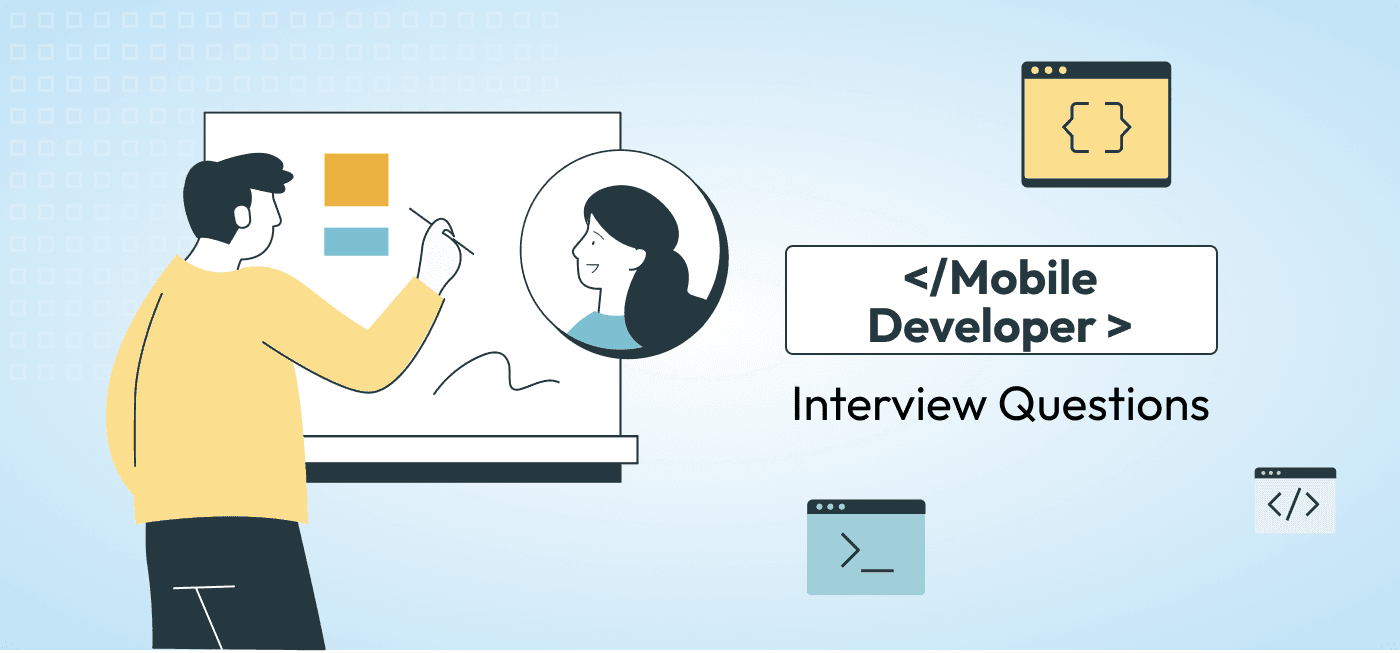
Hiring + recruiting | Blog Post
15 Mobile Developer Interview Questions for Hiring Mobile Engineers
Todd Adams
Share this post
In today’s fast-paced digital world, mobile applications have become essential for businesses to reach and engage their audience. Hiring a skilled mobile developer is critical for building high-quality, user-friendly, and performant apps. Below are 15 insightful mobile developer questions to help you assess a candidate’s technical expertise, problem-solving skills, and experience in mobile development.
Mobile Developer Interview Questions
1. Can you explain the differences between native, hybrid, and cross-platform app development?
Question Explanation:
This Mobile Developer interview question evaluates the candidate’s understanding of different mobile development approaches and their trade-offs. It reveals their knowledge of platform-specific development and tools.
Expected Answer:
Native development involves building mobile apps specifically for one platform, like Android (using Kotlin/Java) or iOS (using Swift/Objective-C). These apps offer optimal performance and full access to platform features but require separate codebases for each platform.
Hybrid apps are built using web technologies like HTML, CSS, and JavaScript and are wrapped in a native shell using tools like Apache Cordova. They are easier to develop but may suffer from performance limitations and reduced native feature support.
Cross-platform development uses frameworks like Flutter or React Native to create apps from a single codebase that work on multiple platforms. This approach balances development speed and platform support but might not always achieve the same level of performance as native apps.
Evaluating Responses:
Look for candidates who demonstrate an understanding of the trade-offs, such as native apps being better for performance-critical tasks, while cross-platform is cost-effective. Strong candidates may also discuss specific use cases or their experience with these approaches.
