience and improve page load speed.
Expected Answer:
- Minimize HTTP Requests: Use techniques like bundling CSS/JS, using image sprites, and lazy-loading images.
- Optimize Assets: Compress images, use modern formats like WebP, and minify CSS/JavaScript.
- Use Content Delivery Networks (CDNs): Distribute assets to reduce latency.
- Enable Caching: Use browser caching and server-side caching to store static assets.
- Implement Asynchronous Loading: Use asynchronous or defer attributes for scripts to prevent blocking the rendering process.
- Reduce Server Response Times: Optimize server performance and use techniques like gzip compression.
- Use Performance Monitoring Tools: Leverage tools like Lighthouse or WebPageTest to identify bottlenecks and monitor Core Web Vitals (e.g., LCP, FID, CLS).
Evaluating Responses:
An ideal response demonstrates a deep understanding of the problem areas that impact performance and provides actionable solutions. The candidate should ideally reference modern tools and techniques for testing and optimizing performance.
5. What is the Document Object Model (DOM), and how is it manipulated in modern web development?
Question Explanation:
This web development interview question evaluates a candidate’s understanding of the DOM, which is central to web development. Proficiency in manipulating the DOM demonstrates the ability to create dynamic, interactive user interfaces.
Expected Answer:
The DOM (Document Object Model) is a programming interface for web documents. It represents the structure of a web page as a tree-like hierarchy of nodes, allowing developers to access, modify, and update content and styles dynamically.
Key operations include:
- Selecting Elements: Using methods like
document.getElementById()
,querySelector()
, andgetElementsByClassName()
. - Modifying Content: Using properties like
innerHTML
,textContent
, or methods likeappendChild()
andremoveChild()
. - Styling Elements: Modifying styles dynamically through the
style
property or class manipulation (classList.add()
/remove()
). - Event Handling: Adding event listeners using
addEventListener()
to respond to user actions like clicks or keypresses. - Modern Tools: Leveraging libraries like React or Vue.js, which abstract direct DOM manipulation for improved efficiency and developer experience.
Evaluating Responses:
Look for clear explanations and examples. A strong candidate should also mention the inefficiency of frequent direct DOM manipulations and suggest modern frameworks or the virtual DOM as a solution.
6. Describe the differences between var
, let
, and const
in JavaScript.
Question Explanation:
This web development interview question checks for the candidate’s knowledge of variable declaration in JavaScript and their understanding of scope and immutability concepts introduced with ES6.
Expected Answer:
var
:- Has function scope or global scope, not block scope.
- Allows variable re-declaration within the same scope.
- Can lead to bugs due to hoisting, where the variable is accessible before its declaration.
let
:- Introduced in ES6, it has block scope.
- Prevents re-declaration within the same scope, improving code predictability.
- Is also hoisted but remains in the “temporal dead zone” until initialized.
const
:- Like
let
, it has block scope but is used for constants. - Cannot be reassigned after initialization, ensuring immutability for primitive values.
- Objects or arrays declared with
const
can still have their contents modified due to reference immutability, not deep immutability.
- Like
Evaluating Responses:
A complete answer should include examples of scope and hoisting behavior. Candidates should demonstrate an understanding of when and why to use each declaration type.
7. Explain the purpose of RESTful APIs in web development.
Question Explanation:
This web development interview question assesses a candidate’s understanding of REST principles and their role in enabling communication between client and server in a web application.
Expected Answer:
REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful APIs allow communication between clients (e.g., a browser or mobile app) and servers using standard HTTP methods.
Key principles:
- Statelessness: Each API request must contain all the information needed to complete the request, as the server does not retain client state.
- Resources: Data is represented as resources, each identified by a unique URL.
- Standard HTTP Methods: Common methods include
GET
(retrieve),POST
(create),PUT
/PATCH
(update), andDELETE
(remove). - JSON/XML: Responses are typically formatted in JSON or XML for easy parsing by clients.
Advantages:
- Platform independence and simplicity.
- Scalability due to stateless nature.
- Easy integration with a wide variety of clients.
Evaluating Responses:
Candidates should articulate the importance of RESTful APIs in separating front-end and back-end concerns and demonstrate knowledge of common HTTP methods and status codes. Bonus points if they can compare REST to alternatives like GraphQL or SOAP.
8. What are CSS preprocessors like SASS or LESS, and why would you use them?
Question Explanation:
This web development interview question tests the candidate’s understanding of CSS preprocessors and their ability to write maintainable and scalable CSS for complex projects.
Expected Answer:
CSS preprocessors like SASS (Syntactically Awesome Stylesheets) and LESS (Leaner Style Sheets) extend CSS by introducing advanced features that simplify and enhance the development process.
Features:
- Variables: Define reusable values for colors, font sizes, and more.