2. How do you optimize the performance of a mobile app?
Question Explanation:
Performance optimization is crucial for user experience. This Mobile Developer interview question assesses the candidate’s ability to identify and mitigate performance bottlenecks.
Expected Answer:
To optimize app performance, the candidate might mention strategies like reducing app startup time by lazy-loading resources, optimizing images for the device screen, and using efficient algorithms for processing data.
They could discuss techniques like caching data to minimize network requests, using hardware-accelerated animations, and offloading heavy computations to background threads. Profiling tools like Android Profiler or Instruments in Xcode should also be mentioned for identifying performance issues.
Evaluating Responses:
A strong response will be detailed and include specific examples, such as how they used tools to analyze and improve performance in past projects. Candidates who can articulate trade-offs, like balancing battery life and performance, demonstrate deeper expertise.
3. What is your approach to handling different screen sizes and device orientations?
Question Explanation:
Mobile apps must adapt to a variety of screen sizes and orientations. This Mobile Developer interview question checks the candidate’s understanding of responsive design and platform-specific tools.
Expected Answer:
For Android, candidates should discuss using ConstraintLayout, dimension qualifiers (e.g., sw600dp
), and resource folders for handling different screen densities and sizes. On iOS, they might mention Auto Layout, size classes, and the use of storyboard constraints.
Responsive design principles, such as flexible layouts, scalable UI components, and testing across devices, should be highlighted. They could also reference adaptive images and dynamic font scaling.
Evaluating Responses:
Good candidates will describe both general principles and platform-specific tools. Bonus points if they mention challenges they’ve faced, such as handling edge cases like foldable devices or notches, and how they resolved them.
4. Explain how you manage state in your mobile applications.
Question Explanation:
State management is crucial for maintaining consistency in app behavior. This Mobile Developer interview question evaluates the candidate’s experience with managing app state and their knowledge of related tools.
Expected Answer:
Candidates might mention that state management involves tracking and updating the UI based on changes in the underlying data. For React Native, they could discuss using Redux, Context API, or MobX. Flutter developers may refer to Provider, Riverpod, or Bloc.
They should highlight strategies like keeping state localized whenever possible and separating UI state from business logic. For native apps, Android developers could discuss ViewModel and LiveData, while iOS developers may reference Combine or SwiftUI’s @State.
Evaluating Responses:
Look for clear, structured answers that explain the benefits and challenges of specific approaches. Candidates should demonstrate an understanding of avoiding unnecessary re-renders and ensuring thread safety when managing state. Examples of state-related bugs they’ve fixed add credibility.
5. Describe a challenging bug or issue you faced in a mobile app project. How did you resolve it?
Question Explanation:
This Mobile Developer interview question assesses the candidate’s problem-solving skills, debugging process, and ability to communicate technical challenges effectively.
Expected Answer:
A strong response would include a specific example of a bug or issue. For instance, the candidate might describe a memory leak causing app crashes. They could explain how they identified the problem using tools like Android Profiler or Instruments in Xcode, pinpointed the source (e.g., unclosed database cursors or retained references), and resolved it by properly releasing resources.
Another example could involve debugging a layout issue caused by inconsistent rendering across devices. They might explain testing on different screen sizes, identifying the root cause (e.g., incorrect constraints or missing density qualifiers), and applying a scalable design solution.
Evaluating Responses:
Look for candidates who describe their process logically, including identifying the issue, debugging, implementing a solution, and validating it. Bonus points if they explain how they prevented similar issues in the future (e.g., adding automated tests or improving documentation).
6. What is the importance of memory management in mobile app development, and how do you handle it?
Question Explanation:
Efficient memory management is critical for maintaining app stability and performance, especially on resource-constrained mobile devices. This Mobile Developer interview question evaluates the candidate’s technical understanding and practical experience.
Expected Answer:
Memory management involves ensuring an app uses resources efficiently without leaks or excessive consumption. For Android, candidates might describe avoiding memory leaks by using weak references, releasing resources in the appropriate lifecycle methods, and using tools like LeakCanary to detect leaks.
On iOS, they might mention Automatic Reference Counting (ARC), managing retain cycles by using weak or unowned references, and avoiding memory over-allocation through careful handling of images, caching, and data structures.
They could also discuss coding practices like limiting the use of large objects in memory, recycling unused objects, and ensuring proper lifecycle handling for listeners or callbacks.
Evaluating Responses:
Good responses will reference tools and techniques relevant to their platform (e.g., LeakCanary, Instruments) and show an awareness of the practical trade-offs in memory management. Candidates who share examples of memory-related issues they’ve resolved demonstrate hands-on expertise.
7. How do you integrate third-party APIs or libraries into a mobile app?
Question Explanation:
Integrating third-party APIs or libraries is a common task in mobile development. This Mobile Developer interview question evaluates the candidate’s familiarity with tools, best practices, and problem-solving skills related to third-party integrations.
Expected Answer:
Candidates might start by describing their preferred package managers (e.g., Gradle for Android, CocoaPods/Swift Package Manager for iOS) for integrating libraries. They should emphasize checking library compatibility, maintaining version control, and reading documentation to configure the API correctly.
For API integration, they might explain setting up HTTP requests using libraries like Retrofit or OkHttp (Android) or Alamofire (iOS) and handling authentication (e.g., API keys, OAuth). They could also highlight practices like robust error handling, logging, and testing responses with mock APIs.
Evaluating Responses:
Good candidates will describe the steps they take to ensure a seamless integration, such as testing for edge cases, monitoring performance impacts, or resolving dependency conflicts. Look for real-world examples where they had to troubleshoot issues, such as version mismatches or poorly documented APIs.
8. Can you explain the lifecycle of a mobile application on Android and/or iOS?
Question Explanation:
Understanding app lifecycles is fundamental for developing stable and responsive apps. This Mobile Developer interview question checks the candidate’s ability to manage app states effectively.
Expected Answer:
For Android, candidates should describe lifecycle methods like onCreate()
, onStart()
, onResume()
, onPause()
, onStop()
, and onDestroy()
in activities and fragments. They might explain handling state restoration with onSaveInstanceState()
and how lifecycle-aware components like ViewModel and LiveData can simplify state management.
For iOS, they might discuss application(_:didFinishLaunchingWithOptions:)
, applicationDidBecomeActive
, applicationWillResignActive
, applicationDidEnterBackground
, and applicationWillTerminate
. They could also mention using Combine or SwiftUI’s lifecycle management tools.
Evaluating Responses:
A thorough answer will include how the candidate uses these lifecycle methods to optimize resource management, handle user interactions, or improve performance. Strong candidates will provide examples, like saving user progress when the app enters the background or managing background tasks effectively.
9. How do you ensure the security of sensitive user data in a mobile app?
Question Explanation:
Security is critical in mobile app development, particularly when handling sensitive user data like personal information, payment details, or credentials. This Mobile Developer interview question evaluates the candidate’s knowledge of secure coding practices and data protection strategies.
Expected Answer:
Candidates should mention encrypting sensitive data, both at rest (e.g., using SQLCipher for databases or Keychain for iOS) and in transit (e.g., enforcing HTTPS with TLS). They might also discuss securing API calls with token-based authentication like OAuth 2.0 and implementing proper session management.
Other strategies include validating all inputs to prevent injection attacks, obfuscating app code to deter reverse engineering, and using platform-specific security features like Android Keystore or iOS Secure Enclave for storing sensitive keys.
Additionally, they should mention performing regular security audits and using tools like OWASP Mobile Security Testing Guide to identify vulnerabilities.
Evaluating Responses:
Strong candidates will provide concrete examples of how they’ve implemented security measures in past projects. They should also demonstrate an awareness of emerging threats and a proactive approach to security, such as monitoring for libraries with known vulnerabilities.
10. What testing strategies and tools do you use to ensure the quality of your apps?
Question Explanation:
Testing is vital for delivering a stable and bug-free mobile application. This Mobile Developer interview question assesses the candidate’s experience with testing techniques and tools.
Expected Answer:
Candidates should describe a comprehensive testing approach, including unit tests (e.g., using JUnit for Android or XCTest for iOS) to validate individual components, integration tests to ensure modules work together, and UI tests (e.g., Espresso, XCTest UI) to validate user flows.
They might also discuss automating tests using tools like Appium, Firebase Test Lab, or BrowserStack and employing continuous integration/continuous deployment (CI/CD) pipelines for consistent testing. Manual testing for usability and exploratory testing for edge cases should also be mentioned.
Candidates could highlight the importance of monitoring and logging tools, like Crashlytics or Sentry, for tracking issues in production.
Evaluating Responses:
Look for candidates who can explain their testing strategy with examples from past projects. Strong candidates will emphasize balancing automated and manual testing and show familiarity with CI/CD and debugging tools.
11. Have you worked with app store submission processes (Google Play Store and Apple App Store)? If so, describe your experience.
Question Explanation:
Publishing apps requires following specific guidelines and procedures for each app store. This Mobile Developer interview question evaluates the candidate’s familiarity with these processes and their attention to detail.