$primary-color: #3498db;
body { color: $primary-color; }
- Nesting: Allows hierarchical structuring of CSS rules
nav {
ul { margin: 0; }
li { display: inline; }
}
- Mixins: Reusable blocks of CSS, making code DRY (Don’t Repeat Yourself).
@mixin flex-center {
display: flex;
justify-content: center;
align-items: center;
}
div { @include flex-center; }
- Partials and Importing: Enables modular CSS by dividing styles into separate files.
Advantages:
- Improves readability and maintainability of styles.
- Enables better organization in large projects.
- Facilitates rapid styling with reusable components.
Evaluating Responses:
Look for candidates who can explain the added value of preprocessors and provide concrete examples of how they’ve used SASS or LESS in projects. Bonus points for mentioning how these tools integrate with build tools like Webpack or Gulp.
9. Can you discuss the importance of cross-browser compatibility and how you handle it?
Question Explanation:
This web development interview question evaluates the candidate’s awareness of web application behavior across different browsers and their ability to write code that provides a consistent user experience.
Expected Answer:
Cross-browser compatibility ensures a website functions and looks consistent across different browsers, platforms, and devices. It’s crucial for reaching a wider audience and avoiding user frustration.
Key Challenges:
- Differences in how browsers interpret HTML, CSS, and JavaScript.
- Variability in support for modern web features.
- Issues with older browsers like Internet Explorer.
Strategies to Handle Cross-Browser Compatibility:
- Use Modern CSS/JS Features with Fallbacks: Implement progressive enhancement and provide polyfills (e.g., Babel for JavaScript).
- Feature Detection: Use tools like Modernizr to detect browser capabilities and adjust behavior accordingly.
- Testing: Regularly test applications in multiple browsers using tools like BrowserStack or Sauce Labs.
- Standards Compliance: Write clean, standards-compliant code to minimize inconsistencies.
- CSS Resets/Normalizers: Use libraries like Normalize.css to standardize default styles across browsers.
Evaluating Responses:
A strong candidate will highlight specific challenges they’ve faced and the tools or methodologies they used to address them. Look for proactive testing practices and awareness of tools like polyfills and transpilers.
10. How do you ensure the security of web applications, particularly against common threats like XSS and SQL injection?
Question Explanation:
This web development interview question examines the candidate’s understanding of web application security fundamentals, which is crucial for protecting sensitive data and user information.
Expected Answer:
Cross-Site Scripting (XSS):
- XSS occurs when an attacker injects malicious scripts into a website.
- Prevention Strategies:
- Escape user input before rendering it in HTML.
- Use Content Security Policy (CSP) headers to restrict allowed sources of scripts.
- Sanitize inputs with libraries like DOMPurify.
SQL Injection:
- SQL Injection involves injecting malicious SQL queries through user input.
- Prevention Strategies:
- Use parameterized queries or prepared statements (e.g., with libraries like Sequelize or Knex in Node.js).
- Validate and sanitize all inputs before using them in database queries.
Other Practices:
- Secure cookies and implement HTTP-only and Secure flags.
- Use HTTPS for secure data transmission.
- Regularly audit and update dependencies to address known vulnerabilities.
Evaluating Responses:
Look for depth in understanding the threats and solutions. Candidates should mention specific techniques and demonstrate practical application, such as writing sanitized queries or using CSPs.
11. What is the difference between client-side and server-side rendering?
Question Explanation:
This web development interview question evaluates a candidate’s understanding of rendering approaches and their impact on performance, SEO, and user experience.
Expected Answer:
- Client-Side Rendering (CSR):
- Rendering occurs in the browser using JavaScript frameworks like React or Angular.
- Pros: Faster initial setup for developers, rich client-side interactivity, reduced server load.
- Cons: Slower initial page load (due to JavaScript loading), poor SEO unless handled with tools like Next.js.
- Server-Side Rendering (SSR):
- Rendering occurs on the server, delivering a fully rendered HTML page to the browser.
- Pros: Faster initial load times, better SEO due to pre-rendered content.
- Cons: Increased server load, slower client-side interactivity.
Hybrid Models:
- Static Site Generation (SSG): Pre-renders pages at build time for fast load times (e.g., Gatsby).
- ISR (Incremental Static Regeneration): Combines benefits of SSR and SSG (e.g., with Next.js).
Evaluating Responses:
A strong candidate should clearly explain the pros and cons and recommend a strategy based on use cases (e.g., CSR for SPAs, SSR for SEO-critical pages). Bonus points for mentioning modern frameworks like Next.js or Nuxt.js.
12. Can you describe your process for debugging and resolving front-end issues?
Question Explanation:
This web development interview question assesses a candidate’s problem-solving skills and their familiarity with debugging tools and techniques used in front-end development.
Expected Answer:
- Reproduce the Issue:
- Gather details from bug reports, including browser/version, steps to reproduce, and expected/actual outcomes.