Expected Answer:
For the Google Play Store, candidates might describe creating a signed APK or AAB, setting up a developer account, and completing the listing with metadata, screenshots, and descriptions. They should highlight awareness of Google’s policy requirements, including permission usage and content guidelines.
For the Apple App Store, they might explain creating an Apple Developer account, generating provisioning profiles, and submitting the app via Xcode or Transporter. They should also mention handling App Store reviews and addressing common rejection issues, like non-compliance with Apple’s Human Interface Guidelines.
They could discuss optimizing for ASO (App Store Optimization) to improve app discoverability.
Evaluating Responses:
Candidates with firsthand experience should mention handling challenges like debugging issues during uploads or rejections. Bonus points if they’ve managed updates, beta testing (e.g., TestFlight or Play Console), or feature releases.
12. What tools and frameworks do you prefer for debugging mobile applications?
Question Explanation:
Debugging is an essential skill for identifying and resolving issues in mobile apps. This Mobile Developer interview question evaluates the candidate’s proficiency with debugging tools and techniques.
Expected Answer:
On Android, candidates might mention using Android Studio’s built-in debugger, Logcat for viewing logs, and tools like Stetho for network debugging. They could also discuss using Firebase Crashlytics to monitor and diagnose crashes in production.
On iOS, they might describe using Xcode’s debugger, Instruments for profiling memory and CPU usage, and network tools like Charles Proxy. Debugging React Native or Flutter apps might involve Chrome Developer Tools or Flutter DevTools.
Strong candidates might also discuss debugging best practices, like isolating issues with breakpoints, using verbose logging, and testing edge cases.
Evaluating Responses:
Good responses will mention specific tools and explain how they’ve used them to solve complex issues. Look for examples of creative debugging techniques, such as using custom logs or simulating edge cases like network interruptions.
13. How do you implement offline functionality in a mobile app?
Question Explanation:
Offline functionality is critical for apps that need to function without consistent internet access. This Mobile Developer interview question evaluates the candidate’s understanding of caching, data synchronization, and storage solutions.
Expected Answer:
Candidates might discuss caching strategies, such as storing data locally using SQLite, Room (Android), Core Data (iOS), or local storage libraries like Hive for Flutter. They could also mention using frameworks like WorkManager (Android) or BackgroundTasks (iOS) to queue background sync operations.
For data synchronization, they may describe techniques like conflict resolution algorithms for merging changes when the app reconnects to the internet. Strategies for handling large datasets efficiently, such as pagination and data compression, should also be mentioned.
They might reference patterns like the Repository Pattern for managing data sources and discuss fallback mechanisms to gracefully degrade app features when offline.
Evaluating Responses:
Look for candidates who show awareness of edge cases, such as syncing issues or handling offline mode transitions. Bonus points for examples where they successfully implemented offline functionality and addressed challenges like storage limitations or data consistency.
14. Describe your experience with mobile app animations and transitions. What tools or techniques do you use?
Question Explanation:
Animations and transitions enhance user experience by making interactions smooth and intuitive. This Mobile Developer interview question assesses the candidate’s ability to design and implement effective animations.
Expected Answer:
Candidates should mention tools like Android’s MotionLayout, Property Animations, or Jetpack Compose animations, and iOS’s UIView animations or SwiftUI’s built-in animation APIs. They might describe creating custom animations for transitions between screens or interactive UI elements.
They could also discuss optimizing animations for performance, ensuring they don’t overuse the main thread, and leveraging hardware acceleration. Candidates might reference libraries like Lottie for vector-based animations or their experience creating micro-interactions.
Evaluating Responses:
Good responses include examples of animations they’ve implemented, such as a carousel, progress bar, or custom transitions. Look for understanding of animation best practices, like maintaining accessibility and avoiding excessive animations that could impact performance.
15. How do you keep yourself updated with the latest trends and technologies in mobile development?
Question Explanation:
This Mobile Developer interview question evaluates the candidate’s passion for their craft and their ability to adapt to the rapidly evolving mobile development landscape.
Expected Answer:
Candidates might describe following blogs, documentation, or platforms like Medium, Dev.to, or official blogs by Google (Android Developers Blog) and Apple (WWDC resources). They might mention attending conferences, watching talks from WWDC or Google I/O, and participating in developer communities like Stack Overflow or GitHub.
They could also highlight their hands-on approach to learning by experimenting with new tools, contributing to open-source projects, or taking online courses on platforms like Udemy or Coursera.
Evaluating Responses:
Strong candidates demonstrate not only an awareness of current trends (e.g., Jetpack Compose, SwiftUI, Flutter, or Kotlin Multiplatform) but also an eagerness to continuously improve their skills. Examples of recent technologies they’ve explored or integrated into projects show initiative.