- Inspect Code and Tools:
- Use browser developer tools (e.g., Chrome DevTools) to inspect DOM, CSS, and network requests.
- Use console logging (
console.log
,console.warn
,console.error
) or debugging breakpoints to trace the issue.
- Testing Hypotheses:
- Check for syntax or logic errors in JavaScript.
- Validate CSS rules to ensure no conflicts or unintended styles.
- Test in multiple browsers to identify compatibility issues.
- Iterate and Solve:
- Modify the code incrementally, testing fixes after each change.
- Use tools like Linters (e.g., ESLint) to catch code-quality issues.
- Prevent Future Issues:
- Write automated tests (unit, integration, and end-to-end).
- Document the root cause and resolution for team reference.
Evaluating Responses:
Candidates should demonstrate a structured approach and familiarity with debugging tools. Look for clarity in problem identification and steps taken to resolve issues effectively. Bonus points for mentioning collaborative techniques, like pair programming or using tools like Git to track code changes.
13. What are WebSockets, and how do they differ from HTTP?
Question Explanation:
This web development interview question tests a candidate’s knowledge of real-time communication technologies and their ability to explain how WebSockets improve upon traditional HTTP for specific use cases.
Expected Answer:
WebSockets are a communication protocol that provides full-duplex communication channels over a single TCP connection. They allow real-time interaction between a client and server without the need for repeated HTTP requests.
Differences Between WebSockets and HTTP:
- Connection: HTTP is stateless and connectionless (new request-response cycle for each interaction), while WebSockets establish a persistent connection.
- Real-Time Communication: WebSockets enable bidirectional communication, making them ideal for real-time applications like chat apps, live notifications, and gaming.
- Efficiency: WebSockets reduce overhead by avoiding repeated HTTP headers and use a single connection for continuous data exchange.
- Use Case: HTTP is better suited for standard request-response scenarios, whereas WebSockets excel in scenarios requiring low-latency updates.
Evaluating Responses:
Candidates should clearly articulate when and why to use WebSockets, highlighting its advantages for specific use cases. Bonus points for mentioning libraries or frameworks like Socket.IO for implementation.
14. Explain the concept of Progressive Web Apps (PWAs) and their advantages.
Question Explanation:
This web development interview question evaluates the candidate’s understanding of modern web development techniques that improve user engagement and offline functionality, emphasizing the importance of PWAs.
Expected Answer:
A Progressive Web App (PWA) is a web application that uses modern web technologies to deliver an app-like experience in the browser.
Key Features of PWAs:
- Offline Functionality: Uses Service Workers to cache assets and data for offline use.
- Installability: Can be installed on devices and appear like native apps with an app icon.
- Responsive Design: Adapts seamlessly to different screen sizes and devices.
- Push Notifications: Engages users with timely updates.
Advantages:
- Improved Performance: Faster load times and seamless offline capabilities.
- Cost-Effective: Eliminates the need to develop separate native apps for multiple platforms.
- User Engagement: Increases retention with features like push notifications and app installability.
- Cross-Platform: Works on all platforms with a standards-compliant browser.
Evaluating Responses:
Look for candidates who understand the technical implementation (e.g., Service Workers, Web App Manifests) and can explain how PWAs improve user experience. Bonus points for examples of projects where they’ve implemented PWAs.
15. How would you implement responsive design to ensure a website works well on all devices?
Question Explanation:
This web development interview question assesses the candidate’s ability to create adaptable and user-friendly designs that provide consistent experiences across devices and screen sizes.
Expected Answer:
Responsive design ensures that a website adjusts its layout, content, and functionality based on the user’s device.
Key Techniques:
- CSS Media Queries:
- Use media queries to apply different styles based on screen size.
@media (max-width: 768px) {
body { font-size: 14px; }
}
- Fluid Layouts and Flexible Grid Systems:
- Use percentage-based widths and tools like CSS Grid or Flexbox for layouts.
- Responsive Images:
- Use the
srcset
attribute to serve different image sizes based on device resolution.
<img src="small.jpg" srcset="medium.jpg 768w, large.jpg 1200w" sizes="(max-width: 768px) 100vw, 50vw" alt="Example">
- Mobile-First Design:
- Start with styles optimized for smaller devices and add enhancements for larger screens.
- Testing Across Devices:
- Test using tools like Chrome DevTools, BrowserStack, or physical devices.
Evaluating Responses:
A strong candidate should explain these techniques and provide examples of their application. Look for awareness of accessibility (e.g., ensuring readable text and tappable buttons) as part of responsive design.
Web Development Interview Questions Conclusion
Hiring web developers requires identifying professionals with a blend of technical expertise, creative problem-solving, and a strong understanding of modern web standards. These questions are designed to test a candidate’s ability to build scalable, secure, and user-friendly web applications. Use this list as a framework to select the best candidates for your team, ensuring they possess the skills needed to thrive in your development environment.